const express = require('express'); const app = express(); const server = require('http').createServer(app); const io = require('socket.io')(server); io.on('connection', (socket) => { console.log('Un cliente se ha conectado'); // Escucha el evento 'coords' socket.on('coords', (coords) => { }); }); console.log('Coords recibidas:', coords); server.listen(3000, () => { }); console.log('Server started on http://localhost:3000'); import { Injectable } from '@angular/core'; import ⋆ as mapboxgl from 'mapbox-gl'; import MapboxGeocoder from '@mapbox/mapbox-gl-geocoder'; import { environment } from '../environments/environment.prod'; import { EventEmitter } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { LocalizarService } from './localizar.service'; import { io, Socket } from 'socket.io-client'; @Injectable({ }) providedIn: 'root', export class MapaService { cbDireccion: EventEmitter = new EventEmitter(); marcadores: { [userId: string]: mapboxgl.Marker } = {}; mapbox = (mapboxgl as typeof mapboxgl); map: mapboxgl.Map; estilo = 'mapbox://styles/mapbox/streets-v12'; latitud = 16.808854263555794; longitud = -89.82447627429616; zoom= 13; puntosRuta: Array = []; private socket: Socket; constructor(private httpClient: HttpClient, private localizar: LocalizarService, ) { this.socket = io('http://localhost:3000'); } constructorMapa(): Promise { return new Promise((resolve, reject) => { try { this.mapbox.accessToken = environment.mapPk; // Asegúrate de asignar el accessToken this.map = new this.mapbox.Map({ }); container: 'map', style: this.estilo, center: [this.longitud, this.latitud], zoom: this.zoom const geocoder = new MapboxGeocoder({ accessToken: environment.mapPk, mapboxgl: this.mapbox }); geocoder.on('result', ($event) => { this.cbDireccion.emit($event.result); }); resolve({ map: this.map, geocoder: geocoder }); } catch (error) { reject(error); } }); } actualizarMarcador Vehiculo(userId: string, coords: any): void { let marker = this.marcadores [userId]; if (!marker) { } const el = this.crearElementoMarcador (userId); marker = new mapboxgl.Marker(el) .setLngLat(coords) .addTo(this.map); this.marcadores [userId] } else { } = marker; marker.setLngLat(coords); console.log( "Coordenadas", coords) this.socket.emit('coords', coords); crearElementoMarcador (userId: string): HTMLElement { const el = document.createElement('div'); el.className = 'marker'; el.style.width = '50px'; el.style.height '50px'; el.style.backgroundImage el.style.backgroundSize = 'url(/assets/iconos/enviado.png)'; = 'cover'; el.style.background Position 'center'; const label = document.createElement('div'); label.textContent = userId; label.style.position = 'absolute'; label.style.top = '-20px'; label.style.left = '50%'; label.style.transform = 'translateX(-50%)'; label.style.color = 'black'; label.style.fontWeight = 'bold'; el.appendChild(label); return el; } }
const express = require('express'); const app = express(); const server = require('http').createServer(app); const io = require('socket.io')(server); io.on('connection', (socket) => { console.log('Un cliente se ha conectado'); // Escucha el evento 'coords' socket.on('coords', (coords) => { }); }); console.log('Coords recibidas:', coords); server.listen(3000, () => { }); console.log('Server started on http://localhost:3000'); import { Injectable } from '@angular/core'; import ⋆ as mapboxgl from 'mapbox-gl'; import MapboxGeocoder from '@mapbox/mapbox-gl-geocoder'; import { environment } from '../environments/environment.prod'; import { EventEmitter } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { LocalizarService } from './localizar.service'; import { io, Socket } from 'socket.io-client'; @Injectable({ }) providedIn: 'root', export class MapaService { cbDireccion: EventEmitter = new EventEmitter(); marcadores: { [userId: string]: mapboxgl.Marker } = {}; mapbox = (mapboxgl as typeof mapboxgl); map: mapboxgl.Map; estilo = 'mapbox://styles/mapbox/streets-v12'; latitud = 16.808854263555794; longitud = -89.82447627429616; zoom= 13; puntosRuta: Array = []; private socket: Socket; constructor(private httpClient: HttpClient, private localizar: LocalizarService, ) { this.socket = io('http://localhost:3000'); } constructorMapa(): Promise { return new Promise((resolve, reject) => { try { this.mapbox.accessToken = environment.mapPk; // Asegúrate de asignar el accessToken this.map = new this.mapbox.Map({ }); container: 'map', style: this.estilo, center: [this.longitud, this.latitud], zoom: this.zoom const geocoder = new MapboxGeocoder({ accessToken: environment.mapPk, mapboxgl: this.mapbox }); geocoder.on('result', ($event) => { this.cbDireccion.emit($event.result); }); resolve({ map: this.map, geocoder: geocoder }); } catch (error) { reject(error); } }); } actualizarMarcador Vehiculo(userId: string, coords: any): void { let marker = this.marcadores [userId]; if (!marker) { } const el = this.crearElementoMarcador (userId); marker = new mapboxgl.Marker(el) .setLngLat(coords) .addTo(this.map); this.marcadores [userId] } else { } = marker; marker.setLngLat(coords); console.log( "Coordenadas", coords) this.socket.emit('coords', coords); crearElementoMarcador (userId: string): HTMLElement { const el = document.createElement('div'); el.className = 'marker'; el.style.width = '50px'; el.style.height '50px'; el.style.backgroundImage el.style.backgroundSize = 'url(/assets/iconos/enviado.png)'; = 'cover'; el.style.background Position 'center'; const label = document.createElement('div'); label.textContent = userId; label.style.position = 'absolute'; label.style.top = '-20px'; label.style.left = '50%'; label.style.transform = 'translateX(-50%)'; label.style.color = 'black'; label.style.fontWeight = 'bold'; el.appendChild(label); return el; } }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
How to issue coordinates through a websocket in angular version 17 websocket
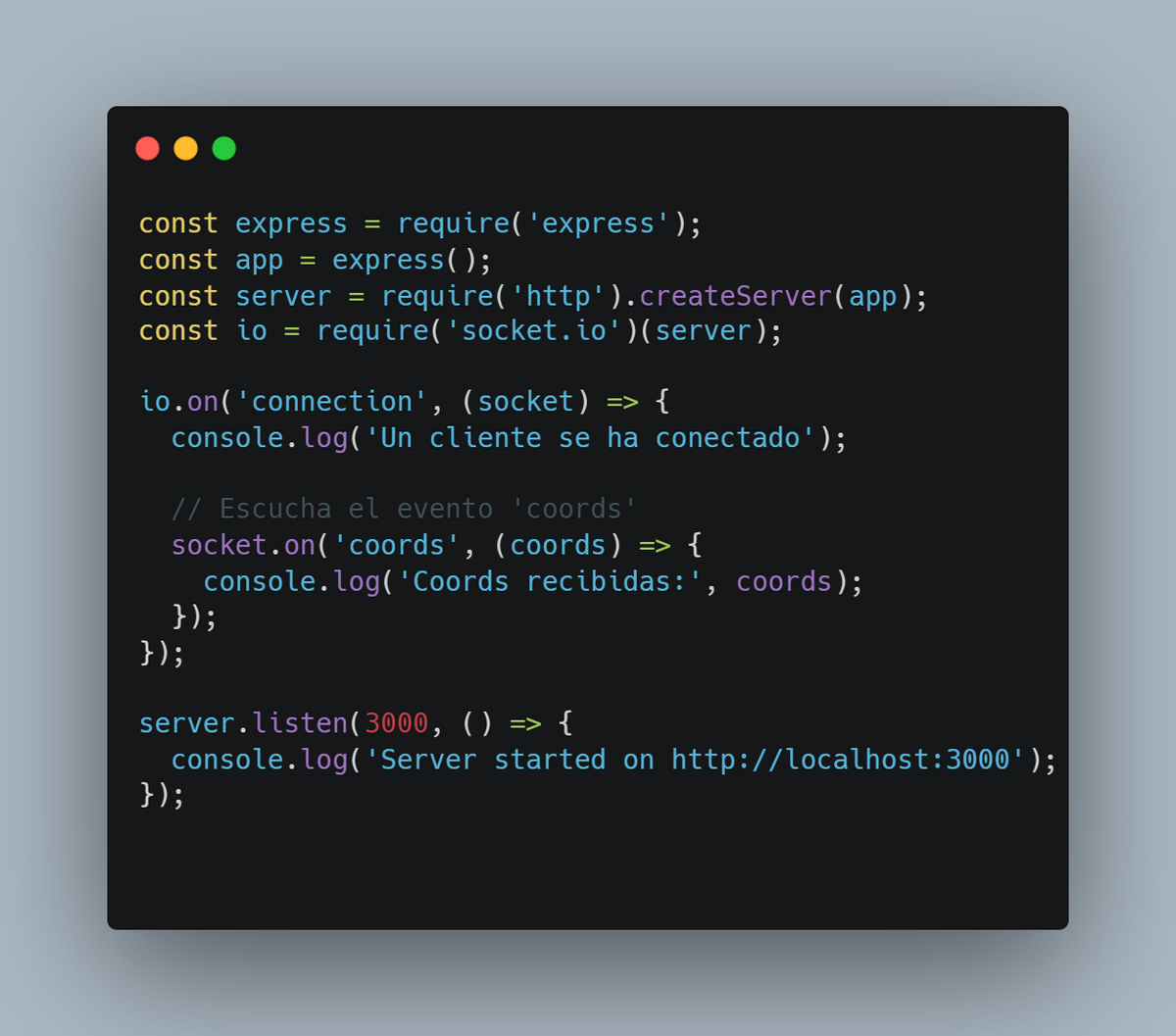
Transcribed Image Text:const express = require('express');
const app = express();
const server = require('http').createServer(app);
const io = require('socket.io')(server);
io.on('connection', (socket) => {
console.log('Un cliente se ha conectado');
// Escucha el evento 'coords'
socket.on('coords', (coords) => {
});
});
console.log('Coords recibidas:', coords);
server.listen(3000, () => {
});
console.log('Server started on http://localhost:3000');
![import { Injectable } from '@angular/core';
import ⋆ as mapboxgl from 'mapbox-gl';
import MapboxGeocoder from '@mapbox/mapbox-gl-geocoder';
import { environment } from '../environments/environment.prod';
import { EventEmitter } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { LocalizarService } from './localizar.service';
import { io, Socket } from 'socket.io-client';
@Injectable({
})
providedIn: 'root',
export class MapaService {
cbDireccion: EventEmitter<any> = new EventEmitter<any>();
marcadores: { [userId: string]: mapboxgl.Marker } = {};
mapbox = (mapboxgl as typeof mapboxgl);
map: mapboxgl.Map;
estilo = 'mapbox://styles/mapbox/streets-v12';
latitud = 16.808854263555794;
longitud = -89.82447627429616;
zoom= 13;
puntosRuta: Array<any> = [];
private socket: Socket;
constructor(private httpClient: HttpClient,
private localizar: LocalizarService,
) {
this.socket = io('http://localhost:3000');
}
constructorMapa(): Promise<any> {
return new Promise((resolve, reject) => {
try {
this.mapbox.accessToken
=
environment.mapPk; // Asegúrate de asignar el accessToken
this.map = new this.mapbox.Map({
});
container: 'map',
style: this.estilo,
center: [this.longitud, this.latitud],
zoom: this.zoom
const geocoder = new MapboxGeocoder({ accessToken: environment.mapPk, mapboxgl: this.mapbox });
geocoder.on('result', ($event) => {
this.cbDireccion.emit($event.result);
});
resolve({ map: this.map, geocoder: geocoder });
} catch (error) {
reject(error);
}
});
}
actualizarMarcador Vehiculo(userId: string, coords: any): void {
let marker = this.marcadores [userId];
if (!marker) {
}
const el =
this.crearElementoMarcador (userId);
marker = new mapboxgl.Marker(el)
.setLngLat(coords)
.addTo(this.map);
this.marcadores [userId]
} else {
}
=
marker;
marker.setLngLat(coords);
console.log( "Coordenadas", coords)
this.socket.emit('coords', coords);
crearElementoMarcador (userId: string): HTMLElement {
const el = document.createElement('div');
el.className = 'marker';
el.style.width
=
'50px';
el.style.height '50px';
el.style.backgroundImage
el.style.backgroundSize
=
'url(/assets/iconos/enviado.png)';
= 'cover';
el.style.background Position 'center';
const label = document.createElement('div');
label.textContent
=
userId;
label.style.position = 'absolute';
label.style.top = '-20px';
label.style.left = '50%';
label.style.transform = 'translateX(-50%)';
label.style.color = 'black';
label.style.fontWeight = 'bold';
el.appendChild(label);
return el;
}
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F81a7aec7-0d9f-4793-bed3-5a53e2519f44%2F9cf9804a-bdc0-4a35-85fe-3c102f653bc7%2F4bb1iob_processed.png&w=3840&q=75)
Transcribed Image Text:import { Injectable } from '@angular/core';
import ⋆ as mapboxgl from 'mapbox-gl';
import MapboxGeocoder from '@mapbox/mapbox-gl-geocoder';
import { environment } from '../environments/environment.prod';
import { EventEmitter } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { LocalizarService } from './localizar.service';
import { io, Socket } from 'socket.io-client';
@Injectable({
})
providedIn: 'root',
export class MapaService {
cbDireccion: EventEmitter<any> = new EventEmitter<any>();
marcadores: { [userId: string]: mapboxgl.Marker } = {};
mapbox = (mapboxgl as typeof mapboxgl);
map: mapboxgl.Map;
estilo = 'mapbox://styles/mapbox/streets-v12';
latitud = 16.808854263555794;
longitud = -89.82447627429616;
zoom= 13;
puntosRuta: Array<any> = [];
private socket: Socket;
constructor(private httpClient: HttpClient,
private localizar: LocalizarService,
) {
this.socket = io('http://localhost:3000');
}
constructorMapa(): Promise<any> {
return new Promise((resolve, reject) => {
try {
this.mapbox.accessToken
=
environment.mapPk; // Asegúrate de asignar el accessToken
this.map = new this.mapbox.Map({
});
container: 'map',
style: this.estilo,
center: [this.longitud, this.latitud],
zoom: this.zoom
const geocoder = new MapboxGeocoder({ accessToken: environment.mapPk, mapboxgl: this.mapbox });
geocoder.on('result', ($event) => {
this.cbDireccion.emit($event.result);
});
resolve({ map: this.map, geocoder: geocoder });
} catch (error) {
reject(error);
}
});
}
actualizarMarcador Vehiculo(userId: string, coords: any): void {
let marker = this.marcadores [userId];
if (!marker) {
}
const el =
this.crearElementoMarcador (userId);
marker = new mapboxgl.Marker(el)
.setLngLat(coords)
.addTo(this.map);
this.marcadores [userId]
} else {
}
=
marker;
marker.setLngLat(coords);
console.log( "Coordenadas", coords)
this.socket.emit('coords', coords);
crearElementoMarcador (userId: string): HTMLElement {
const el = document.createElement('div');
el.className = 'marker';
el.style.width
=
'50px';
el.style.height '50px';
el.style.backgroundImage
el.style.backgroundSize
=
'url(/assets/iconos/enviado.png)';
= 'cover';
el.style.background Position 'center';
const label = document.createElement('div');
label.textContent
=
userId;
label.style.position = 'absolute';
label.style.top = '-20px';
label.style.left = '50%';
label.style.transform = 'translateX(-50%)';
label.style.color = 'black';
label.style.fontWeight = 'bold';
el.appendChild(label);
return el;
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
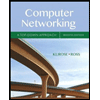
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
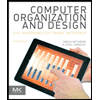
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
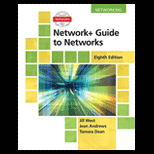
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
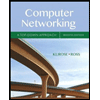
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
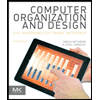
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
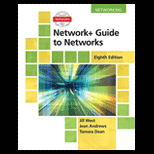
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
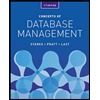
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
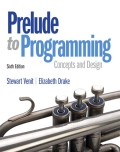
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
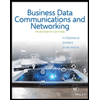
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY