Hi, would you be able to check my code and see if it meet the intended requirements, if not, please assist and correct any error in it for me. Below is the code and the requirements. Thank you. #Spefication/Requirement 1. The program consists of a single class called Loops 2. Loops has a main method 3. Loops has the following static methods a. calculateSum – the input to this method is an integer. The methods returns the sum 1+2+3+…+n. Use a for loop to calculate the sum b. calculateProduct – the input to this method is an integer. The method returns 1*2*3*…*n. Use a while loop to calculate the product. c. calculatePower – the input to this method is a double value, say x, and an integer value – say n. The method calculates and returns xy . Use a for loop to do the calculation. d. menu – this method requires no input parameters. It provides the user the following choices: 1. Calculate sum 1+2+3+4 2. Calculate product 1*2*3*…*n 3. CalculateXToYPower 4. QUIT The method returns the user’s selection. 4. In the main method, you should implement the following: main() { display menu and get user’s choice (1,2,3,4) LOOP (Use a while or do-while) if the choice is 1 ask the user to enter an integer – say n, then read the input from the user call calculateSum to get sum 1+2+3+…+n print n and the sum if the choice is 2 ask the user to enter an integer – say n, then read the input from the user call calculateProduct to get the product 1*2*3*…*n print n and the product if the choice is 3 ask the user to enter a double, say x, then read the input ask the user to enter an integer – say n, then read the input call calculatePower to get xn print the answer if the choice is 4, terminate the program (You can say System.exit(0); display menu again and get new choice END LOOP #Code import java.util.Scanner; public class Loops { public static int calculateSum(int n) { int sum = 0; for (int i = 1; i <= n; i++) { sum += i; } return sum; } public static double calculateProduct(int n) { double product = 1; int i = 1; while (i <= n) { product *= i; i++; } return product; } public static double calculatePower(double x, int n) { double result = 1; for (int i = 0; i < n; i++) { result *= x; } return result; } public static int menu() { System.out.println("\n1. Calculate sum 1+2+3+...+n"); System.out.println("2. Calculate product 1*2*3*...*n"); System.out.println("3. Calculate X to the power of Y"); System.out.println("4. QUIT"); System.out.print("Enter your choice: "); Scanner scanner = new Scanner(System.in); return scanner.nextInt(); } public static void main(String[] args) { int choice = menu(); while (choice != 4) { Scanner scanner = new Scanner(System.in); if (choice == 1) { System.out.print("Enter an integer: "); int n = scanner.nextInt(); System.out.println("Sum: " + calculateSum(n)); } else if (choice == 2) { System.out.print("Enter an integer: "); int n = scanner.nextInt(); System.out.println("Product: " + calculateProduct(n)); } else if (choice == 3) { System.out.print("Enter a double: "); double x = scanner.nextDouble(); System.out.print("Enter an integer: "); int n = scanner.nextInt(); System.out.println("Power: " + calculatePower(x, n)); } choice = menu(); } } }
Hi, would you be able to check my code and see if it meet the intended requirements, if not, please assist and correct any error in it for me. Below is the code and the requirements. Thank you.
#Spefication/Requirement
1. The program consists of a single class called Loops
2. Loops has a main method
3. Loops has the following static methods
a. calculateSum – the input to this method is an integer. The methods returns
the sum 1+2+3+…+n. Use a for loop to calculate the sum
b. calculateProduct – the input to this method is an integer. The method
returns 1*2*3*…*n. Use a while loop to calculate the product.
c. calculatePower – the input to this method is a double value, say x, and an
integer value – say n. The method calculates and returns xy
. Use a for loop to do
the calculation.
d. menu – this method requires no input parameters. It provides the user the
following choices:
1. Calculate sum 1+2+3+4
2. Calculate product 1*2*3*…*n
3. CalculateXToYPower
4. QUIT
The method returns the user’s selection.
4. In the main method, you should implement the following:
main()
{
display menu and get user’s choice (1,2,3,4)
LOOP (Use a while or do-while)
if the choice is 1 ask the user to enter an integer – say n, then read the input from the user
call calculateSum to get sum 1+2+3+…+n
print n and the sum
if the choice is 2 ask the user to enter an integer – say n, then read the input from the user
call calculateProduct to get the product 1*2*3*…*n
print n and the product
if the choice is 3 ask the user to enter a double, say x, then read the input
ask the user to enter an integer – say n, then read the input
call calculatePower to get xn
print the answer
if the choice is 4, terminate the program (You can say System.exit(0);
display menu again and get new choice
END LOOP
#Code
public class Loops {
public static int calculateSum(int n) {
int sum = 0;
for (int i = 1; i <= n; i++) {
sum += i;
}
return sum;
}
public static double calculateProduct(int n) {
double product = 1;
int i = 1;
while (i <= n) {
product *= i;
i++;
}
return product;
}
public static double calculatePower(double x, int n) {
double result = 1;
for (int i = 0; i < n; i++) {
result *= x;
}
return result;
}
public static int menu() {
System.out.println("\n1. Calculate sum 1+2+3+...+n");
System.out.println("2. Calculate product 1*2*3*...*n");
System.out.println("3. Calculate X to the power of Y");
System.out.println("4. QUIT");
System.out.print("Enter your choice: ");
Scanner scanner = new Scanner(System.in);
return scanner.nextInt();
}
public static void main(String[] args) {
int choice = menu();
while (choice != 4) {
Scanner scanner = new Scanner(System.in);
if (choice == 1) {
System.out.print("Enter an integer: ");
int n = scanner.nextInt();
System.out.println("Sum: " + calculateSum(n));
} else if (choice == 2) {
System.out.print("Enter an integer: ");
int n = scanner.nextInt();
System.out.println("Product: " + calculateProduct(n));
} else if (choice == 3) {
System.out.print("Enter a double: ");
double x = scanner.nextDouble();
System.out.print("Enter an integer: ");
int n = scanner.nextInt();
System.out.println("Power: " + calculatePower(x, n));
}
choice = menu();
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

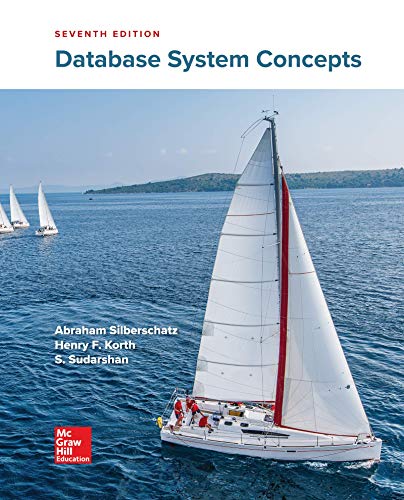
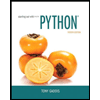
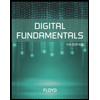
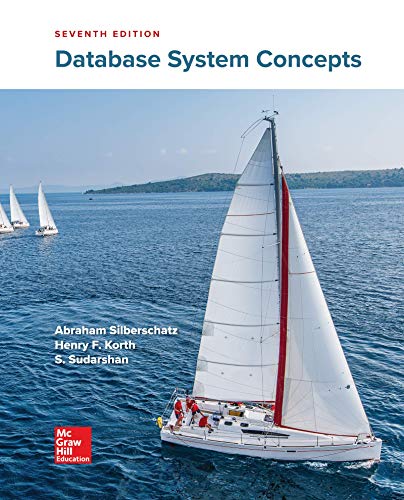
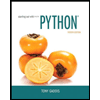
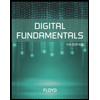
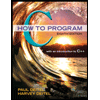
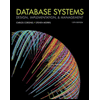
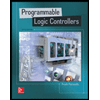