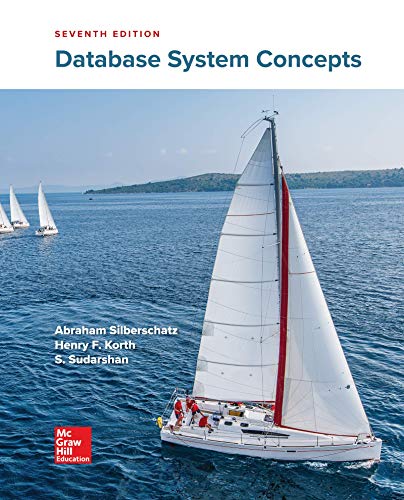
How to write a c++ program and run it N= 250, 500, 1000 times etc? I have an
Code is here: please edit it and paste whole code including mine here.
#include <iostream> #include <stdlib.h> #include <time.h> using namespace std; //randInt function to generate random numbers between 1 to 5 int randInt(int i, int j) { int num=(rand()%(j-i+1))+i; return num; } //main function int main() { srand(time(0)); //Algorithm 1 //initializing array 'a' to 0 int a[5]={0,0,0,0,0}; int a_size=sizeof(a)/sizeof(a[0]); //iterating through the array and generating random number until it is not already in the array for(int i=0;i<a_size;i++){ int num; while(i>=0){ //calling randInt function num=randInt(1,5); //if num is already in the array, skipping the iteration if(num==a[0] || num==a[1] || num==a[2] || num==a[3] || num==a[4]){ continue; } //else, breaking the loop else{ break; } } //adding num to array a[i]=num; } //printing the array cout<<"Array 'a' after using Algorithm 1: "<<endl; for(int i=0;i<a_size;i++){ cout<<a[i]<<" "; } //Algorithm 2 //assigning array 'a' to 0 for(int i=0;i<a_size;i++){ a[i]=0; } //initializing used array to have all false values bool used[a_size]={false,false,false,false,false}; //iterating through the array for(int i=0;i<a_size;i++){ int num; while(i>=0){ //calling randInt function num=randInt(1,5); //if used[num] is false if(!used[num]){ //assigning it as true used[num]=true; //adding num to array a[i]=num; //breaking the loop break; } //else, skipping the iteration else{ continue; } } } //printing the array cout<<"\nArray 'a' after using Algorithm 2: "<<endl; for(int i=0;i<a_size;i++){ cout<<a[i]<<" "; } //Algorithm 3 //filling array 'a' with i+1 values for(int i=0;i<a_size;i++){ a[i]=i+1; } //swapping with random positions using swap() function for(int i=0;i<a_size;i++){ swap(a[i], a[randInt(0,i)]); } //printing the array cout<<"\nArray 'a' after using Algorithm 3: "<<endl; for(int i=0;i<a_size;i++){ cout<<a[i]<<" "; } return 0; }

Step by stepSolved in 3 steps

- Create a flowchart for this program in c++, #include <iostream>#include <vector> // for vectors#include <algorithm>#include <cmath> // math for function like pow ,sin, log#include <numeric>using std::vector;using namespace std;int main(){ vector <float> x, y;//vector x for x and y for y float x_tmp = -2.5; // initial value of x float my_function(float x); while (x_tmp <= 2.5) // the last value of x { x.push_back(x_tmp); y.push_back(my_function(x_tmp)); // calculate function's value for given x x_tmp += 1;// add step } cout << "my name's khaled , my variant is 21 ," << " my function is y = 0.05 * x^3 + 6sin(3x) + 4 " << endl; cout << "x\t"; for (auto x_tmp1 : x) cout << '\t' << x_tmp1;//printing x values with tops cout << endl; cout << "y\t"; for (auto y_tmp1 : y) cout << '\t' << y_tmp1;//printing y values with tops…arrow_forwardIn C write a grading program as follows.- Ask the user for the number of students and store it in an integer variable.- Create an array of floats with four rows and columns equal to the number of students storedearlier.- Initialize the array to zeros.Create a menu with the following options (use a do-while loop and repeatedly display the menu):A or a to add student info one student at a timeT or t to display class average for homeworkS or s to display class average for quizzesB or b to display class average for examsZ or z to exit program (program repeats until this exit command is entered)arrow_forwardin python create function that has a parameter of dict[str, str] key should be a name value should be a word when given a dict of names and word, function should return the word that is most common ******************************* no using key, value for loops no .get, .items, .defaultset no importing builtins no using .values, .keys, or .index must do it through dictionary cycling ************************** should return a stringarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
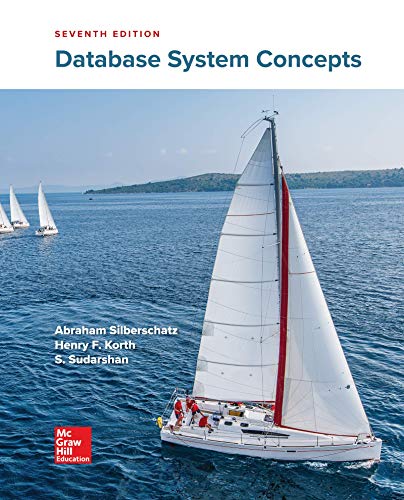
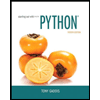
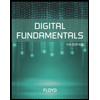
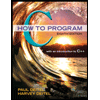
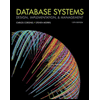
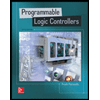