I JUST WANT TO MAKE SURE IF MY HOMEWORK ANSWERS ARE CORRECT C++ HOMEWORKS Write the program Write a function to output a pyramid like this * *****. ********* Answer: #include using namespace std; void printPyramid(int n) { for (int i = 0; i < n; i++) { for (int j = 0; j < n - i - 1; j++) { cout << " "; } for (int j = 0; j <= i; j++) { cout << "*"; } for (int j = 0; j < i; j++) { cout << "*"; } cout << endl; } } int main() { printPyramid(4); return 0; } Write a function to count how many numbers in one string.. Answer: #include #include using namespace std; int countNumbers(string str) { int count = 0; for (char c : str) { if (isdigit(c)) { count++; } } return count; } int main() { string str = "Hello, World, 00000"; cout << countNumbers(str) << endl; return 0; } Write a recursive function that returns the nth number in a fibonacci sequence when n is passed to function. The fibonacci sequence is like 0,1,1,2,3,5,8,13...... Answer: #include using namespace std; int getFibonacci(int n) { if (n == 0 || n == 1) return n; else return getFibonacci(n - 1) + getFibonacci(n - 2); } int main() { int n = 7; int result = getFibonacci(n); cout << result; } Write a function to change all the upper case to lower case in a given string. Answer: #include #include using namespace std; string toLowerCase(string str) { for (char& c : str) { if (isupper(c)) { c = tolower(c); } } return str; } int main() { string str = "HI, MY NAME IS KHALED MOHAMMED!"; cout << toLowerCase(str) << endl; return 0; } Write a function to show the max number in ten input numbers. Answer: #include #include using namespace std; int numbers[] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; int maxNumber = *max_element(numbers, numbers + 10); int findMax(int a, int b, int c, int d, int e, int f, int g, int h, int i, int j) { int max = a; if (b > max) { max = b; } if (c > max) { max = c; } if (d > max) { max = d; } if (e > max) { max = e; } if (f > max) { max = f; } if (g > max) { max = g; } if (h > max) { max = h; } if (i > max) { max = i; } if (j > max) { max = j; } return max; } int main() { cout << findMax(1, 2, 3, 4, 5, 6, 7, 8, 9, 10) << endl; return 0; } .Write a function to convert a given string : Answer: #include #include using namespace std; void toUpper(string &str) { for (int i = 0; i < str.length(); i++) { str[i] = toupper(str[i]); } } void toLower(string &str) { for (int i = 0; i < str.length(); i++) { str[i] = tolower(str[i]); } } int main() { string str = "khaled"; toUpper(str); cout << str << endl; str = "MOHAMMED"; toLower(str); cout << str << endl; return 0; } Write a function to calculate the sum of the even number from 1 - 100. Answer: #include using namespace std; int sumEven() { int sum = 0; for (int i = 1; i <= 100; i++) { if (i % 2 == 0) { sum += i; } } return sum; } int main() { cout << sumEven() << endl; return 0; } Write a function to convert a decimal number to a binary number. Answer: #include #include using namespace std; string decToBin(int dec) { string bin = ""; while (dec > 0) { bin = to_string(dec % 2) + bin; dec /= 2; } return bin; } int main() { cout << decToBin(10) << endl; return 0; } Write a function to calculate s =1!+2!+3!+....+10! Answer: #include using namespace std; int findFactSum(int n) { int f = 1, Sum = 0; for (int i = 1; i <= n; i++) { f = f * i; Sum += f; } return Sum; } int main() { int n = 10; cout << findFactSum(n); return 0; } Write a function to realize the binary search algorithm. Answer: #include #include using namespace std; int binarySearch(vector& arr, int x) { int left = 0; int right = arr.size() - 1; while (left <= right) { int mid = left + (right - left) / 2; if (arr[mid] == x) { return mid; } if (arr[mid] > x) { right = mid - 1; } else { left = mid + 1; } } return -1; } int main() { vector arr = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; cout << binarySearch(arr, 5) << endl; return 0; }
I JUST WANT TO MAKE SURE IF MY HOMEWORK ANSWERS ARE CORRECT
C++ HOMEWORKS
Write the program
- Write a function to output a pyramid like this
*
*****.
*********
Answer:
#include <iostream>
using namespace std;
void printPyramid(int n) {
for (int i = 0; i < n; i++) {
for (int j = 0; j < n - i - 1; j++) {
cout << " ";
}
for (int j = 0; j <= i; j++) {
cout << "*";
}
for (int j = 0; j < i; j++) {
cout << "*";
}
cout << endl;
}
}
int main() {
printPyramid(4);
return 0;
}
- Write a function to count how many numbers in one string..
Answer:
#include <iostream>
#include <string>
using namespace std;
int countNumbers(string str) {
int count = 0;
for (char c : str) {
if (isdigit(c)) {
count++;
}
}
return count;
}
int main() {
string str = "Hello, World, 00000";
cout << countNumbers(str) << endl;
return 0;
}
- Write a recursive function that returns the nth number in a fibonacci sequence when n is passed to function. The fibonacci sequence is like 0,1,1,2,3,5,8,13......
Answer:
#include <iostream>
using namespace std;
int getFibonacci(int n) {
if (n == 0 || n == 1)
return n;
else
return getFibonacci(n - 1) + getFibonacci(n - 2);
}
int main() {
int n = 7;
int result = getFibonacci(n);
cout << result;
}
- Write a function to change all the upper case to lower case in a given string.
Answer:
#include <iostream>
#include <string>
using namespace std;
string toLowerCase(string str) {
for (char& c : str) {
if (isupper(c)) {
c = tolower(c);
}
}
return str;
}
int main() {
string str = "HI, MY NAME IS KHALED MOHAMMED!";
cout << toLowerCase(str) << endl;
return 0;
}
- Write a function to show the max number in ten input numbers.
Answer:
#include <iostream>
#include <
using namespace std;
int numbers[] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
int maxNumber = *max_element(numbers, numbers + 10);
int findMax(int a, int b, int c, int d, int e, int f, int g, int h, int i, int j) {
int max = a;
if (b > max) {
max = b;
}
if (c > max) {
max = c;
}
if (d > max) {
max = d;
}
if (e > max) {
max = e;
}
if (f > max) {
max = f;
}
if (g > max) {
max = g;
}
if (h > max) {
max = h;
}
if (i > max) {
max = i;
}
if (j > max) {
max = j;
}
return max;
}
int main() {
cout << findMax(1, 2, 3, 4, 5, 6, 7, 8, 9, 10) << endl;
return 0;
}
- .Write a function to convert a given string :
Answer:
#include <iostream>
#include <string>
using namespace std;
void toUpper(string &str)
{
for (int i = 0; i < str.length(); i++)
{
str[i] = toupper(str[i]);
}
}
void toLower(string &str)
{
for (int i = 0; i < str.length(); i++)
{
str[i] = tolower(str[i]);
}
}
int main()
{
string str = "khaled";
toUpper(str);
cout << str << endl;
str = "MOHAMMED";
toLower(str);
cout << str << endl;
return 0;
}
- Write a function to calculate the sum of the even number from 1 - 100.
Answer:
#include <iostream>
using namespace std;
int sumEven() {
int sum = 0;
for (int i = 1; i <= 100; i++) {
if (i % 2 == 0) {
sum += i;
}
}
return sum;
}
int main() {
cout << sumEven() << endl;
return 0;
}
- Write a function to convert a decimal number to a binary number.
Answer:
#include <iostream>
#include <string>
using namespace std;
string decToBin(int dec) {
string bin = "";
while (dec > 0) {
bin = to_string(dec % 2) + bin;
dec /= 2;
}
return bin;
}
int main() {
cout << decToBin(10) << endl;
return 0;
}
- Write a function to calculate s =1!+2!+3!+....+10!
Answer:
#include <iostream>
using namespace std;
int findFactSum(int n)
{
int f = 1, Sum = 0;
for (int i = 1; i <= n; i++) {
f = f * i;
Sum += f;
}
return Sum;
}
int main()
{
int n = 10;
cout << findFactSum(n);
return 0;
}
- Write a function to realize the binary search algorithm.
Answer:
#include <iostream>
#include <
using namespace std;
int binarySearch(vector<int>& arr, int x) {
int left = 0;
int right = arr.size() - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == x) {
return mid;
}
if (arr[mid] > x) {
right = mid - 1;
}
else {
left = mid + 1;
}
}
return -1;
}
int main() {
vector<int> arr = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
cout << binarySearch(arr, 5) << endl;
return 0;
}

Step by step
Solved in 4 steps with 2 images

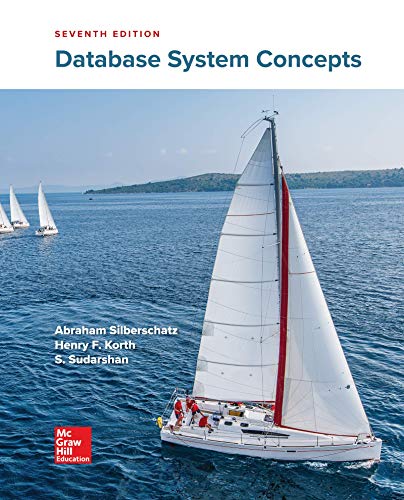
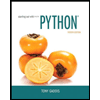
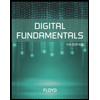
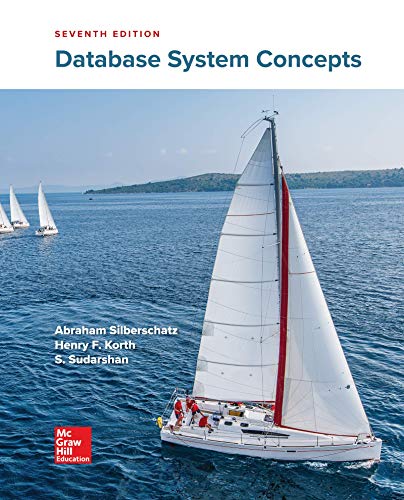
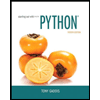
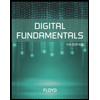
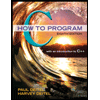
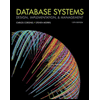
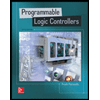