//in c# // I am having problem with my regex fitting the requirments using System; using System.Text.RegularExpressions; namespace UsernameProcessor.Question { public sealed class UsernameProcessorService { /// /// Requirements: /// - A valid username shall be at least 4 characters.
//in c#
// I am having problem with my regex fitting the requirments
using System;
using System.Text.RegularExpressions;
namespace UsernameProcessor.Question
{
public sealed class UsernameProcessorService
{
/// <summary>
/// Requirements:
/// - A valid username shall be at least 4 characters.
/// - A valid username shall contain only letters, numbers and an optional underscore.
/// - A valid username shall start with a letter, and shall not end with an underscore.
/// </summary>
/// <param name="username"></param>
/// <returns>Whether or not the username is valid per the above requirements.</returns>
private static Regex sUserNameAllowedRegEx = new Regex(@"^(?=[a-zA-Z])[-\w.]{0,23}([a-zA-Z\d])$", RegexOptions.Compiled);
private static Regex sUserNameIllegalEndingRegEx = new Regex(@"(\-_)$", RegexOptions.Compiled);
public static bool IsValidUsername(string username)
{
if (string.IsNullOrEmpty(username)
|| !sUserNameAllowedRegEx.IsMatch(username)
|| sUserNameIllegalEndingRegEx.IsMatch(username))
{
return false;
}
throw new NotImplementedException("TODO: please validate our usernames!!!");
}
/// <summary>
/// Feel free to use the below main function to test and develop accordingly!
/// </summary>
public static void Main(string[] args)
{
Console.WriteLine(IsValidUsername("john_smith"));
Console.WriteLine(IsValidUsername("jsim_"));
}
}
}

Step by step
Solved in 3 steps with 2 images

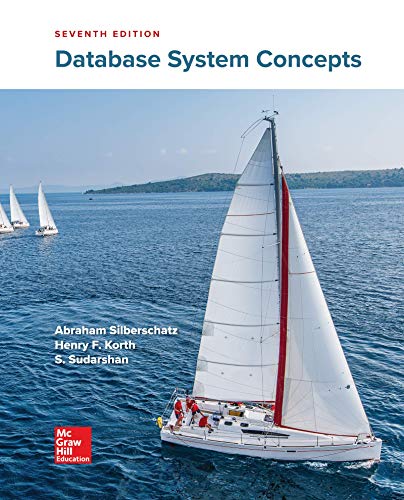
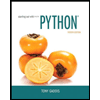
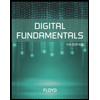
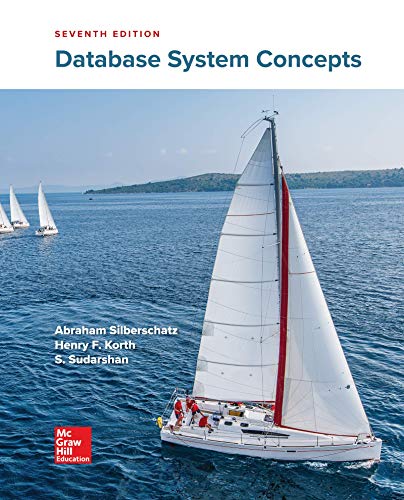
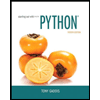
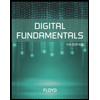
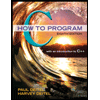
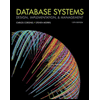
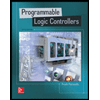