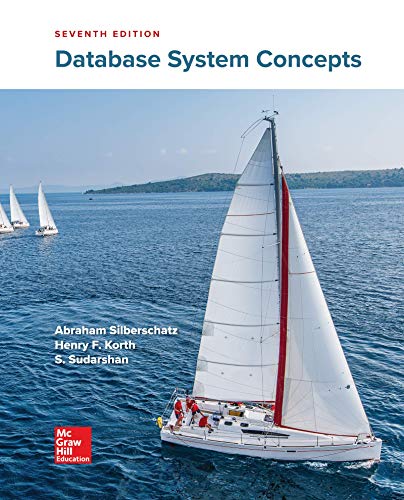
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
I am having trouble figuring out part b. Part b is important because the calculate button determines the entire problem, and I cannot figure out how to make it so it shows any text in the total due box. This is what I have for code so far, and none of it has 'errors', it just doesn't properly work.
Public Class frmMain
Private Function GetBusiness(ByVal dblConnections As Double) As Double
' Calculate the amount due.
Dim dblAmountDue As Double
dblAmountDue = 106.5
If dblConnections >= 10 Then
dblAmountDue += 4 * (dblConnections - 10)
End If
Return dblAmountDue
End Function
Private Function GetResidential(ByVal dblChannels As Double) As Double
' Calculate the amount due.
Dim dblAmountDue As Double
dblAmountDue = 34.5
If dblChannels >= 1 Then
dblAmountDue += 5 * (dblChannels - 1)
End If
Return dblAmountDue
End Function
Private Sub frmMain_Load(sender As Object, e As EventArgs) Handles MyBase.Load
' Populate lists.
For intPremium As Integer = 0 To 20 ' Step 1
lstPremium.Items.Add(intPremium)
Next intPremium
lstPremium.SelectedIndex = 0
For intConnections As Integer = 0 To 100 ' Step 2
lstConnections.Items.Add(intConnections)
Next intConnections
lstConnections.SelectedIndex = 0
End Sub
Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click
Me.Close()
End Sub
Private Sub btnCalc_Click(sender As Object, e As EventArgs) Handles btnCalc.Click
' Display the amount due.
Dim dblTotalDue As Double
Dim dblPremium As Double
Dim dblConnections As Double
Dim dblChannels As Double
Double.TryParse(txtTotalDue.Text, dblTotalDue)
Double.TryParse(lstPremium.SelectedItem.ToString, dblPremium)
Double.TryParse(lstConnections.SelectedItem.ToString, dblConnections)
Dim dblAmountDue As Double
' Determine which radio button is selected and assign the fee.
If radResidential.Checked = True Then
dblAmountDue = 34.5
ElseIf dblChannels >= 1 Then
dblAmountDue += 5 * (dblChannels - 1)
ElseIf radBusiness.Checked = True Then
dblAmountDue = 106.5
ElseIf dblConnections >= 10 Then
dblAmountDue += 4 * (dblConnections - 10)
End If
End Sub
Private Sub radBusiness_CheckedChanged(sender As Object, e As EventArgs) Handles radBusiness.CheckedChanged
txtTotalDue.Text = String.Empty
End Sub
Private Sub radResidential_CheckedChanged(sender As Object, e As EventArgs) Handles radResidential.CheckedChanged
txtTotalDue.Text = String.Empty
End Sub
Private Sub frmMain_FormClosing(sender As Object, e As System.Windows.Forms.FormClosingEventArgs) Handles Me.FormClosing
' Verify that the user wants to exit the application.
Dim dlgButton As DialogResult
dlgButton = MessageBox.Show("Do you want to exit?",
"Chambers Sales",
MessageBoxButtons.YesNo,
MessageBoxIcon.Exclamation)
' If the No button is selected, do not close the form.
If dlgButton = DialogResult.No Then
e.Cancel = True
End If
End Sub
End Class
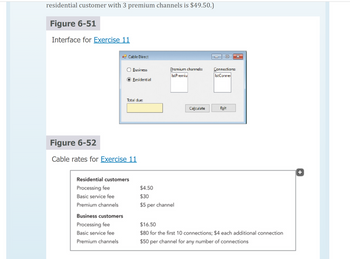
Transcribed Image Text:residential customer with 3 premium channels is $49.50.)
Figure 6-51
Interface for Exercise 11
Cable Direct
Business customers
Processing fee
Basic service fee
Premium channels
Business
Residential
Total due:
Figure 6-52
Cable rates for Exercise 11
Residential customers
Processing fee
Basic service fee
Premium channels
Premium channels:
Ist Premiu
$4.50
$30
$5 per channel
Calculate
Connections:
Ist Conne
Exit
$16.50
$80 for the first 10 connections; $4 each additional connection
$50 per channel for any number of connections
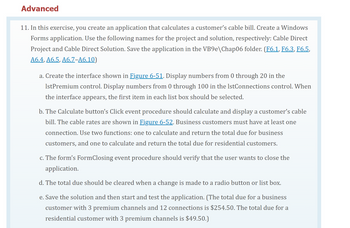
Transcribed Image Text:Advanced
11. In this exercise, you create an application that calculates a customer's cable bill. Create a Windows
Forms application. Use the following names for the project and solution, respectively: Cable Direct
Project and Cable Direct Solution. Save the application in the VB9e\Chap06 folder. (F6.1, F6.3, F6.5,
A6.4, A6.5, A6.7-A6.10)
a. Create the interface shown in Figure 6-51. Display numbers from 0 through 20 in the
IstPremium control. Display numbers from 0 through 100 in the IstConnections control. When
the interface appears, the first item in each list box should be selected.
b. The Calculate button's Click event procedure should calculate and display a customer's cable
bill. The cable rates are shown in Figure 6-52. Business customers must have at least one
connection. Use two functions: one to calculate and return the total due for business
customers, and one to calculate and return the total due for residential customers.
c. The form's FormClosing event procedure should verify that the user wants to close the
application.
d. The total due should be cleared when a change is made to a radio button or list box.
e. Save the solution and then start and test the application. (The total due for a business
customer with 3 premium channels and 12 connections is $254.50. The total due for a
residential customer with 3 premium channels is $49.50.)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Assignment 5B: Keeping Score. Now that we know about arrays, we can keep track of when events occur during our program. To prove this, let's create a game of Rock-Paper-Scissors. For those not familiar with this game, the basic premise is that each player says "Rock-Paper- Scissors!" and then makes their hand into the shape of one of the three objects. Winners are determined based on the following rules: Rock beats Scissors Scissors beats Paper Paper beats Rock At the start of the program, it will ask the player how many rounds of Rock-Paper- Scissors they want to play. After this, the game will loop for that many number of times. Each loop, it will ask the player what item they want to use – Rock, Paper, or Scissors. The computer will randomly generate its own item, and a winner will be determined. The game will then save the result as an element of an array, and the next round will begin. Once all the rounds have been played, the program will say "Game Over" and display a list of who…arrow_forwardMay assignment is asking me to build a program in PyChart that makes it possible for the player to visit each room and select the items in that room to fight a giant at the end. In order to win the game you have to collect all of the items from all of the rooms. When I run the code this is the result: Text Adventure Game ***************************Welcome to the Giant text game, Collect all of the items to defeat the Giant!To move: South, North, East, WestTo get items: get ’item name’ ***************************You are in the BasementItem: You see ArmorEnter a move: When I input the direction to go north the next result comes up correctly. However when I tell it to get and item (which is the command written into the code I get this: Invalid direction! Please enter a valid direction. ***************************You are in the BasementItem: You see ArmorEnter a move: This is my complete program code, can someone please tell me what I am doing wrong. #Functions showing goal of game and…arrow_forwardCould you help me with this C++ code please: Modify the class Song you created by doing thefollowing:(a) Add methods set_title(title), set_artist(artist) andset_year(year) that set the title, artist and recording year of thesong to the given values. The first two methods take a string as argument, the third one takes an integer.(b) Add a method set(title, artist, year) that sets the title,artist and recording year of the song to the given values. The firsttwo arguments are strings, the third one is an integer.(c) Replace the equals method by an equality operator (==).(d) Replace the less_than method by a less-than operator (<).(e) Replace the print method by an output operator (<<).(f) Replace the read method by an input operator (>>).(g) Organize your code into separate files as explained in Section 2.4 ofthe notes.Once again, you’ll need to decide how arguments should be passed to themethods and operators.Declare methods to be constant, as appropriate. Declare methods…arrow_forward
- In python and include doctring: First, write a class named Movie that has four data members: title, genre, director, and year. It should have: an init method that takes as arguments the title, genre, director, and year (in that order) and assigns them to the data members. The year is an integer and the others are strings. get methods for each of the data members (get_title, get_genre, get_director, and get_year). Next write a class named StreamingService that has two data members: name and catalog. the catalog is a dictionary of Movies, with the titles as the keys and the Movie objects as the corresponding values (you can assume there aren't any Movies with the same title). The StreamingService class should have: an init method that takes the name as an argument, and assigns it to the name data member. The catalog data member should be initialized to an empty dictionary. get methods for each of the data members (get_name and get_catalog). a method named add_movie that takes a Movie…arrow_forwardExercise 5 - Number to Word Make a new class in the Lab2 project called Num2wordthat prompts the user enter a number between 0 and 9 and then displays the corresponding word (i.e. "zero"for 0, "one"for 1, etc.). Use a switchstatement to do this. Include a default case that lets the user know they entered a wrong number. The switch statement has the following syntax: switch (variable) { case value1: // Code that should execute when variable==value1 break; // this prevents it from automatically going to the next case case value2: // Code that should execute when variable==value2 break; // this prevents it from automatically going to the next case default: // Code that should execute when variable has a value that didn't match the above }arrow_forwardHelp with codearrow_forward
- For your homework assignment, build a simple application for use by a local retail store. Your program should have the following classes: Item: Represents an item a customer might buy at the store. It should have two attributes: a String for the item description and a float for the price. This class should also override the __str__ method in a nicely formatted way. Customer: Represents a customer at the store. It should have three attributes: a String for the customer's name, a Boolean for the customer's preferred status, and a list of 5 indexes to hold Item objects. Include the following methods: make_purchase: accepts a String and a double as parameters to represent the name and price of an item this customer is purchasing. Create a new Item object with this info and append it to the internal list. If the customer is a preferred customer based on the Boolean attribute's value, take 10% off the total sale price. __str__: Override this method to print the customer's name and every…arrow_forwardInstructor note: This lab is part of the assignment for this chapter. This lab uses two Java files, LabProgram.java and SimpleCar.java. The SimpleCar class has been developed and provided to you already. You don't need to change anything in that class. Your job is to use the SimpleCar class to complete the specified tasks in the main() method of LabProgram.java Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar object that performs the following operations: Drives input number of miles forward Drives input number of miles in reverse Honks the horn Reports car status The SimpleCar class is found in the file SimpleCar.java. Ex: If the input is: 100 4 the output is: beep beep Car has driven: 96 milesarrow_forwardtrue of false: a) The first step in any programming task is to start coding. b) The only places we would ever want to put a comment in our code are above a method and above a class.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
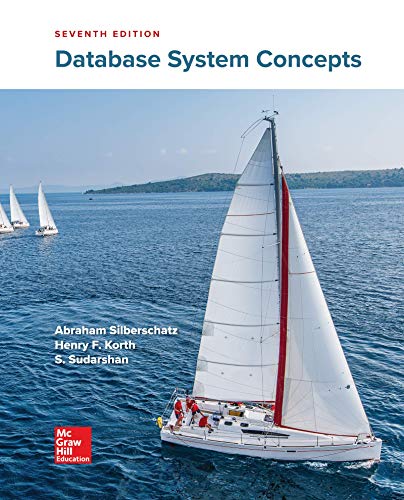
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
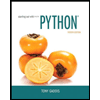
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
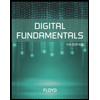
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
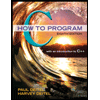
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
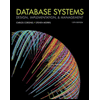
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
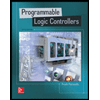
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education