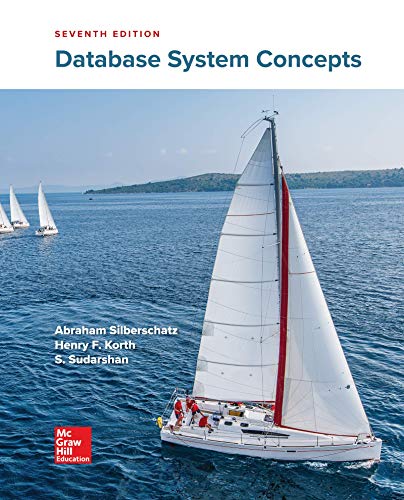
How could I get the code to answer another user as winner besides none?
class Mancala:
def __init__(self):
"""Initialize the game."""
# create the board
self.board = [0] * 14
# initialize the seed count
self.seed_count = 48
# player 1 starts the game
self.current_player = 1
def create_players(self):
"""Create the two players."""
# player 1 is the human player
self.player1 = Player("Human", 1)
# player 2 is the computer player
self.player2 = Player("Computer", 2)
def print_board(self):
"""Print the current state of the board."""
print("+-----+-----+-----+-----+-----+-----+")
print("| {} | {} | {} | {} | {} | {} |".format(*self.board[6:12]))
print("+-----+-----+-----+-----+-----+-----+")
print("| {} | {} | {} | {} | {} | {} |".format(*self.board[6:12]))
print("+-----+-----+-----+-----+-----+-----+")
def return_winner(self):
"""Return the winner of the game."""
# player 1 wins if the game is over and player 2 has no more seeds
if self.is_game_over() and self.board[6] == 0:
return self.player1
# player 2 wins if the game is over and player 1 has no more seeds
if self.is_game_over() and self.board[13] == 0:
return self.player2
# otherwise, the game is not over yet
return None
def play_game(self):
"""Play the game."""
# create the players
self.create_players()
# print the initial state of the board
self.print_board()
# while the game is not over, keep playing
while not self.is_game_over():
# get the next move from the current player
move = self.current_player.get_move(self)
# make the move
self.make_move(move)
# print the current state of the board
self.print_board()
# check if the game is over
if self.is_game_over():
# if so, return the winner
return self.return_winner()
# switch to the other player
self.current_player = self.player1 if self.current_player == self.player2 else self.player2
def make_move(self, move):
"""Make a move in the game."""
# get the start position and end position for the move
start = move[0]
end = move[1]
# get the number of seeds to move
seeds_to_move = self.board[start]
# clear the start position
self.board[start] = 0
# move the seeds
for i in range(start+1, start+seeds_to_move+1):
self.board[i % len(self.board)] += 1
# check if the last seed was moved into the player's mancala
if self.current_player.mancala_position == end:
# if so, the player gets another turn
return
# check if the last seed was moved into an empty pit on the player's side
if self.board[end] == 1 and end in self.current_player.pit_positions:
# if so, the player captures the seeds in the opposite pit
captured_seeds = self.board[self.get_opposite_pit(end)]
self.board[self.get_opposite_pit(end)] = 0
self.board[end] = 0
self.board[self.current_player.mancala_position] += captured_seeds
return
if self.board[end] == 1 and end not in self.current_player.pit_positions:
return
self.current_player = self.player1 if self.current_player == self.player2 else self.player2
def get_opposite_pit(self, pit_position):
return len(self.board) - pit_position - 1
def is_game_over(self):
return self.board[6] == 0 or self.board[13] == 0
class Player:
def __init__(self, name, player_id):
self.name = name
self.id = player_id
self.mancala_position = 6 if self.id == 1 else 13
self.pit_positions = list(range(0, 6)) if self.id == 1 else list(range(7, 13))
def get_move(self, mancala):
possible_moves = mancala.get_possible_moves()
if len(possible_moves) == 0:
return None
print("{}, please enter a move: ".format(self.name))
print("Possible moves: {}".format(possible_moves))
move = input()
return move
def main():
game = Mancala()
winner = game.play_game()
print("{} wins!".format(winner))
if __name__ == "__main__":
main()

Step by stepSolved in 2 steps

How would I get one of the players to win instead of none?
How would I get one of the players to win instead of none?
- Focus on classes, objects, methods and good programming style Your task is to create a BankAccount class(See the pic attached) The bank account will be protected by a 4-digit pin number (i.e. between 1000 and 9999). The pin should be generated randomly when the account object is created. The initial balance should be 0. get_pin()should return the pin. check_pin(pin) should check the argument against the saved pin and return True if it matches, False if it does not. deposit(amount) should receive the amount as the argument, add the amount to the account and return the new balance. withraw(amount) should check if the amount can be withdrawn (not more than is in the account), If so, remove the argument amount from the account and return the new balance if the transaction was successful. Return False if it was not. get_balance() should return the current balance. Finally, write a main() to demo your bank account class. Present a menu offering a few actions and perform the action the user…arrow_forwardmau Open Leathing inta... - The class has data members that can hold the name of your cube, length of one of the sides and the color of your cube. - The class has a constructor that accepts the name, length of one of the sides, and color as arguments and sets the data members to those values. - The class has the following methods to set the corresponding data member. setName(string newName) setSide(double newSide) setColor(string newColor) - The class has a method called getVolume() that returns the volume of the cube, calculated by cubing the length of the side. - The class has a method called volumelncrease(double newVolume) that receive the percent the volume should increase, and then the side to the corresponding value. i - For example, 2.5 indicates 2.5% increasing of the volume. So you take the current volume, increase it by 2.5%, and then find the cube root to calculate the new side length. You can use the built in function called cbrt, part of the math library, to find the cube…arrow_forwardsolve in python Street ClassStep 1:• Create a class to store information about a street:• Instance variables should include:• the street name• the length of the street (in km)• the number of cars that travel the street per day• the condition of the street (“poor”, “fair”, or “good”).• Write a constructor (__init__), that takes four arguments corresponding tothe instance variables and creates one Street instance. Street ClassStep 2:• Add additional methods:• __str__• Should return a string with the Street information neatly-formatted, as in:Elm is 4.10 km long, sees 3000 cars per day, and is in poor condition.• compare:• This method should compare one Street to another, with the Street the method is calledon being compared to a second Street passed to a parameter.• Return True or False, indicating whether the first street needs repairs more urgently thanthe second.• Streets in “poor” condition need repairs more urgently than streets in “fair” condition, whichneed repairs more…arrow_forward
- class SavingsAccount(object): RATE = 0.02 def __init__(self, name, pin, balance = 0.0): self._name = name self._pin = pin self._balance = balance def __str__(self): result = 'Name: ' + self._name + '\n' result += 'PIN: ' + self._pin + '\n' result += 'Balance: ' + str(self._balance) return result def __eq__(self,a): if self._name == a._name and self._pin == a._pin and self._balance == a._balance: return True else: return False def __gt__(self,a): if self._balance > a._balance: return True else: return False def getBalance(self): return self._balance def getName(self): return self._name def getPin(self): return self._pin def deposit(self, amount): self._balance += amount return self._balance…arrow_forwardAttributes item_name (string) item_price (int) item_quantity (int) Default constructor Initializes item's name="none", item's price = 0, item's quantity=0 Method print_item_cost() Ex of print_item_cost() output: Bottled Water 10 @ $1 = $10 in the main section of your code, prompt the user for two items and create two objects of the ItemToPurchase class. Exc Item 1 Enter the item name: Chocolate Chips Enter the item price: 3 Enter the item quantity: 1 Item 2 Enter the item name: Bottled Water Enter the item price: 1 Enter the item quantity: 10 Add the costs of the two items together and output the total cost Ex TOTAL COST Chocolate Chips 1 @ $3-$3 Bottled Water 10 @ $1= $10 Total: $13arrow_forwardclass Duration: def __init__(self, hours, minutes): self.hours = hours self.minutes = minutes def __add__(self, other): total_hours = self.hours + other.hours total_minutes = self.minutes + other.minutes if total_minutes >= 60: total_hours += 1 total_minutes -= 60 return Duration(total_hours, total_minutes) first_trip = Duration(3, 36)second_trip = Duration(0, 47) first_time = first_trip + second_tripsecond_time = second_trip + second_trip print(first_time.hours, first_time.minutes) what is the outputarrow_forward
- class Circle: """ A circle with a radius. """ def __init__(self, r: int) -> None: """Create a circle with a radius r. >>> c = Circle(10) >>> c.radius 10 """arrow_forward#ClubMember classclass ClubMember: #__init_ functiondef __init__(self,id,name,gender,weight,phone):self.id = idself.name = nameself.gender = genderself.weight = weightself.phone = phone #__str__ functiondef __str__(self):return "Id:"+str(self.id)+",Name:"+self.name+",Gender:"+self.gender+",Weight:"+str(self.weight)+",Phone:"+str(self.phone) #Club classclass Club: #__init__ functiondef __init__(self,name):self.name = nameself.members = {}self.membersCount = 0 def run(self):print("Welcome to "+self.name+"!")while True:print("""1. Add New Member2. View Member Info3. Search for a member4. Browse All Members5. Edit Member6. Delete a Member7. Exit""") choice = int(input("Choice:")) if choice == 1:self.membersCount = self.membersCount+1id = self.membersCountname = input("Member name:")gender = input("Gender:")weight = int(input("Weight:"))phone = int(input("Phone No.")) member = ClubMember(id,name,gender,weight,phone)self.members[id] = memberelif choice == 2:name = input("Enter name of…arrow_forward3- Create a class named Player with a default name as "player", a default level as 0, and a default class type of "fighter". Feel free to add any other attributes you wish! Create an instance method in the Player class called attack that takes another player as a parameter. This function should compare the level of the two players, and return whether the player won, lost, or tied. Finally create a loop that allows the user to input a name and a class type for two players; the level of both players should be determined at random (between 1 and 99). Print out the name and attributes of each player, and have the first player attack the second. Print whether the player won, lost, or tied. Ask if the user wants to try again. (Python code)arrow_forward
- class Student: def _init_(self, firstname, lastname, idnum): self.first = firstname self.last = lastname self.id = idnum self.courses = [] def add_course(self, course): ''' don't allow overloads or signing up for the same course twice if course not in self.courses and len(self.courses) = 4 What are all of the attributes of this class? last overload idnum add_course self first course firstname courses id clear_courses lastnamearrow_forwardFor your homework assignment, build a simple application for use by a local retail store. Your program should have the following classes: Item: Represents an item a customer might buy at the store. It should have two attributes: a String for the item description and a float for the price. This class should also override the __str__ method in a nicely formatted way. Customer: Represents a customer at the store. It should have three attributes: a String for the customer's name, a Boolean for the customer's preferred status, and a list of 5 indexes to hold Item objects. Include the following methods: make_purchase: accepts a String and a double as parameters to represent the name and price of an item this customer is purchasing. Create a new Item object with this info and append it to the internal list. If the customer is a preferred customer based on the Boolean attribute's value, take 10% off the total sale price. __str__: Override this method to print the customer's name and every…arrow_forwardTime This class is going to represent a 24-hour cycle clock time. That means 0:0:0 (Hour:Minute:Second) is the beginning of a day and 23:59:59 is the end of the day. This class has these data members. int sec int min int hourarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
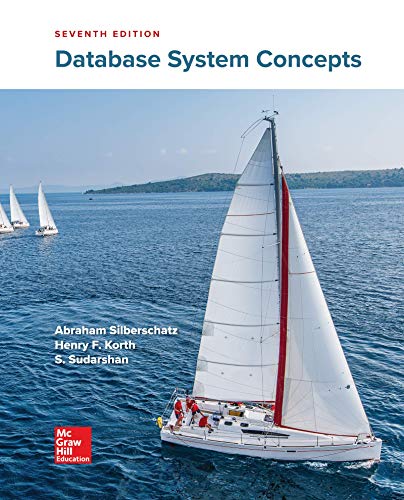
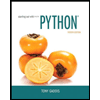
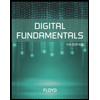
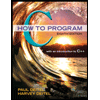
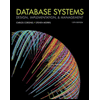
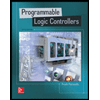