I am trying to make a while loop that checks for a condition from user input. If the user inputs anything except for valid integers, the while loop requires the user to reenter until a valid integer is input. I have tested this code, but the while loop isn't overwriting the maxNotNumber variable when reentered. Please tell me what I'm doing wrong. let min = 1; //minimum number let score = 0; let max = Math.ceil(prompt("Enter a maximum number 1 or greater for the guessing game."));//asks for user's max number input and rounds any decimals up maxNotNumber = Number.isInteger(max);//checks for nonnumbers console.log(maxNotNumber); //initial user max number input let random = Math.ceil(Math.random() * (max - min) + min); console.log(random); //randomly generated number between 1 and user max while (maxNotNumber != true) {//Illegal number check maxNotNumber = Number.isInteger(max);//checks for nonnumbers max = prompt("That's not a number. Try again."); console.log("This is from the while loop for maxNotNumber. " + max); // while (max < 1) {//Less than 1 check // max = prompt("Needs to be 1 or greater."); // } }//max number parameter check let guess = parseInt(prompt("Now guess the random number."));//asks user to guess randomly generated number, converts string to integer if necessary let isNumber = Number.isInteger(guess);//Checks that input from user is integer while (guess > max) { let score = score++;//increments score each wrong guess guess = prompt("Your guess is too high. Try again."); while (guess < max) { score = score++;//increments score each wrong guess prompt("Your guess is too low. Try again."); console.log("This is the console.log for score. " + score) } }
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
I am trying to make a while loop that checks for a condition from user input. If the user inputs anything except for valid integers, the while loop requires the user to reenter until a valid integer is input. I have tested this code, but the while loop isn't overwriting the maxNotNumber variable when reentered. Please tell me what I'm doing wrong.
let min = 1;
//minimum number
let score = 0;
let max = Math.ceil(prompt("Enter a maximum number 1 or greater for the guessing game."));//asks for user's max number input and rounds any decimals up
maxNotNumber = Number.isInteger(max);//checks for nonnumbers
console.log(maxNotNumber);
//initial user max number input
let random = Math.ceil(Math.random() * (max - min) + min);
console.log(random);
//randomly generated number between 1 and user max
while (maxNotNumber != true) {//Illegal number check
maxNotNumber = Number.isInteger(max);//checks for nonnumbers
max = prompt("That's not a number. Try again.");
console.log("This is from the while loop for maxNotNumber. " + max);
// while (max < 1) {//Less than 1 check
// max = prompt("Needs to be 1 or greater.");
// }
}//max number parameter check
let guess = parseInt(prompt("Now guess the random number."));//asks user to guess randomly generated number, converts string to integer if necessary
let isNumber = Number.isInteger(guess);//Checks that input from user is integer
while (guess > max) {
let score = score++;//increments score each wrong guess
guess = prompt("Your guess is too high. Try again.");
while (guess < max) {
score = score++;//increments score each wrong guess
prompt("Your guess is too low. Try again.");
console.log("This is the console.log for score. " + score)
}
}

Step by step
Solved in 2 steps

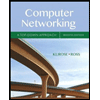
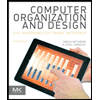
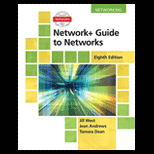
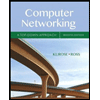
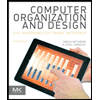
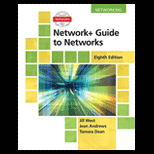
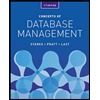
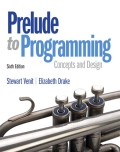
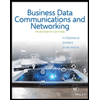