Implement a C++ program:RESTAURANT that has multiple branches, and each branch has menus of food items, their stock and a list of customers. A branch may have for example a breakfast menu and lunch menu with different food items, and the stock (available quantity) of each food item in the branch. Also, the branch will have a list of regular customers and their contact information to contact them for offers and new food items. Class Names Data and Member Functions Food Data Members: ID, Name, Calories, Price Member Functions: getID, getName, getCalories, getPrice setID, setName, setCalories, setPrice Stock Data Members: ID, Food, Stock Member Functions: getID, getFood, getStock setID, setFood, setStock Customer Data Members: ID, Name, Phone Member Functions: getID, getName, getPhone setID, setName, setPhoe Menu Data Members: ID, Name, foodList Member Functions: getID, getName, getFoodList setID, setName Branch Data Members: ID, Address, menuList, stockList, customerList Member Functions: getID, getAddress, getMenuList, getStockList, getCustomerList setID, setAddress After developing these classes, the following three parts of the project must be implemented: Part1: Create the following Linked Lists: foodList must store Food objects. stockList that stores Stock objects. customerList to store Customer objects. menuList to store Menu objects. RESTAURANT has branchList to store a Linked list of Branch objects with their menuList, stockList and customerList. The main function to test the above classes and data structures must display the following menu to the user: Main Menu: Branches Food Items Menus Customers The Submenu under each item of the Main Menu must have the following operations: Display Insert Delete Modify Find Part 2: Covert the above lists in part 1 into Stack. Part 3: Covert the above lists in part 1 into Queue
Implement a C++ program:
RESTAURANT that has multiple branches, and each branch has menus of food items, their stock and a list of customers. A branch may have for example a breakfast menu and lunch menu with different food items, and the stock (available quantity) of each food item in the branch. Also, the branch will have a list of regular customers and their contact information to contact them for offers and new food items. Class Names Data and Member Functions
- Food Data Members: ID, Name, Calories, Price Member Functions: getID, getName, getCalories, getPrice setID, setName, setCalories, setPrice
- Stock Data Members: ID, Food, Stock Member Functions: getID, getFood, getStock setID, setFood, setStock
- Customer Data Members: ID, Name, Phone Member Functions: getID, getName, getPhone setID, setName, setPhoe
- Menu Data Members: ID, Name, foodList Member Functions: getID, getName, getFoodList setID, setName
- Branch Data Members: ID, Address, menuList, stockList, customerList Member Functions: getID, getAddress, getMenuList, getStockList, getCustomerList setID, setAddress
After developing these classes, the following three parts of the project must be implemented:
Part1: Create the following Linked Lists:
- foodList must store Food objects.
- stockList that stores Stock objects.
- customerList to store Customer objects.
- menuList to store Menu objects.
- RESTAURANT has branchList to store a Linked list of Branch objects with their menuList, stockList and customerList.
The main function to test the above classes and data structures must display the following menu to the user:
Main Menu:
- Branches
- Food Items
- Menus
- Customers
The Submenu under each item of the Main Menu must have the following operations:
- Display
- Insert
- Delete
- Modify
- Find
Part 2: Covert the above lists in part 1 into Stack.
Part 3: Covert the above lists in part 1 into Queue
Unlock instant AI solutions
Tap the button
to generate a solution
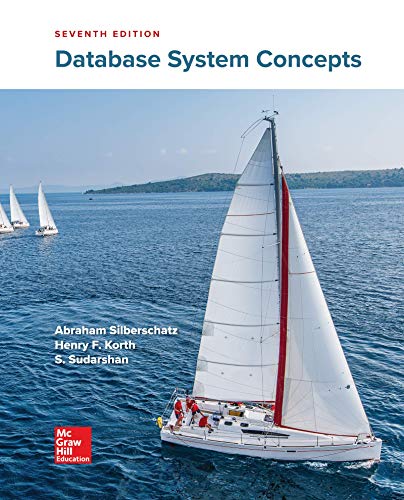
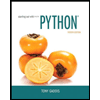
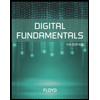
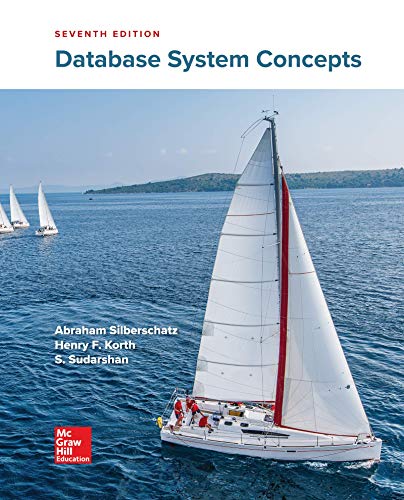
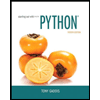
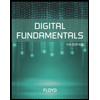
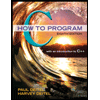
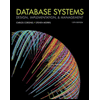
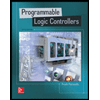