I have my code below and I am stuck for days at displaying the number of available burgers and customer waiting. What should be edited to make the program work? sample output is given below.
I have my code below and I am stuck for days at displaying the number of available burgers and customer waiting. What should be edited to make the program work? sample output is given below.
import java.util.Scanner;
import java.util.Random;
class Exam implements Runnable {
private String cName = new String("");
public Exam (String cName, int x) {
this.cName = cName;
this.x = x;
}
static int x;
@Override
public void run() {
Random r = new Random();
int j=0, avail=0;
for(int i = 1; i <= this.x; i++) {
Exam e[] = new Exam [this.x];
if (cName == "Consumer #1" || cName == "Consumer #2"){
if (i > 0 && j == 0){
System.out.println(cName + " of Thread #" + i + " buys 1 burger # of available burgers [" + avail + "] Customer(s) waiting [" + j++ + "]");
}else{
avail = i - 1;
System.out.println(cName + " of Thread #" + i + " buys 1 burger # of available burgers [" + avail + "] Customer(s) waiting [" + j++ + "]");
}
}else {
int num = this.x * 2;
avail = i - 1;
for(i = 1; i <= num; i++){
System.out.println(cName + " is cooking burger #" + i + "\t\t\t # of available burgers [" + i + "] Customer(s) waiting [" + j + "]");
}
}
}
}
}
public class MidtermExam{
public static void main(String args[]){
Scanner sc = new Scanner(System.in);
System.out.print("# of burgers to cook per consumer: ");
int input = sc.nextInt();
System.out.println();
Exam r1 = new Exam("Producer",input);
Thread Producer = new Thread(r1);
Producer.start();
Exam r2 = new Exam("Consumer #1",input);
Thread Consumer1 = new Thread(r2);
Consumer1.start();
Exam r3 = new Exam("Consumer #2",input);
Thread Consumer2 = new Thread(r3);
Consumer2.start();
}
}
![Sample output: Assume the system generates 4
Command Prompt
# of burgers to cook per Consumer = 4
Producer is Cooking Burger #1
Producer is Cooking Burger #2
Consumer #1 of Thread# 1 buys 1 burger
Consumer #2 of Thread# 1 buys 1 burger
Producer is Cooking Burger #3
Consumer #1 of Thread# 2 buys 1 burger
Producer is Cooking Burger #4
Producer is Cooking Burger #5
Producer is Cooking Burger #6
Consumer #3 of Thread# 1 buys 1 burger
Producer is Cooking Burger #7
Consumer #2 of Thread# 2 buys 1 burger
Consumer #3 of Thread# 2 buys 1 burger
Producer is Cooking Burger #8
Consumer #4 of Thread# 1 buys 1 burger
Consumer #4 of Thread# 2 buys 1 burger
# of Available burgers [1] Customer(s) Waiting: [0]
# of Available burgers [2] Customer(s) Waiting: [0]
# of Available burgers [1] Customer(s) Waiting: [0]
# of Available burgers [0] Customer(s) Waiting: [0]
# of Available burgers [1] Customer(s) Waiting: [0]
# of Available burgers [0] Customer(s) Waiting: [0]
# of Available burgers [1] Customer(s) Waiting: [0]
# of Available burgers [2] Customer(s) Waiting: [0]
# of Available burgers [3] Customer(s) Waiting: [0]
# of Available burgers [2] Customer(s) Waiting: [0]
# of Available burgers [3] Customer(s) Waiting: [0]
# of Available burgers [2] Customer(s) Waiting: [0]
# of Available burgers [1] Customer(s) Waiting:
# of Available burgers [2] Customer(s) Waiting: [0]
# of Available burgers [1] Customer(s) Waiting: [0]
# of Available burgers [0] Customer(s) Waiting: [0]
Sample output: Assume the
system generates 2
%3D
Command Prompt
# of burgers to cook per Consumer = 2
Producer is Cooking Burger #1
Consumer #1 of Thread# 1 buys 1 burger
Consumer #1 of Thread# 2 buys 1 burger
Consumer #2 of Thread# 1 buys 1 burger
Producer is Cooking Burger #2
Consumer #2 of Thread# 2 buys 1 burger
Producer is Cooking Burger #3
Producer is Cooking Burger #4
# of Available burgers [1] Customer(s) Waiting: [0]
# of Available burgers [0] Customer(s) Waiting: [0]
# of Available burgers [0] Customer(s) Waiting: [1]
# of Available burgers [0] Customer(s) Waiting: [2]
# of Available burgers [0] Customer(s) Waiting: [1]
# of Available burgers [0] Customer(s) Waiting: [2]
# of Available burgers [0] Customer(s) Waiting: [1]
# of Available burgers [0] Customer(s) Waiting: [0]
Note:
|Customer(s) Waiting: [1]
Since There are no more Available burgers created by the Producer Thread, the Customer “waits" !
until the Producer makes a new Burger.
Producer is Cooking Burger #2 # of Available burgers [0] Customer(s) Waiting: [1]
Although the Producer creates a new Burger, It will not be counted on the “Available" because
there were 2 customer(s) waiting. That's why on this line, Waiting was instead decremented
from 2 (see previous line) to 1.
Waiting: [1]
The same concept happened here.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fca2180eb-e455-463f-9a9e-dd04cac7e66f%2F0262482c-47f1-41be-b7b6-608d45221653%2Fjdmnqwd_processed.jpeg&w=3840&q=75)


Step by step
Solved in 3 steps with 1 images

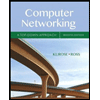
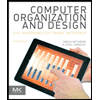
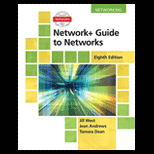
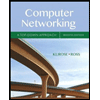
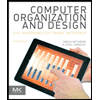
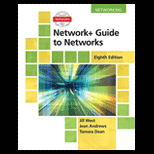
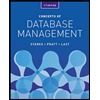
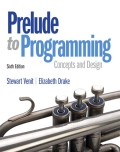
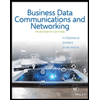