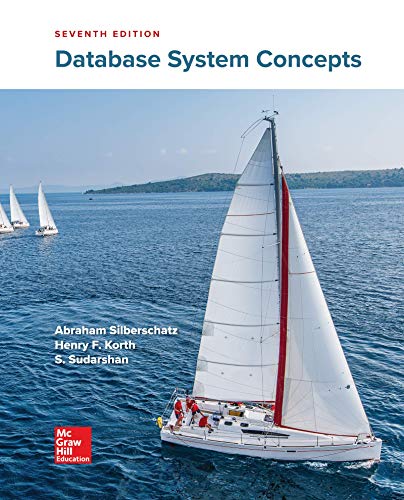
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
In C++ language
For number 2 through 4 create an integer array with 100 randomly generated values between 0 and 99; pass this array into all subsequent functions. Place code in your main to call all the methods and demonstrate they work correctly.
-
Using just the at, length, and substr string methods and the + (concatenate) operator, write a function that accepts a string s, a start position p, and a length l, and returns a subset of s with the characters starting at position p for a length of l removed. Don’t forget that strings start at position 0. Thus (“abcdefghijk”, 2, 4) returns “abghijk”
- Create a function that accepts the integer array described above returns the standard deviation of the values in a. The standard deviation is a statistical measure of the average distance each value in an array is from the mean. To calculate the standard deviation, you first call a second mean function that you need to write (do not use a built in gadget. Then sum the square of the difference of each value in the array a and that mean. The standard deviation is the square root of that sum divided by the number of elements in the array. Pay attention to what type of function your standard deviation and mean functions should be. As part of testing the functions, your main should display your test array in a square.
- Create a function frequencyCount that given a value target t, returns how many times t occurs in an integer array a. You need to write your own function, not a built in gadget.
- Create a function that returns the mode of the value in the array. You need to write your own, not use a built in gadget. The mode is the most frequently occurring value in the array. Your mode function will need to call your frequencycount function. To make things easier, if two or more different values have the same frequency count, we will simply return the smallest. Once you have tested your mode function with an array of length 100, test it with an array of length 10000 with randomly generated values between 0 and 9. I suggest not trying to display all 10000 values in the console window.
- Write code to move the integer values of each character (e.g. Ascii) in your string into an array of integers and calculate the mode of that array, which then should be converted back into a character.
- Write a function that accepts an array of integers and returns -1 if sorted in ascending order, 1 if sorted in descending order, and 0 if not sorted. To be as efficient as possible, your function should make only one pass through the array.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Assuming slogan is a char array which stores a valid C-String "Giants", the function call strlen(slogan) will return:arrow_forwardA C-string variable is an array, so we can use index operator [ ] to access individual character. True or false?arrow_forwardWrite a function named "changeCase" that takes an array of characters terminating by NULL character (C-string) and a boolean flag of toUpper. If the toUpper flag is true, it will go through the array and convert all lowercase characters to uppercase. Otherwise, it will convert all uppercase to lowercase. For example, if the array is {'H', 'e', 'l', 'l', 'o', '\0'} and the flag is true, then the array will become {'H', 'E', 'L', 'L', 'O', '\0'}. And if the flag is false, the array will become{'h', 'e', 'l', 'l', 'o', '\0'}arrow_forward
- vowelIndex Write a function vowelIndex that accepts a word (a str) as an argument and returns the index of the first vowel in the word. If there is no vowel, -1 is returned. For this problem, both upper and lower case vowels count, and 'y' is not considered to be a vowel. Sample usage: >>> vowelIndex('hello') 1 >>> vowelIndex('string') 3 >>> vowelIndex('string') 3 >>> vowelIndex('BUY') 1 >>> vowelIndex('APPLE') 0 >>> vowelIndex('nymphly') -1 >>> vowelIndex('string')==3 True >>> vowel Index ('nymphly')==-1 Truearrow_forwardmergeAndRemove(int[], int[]) This is a public static function that takes a int[] and int[] for the parameters and returns an int[]. Given two arrays of integers. Your job is to combine them into a single array and remove any duplicates, finally return the merged array.arrow_forward1. Write a program that defines a struct named Info. That Info struct should define two strings, a double and array of strings. In your main program, define a variable v using that Info struct and then initialize it to hold your first name, your last name, your lucky non-whole number (e.g. 26.5), your major, and a listing (an array) of your six most favorite college courses you have ever taken or plan to take. Then your program should access and print all the stored data held in the variable v. 2. print the data twice, once using the structure variable and do-while loop, and next using a pointer variable and a while-do loop.) Using the embed icon shown above show screenshots demoing the execution of your program.arrow_forward
- Match the std::string constructor on right to its function on left. Source: https://cplusplus.com/reference/string/string/string/ E string() string (const string& str) string (const char* s) string (size_t n, char c) [Choose] [Choose ] constructs a copy of str. constructs an empty string, with a length of zero characters. copies the null-terminated character sequence (C-string) pointed by s. fills the string with n consecutive copies of character c. [Choose ]arrow_forwardIn c++ Please Write the InOrder() function, which receives a vector of integers as a parameter, and returns true if the numbers are sorted (in order from low to high) or false otherwise. The program outputs "In order" if the vector is sorted, or "Not in order" if the vector is not sorted. Ex: If the vector passed to the InOrder() function is [5, 6, 7, 8, 3], then the function returns false and the program outputs: Not in order Ex: If the vector passed to the InOrder() function is [5, 6, #include <iostream>#include <vector>using namespace std; bool InOrder(vector<int> nums) { /* Type your code here */} int main() { vector<int> nums1(5); nums1.at(0) = 5; nums1.at(1) = 6; nums1.at(2) = 7; nums1.at(3) = 8; nums1.at(4) = 3; if (InOrder(nums1)) { cout << "In order" << endl; } else { cout << "Not in order" << endl; } vector<int> nums2(5); nums2.at(0) = 5; nums2.at(1) = 6;…arrow_forwardThe programming language is - Python a. Write a function called daysOver that takes three arguments: a dictionary, a location (such as ‘Sydney’, ‘Adelaide’,etc) and a temperature and returns the number of days that were over the given temperature for the given location. For example, if we call the function using the line: total = daysOver(dictionaryData, ‘Adelaide’, 40) total will hold the number of days that Adelaide had a temperature greater than 40 celsius in the data. As a test, there were 54 days over 40 celsius in the data. Check that total is 54 for the example when you run your code. b. Use the daysOver function to print the number of days over 35 celsius for each of the following cities: 'Adelaide','Perth','Melbourne','Canberra','Sydney','Brisbane','Darwin' c. Which of the Australian cities has the most number of days over 35 celsius? My spreadsheet looks like this Since I cannot attach an excel file, my excel file name is "weatherAUS.csv"arrow_forward
- happy Eidarrow_forward4. Complete the function show_upper. This function takes one parameter - a string (s). It should return a string made up of all the upper-case characters in s. For example, if s is “aBdDEfgHijK” then show_upper should return “BDEHK”. It should return the upper-case string - not print it. Do not change anything outside show_upper.arrow_forwardimport numpy as np import torch import matplotlib.pyplot as plt Quiz Preparation Question 1. Write a function that generates a random tensor of n x n (n is the input). The output is the mean of the tensor. = 2, 4, 8, 16, ... 1024 and generate a vector 2. Write a code (for loop) that call that calls the function above with n = M(n). Plot the vector as a function of the log of n. 3. Given an n x m array write a code that replaces every element that is larger than 0.5 with 0.5. 4. The second derivative of a function can be approximated by the finite difference 1 ƒ"(x₂) = 73 (ƒ(£;+1) — 2ƒ(x;) + f(x;-1)) Given a vector f with values f = [f(xo),..., f(n) write a code that computes the approximation to f"(x) 5. The power method is a method to compute the largest eigenvalue of a matrix. Your google search is using this method every time you perform a google search. The method consists of the iteration Vj+1 = Av; || Avi|| where v; is a vector with chosen as a random vector, and A is the matrix…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
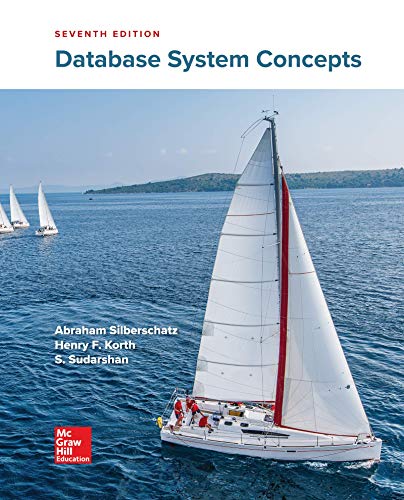
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
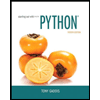
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
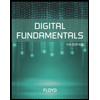
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
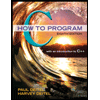
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
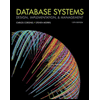
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
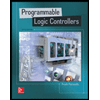
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education