import numpy as np import torch import matplotlib.pyplot as plt Quiz Preparation Question 1. Write a function that generates a random tensor of n x n (n is the input). The output is the mean of the tensor. 2. Write a code (for loop) that call that calls the function above with n = 2, 4, 8, 16,... 1024 and generate a vector M(n). Plot the vector as a function of the log of n. 3. Given an n x m array write a code that replaces every element that is larger than 0.5 with 0.5. 4. The second derivative of a function can be approximated by the finite difference ƒ"(x;) = 7/3 (ƒ(£;;1) — 2ƒ(2;) + ƒ(2;-1)) - h² Given a vector f with values f = [f(xo),..., f(n) write a code that computes the approximation to f"(x) 5. The power method is a method to compute the largest eigenvalue of a matrix. Your google search is using this method every time you perform a google search. The method consists of the iteration Avj Vj+1 || Av;|| where v; is a vector with v chosen as a random vector, and A is the matrix that we seek its eigenvalue/eigenvector. The iteration is terminated when ||Vj+1-vj|| < tol where tol is a specified tolerance. Write a python program that gets A, a matrix and tol, a tolerance and returns the estimated eigenvector v Note that to multiply matrix and a vector you can use the matmul(A,v) or A@v
import numpy as np import torch import matplotlib.pyplot as plt Quiz Preparation Question 1. Write a function that generates a random tensor of n x n (n is the input). The output is the mean of the tensor. 2. Write a code (for loop) that call that calls the function above with n = 2, 4, 8, 16,... 1024 and generate a vector M(n). Plot the vector as a function of the log of n. 3. Given an n x m array write a code that replaces every element that is larger than 0.5 with 0.5. 4. The second derivative of a function can be approximated by the finite difference ƒ"(x;) = 7/3 (ƒ(£;;1) — 2ƒ(2;) + ƒ(2;-1)) - h² Given a vector f with values f = [f(xo),..., f(n) write a code that computes the approximation to f"(x) 5. The power method is a method to compute the largest eigenvalue of a matrix. Your google search is using this method every time you perform a google search. The method consists of the iteration Avj Vj+1 || Av;|| where v; is a vector with v chosen as a random vector, and A is the matrix that we seek its eigenvalue/eigenvector. The iteration is terminated when ||Vj+1-vj|| < tol where tol is a specified tolerance. Write a python program that gets A, a matrix and tol, a tolerance and returns the estimated eigenvector v Note that to multiply matrix and a vector you can use the matmul(A,v) or A@v
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.6: The Standard Template Library (stl)
Problem 8E
Related questions
Question

Transcribed Image Text:import numpy as np
import torch
import matplotlib.pyplot as plt
Quiz Preparation Question
1. Write a function that generates a random tensor of n x n (n is the input). The output is the mean of the tensor.
= 2, 4, 8, 16, ... 1024 and generate a vector
2. Write a code (for loop) that call that calls the function above with n =
M(n).
Plot the vector as a function of the log of n.
3. Given an n x m array write a code
that replaces every element that is larger than 0.5 with 0.5.
4. The second derivative of a function can be approximated by the finite difference
1
ƒ"(x₂) = 73 (ƒ(£;+1) — 2ƒ(x;) + f(x;-1))
Given a vector f with values f = [f(xo),..., f(n) write a code that computes the approximation to f"(x)
5. The power method is a method to compute the largest eigenvalue of a matrix.
Your google search is using this method every time you perform a google search.
The method consists of the iteration
Vj+1 =
Av;
|| Avi||
where v; is a vector with chosen as a random vector, and A is the matrix that we seek its eigenvalue/eigenvector. The
iteration is terminated when
||Vj+1-vj|| < tol
where tol is a specified tolerance.
Write a python program that gets A, a matrix and tol, a tolerance and returns the estimated eigenvector v
Note that to multiply matrix and a vector you can use the matmul(A,v) or A@v
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
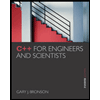
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
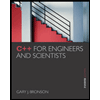
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr