Please Use PYTHON def count_scrabble_points(user_input): """ The function ... """ tile_dict = { 'A': 1, 'B': 3, 'C': 3, 'D': 2, 'E': 1, 'F': 4, 'G': 2, 'H': 4, 'I': 1, 'J': 8, 'K': 5, 'L': 1, 'M': 3, 'N': 1, 'O': 1, 'P': 3, 'Q': 10, 'R': 1, 'S': 1, 'T': 1, 'U': 1, 'V': 4, 'W': 4, 'X': 8, 'Y': 4, 'Z': 10 } if __name__ == "__main__": ''' Type your code here. '''
Please Use PYTHON def count_scrabble_points(user_input): """ The function ... """ tile_dict = { 'A': 1, 'B': 3, 'C': 3, 'D': 2, 'E': 1, 'F': 4, 'G': 2, 'H': 4, 'I': 1, 'J': 8, 'K': 5, 'L': 1, 'M': 3, 'N': 1, 'O': 1, 'P': 3, 'Q': 10, 'R': 1, 'S': 1, 'T': 1, 'U': 1, 'V': 4, 'W': 4, 'X': 8, 'Y': 4, 'Z': 10 } if __name__ == "__main__": ''' Type your code here. '''
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please Use PYTHON
def count_scrabble_points(user_input):
"""
The function ...
"""
tile_dict = { 'A': 1, 'B': 3, 'C': 3, 'D': 2, 'E': 1, 'F': 4, 'G': 2, 'H': 4, 'I': 1, 'J': 8,
'K': 5, 'L': 1, 'M': 3, 'N': 1, 'O': 1, 'P': 3, 'Q': 10, 'R': 1, 'S': 1, 'T': 1,
'U': 1, 'V': 4, 'W': 4, 'X': 8, 'Y': 4, 'Z': 10 }
if __name__ == "__main__":
''' Type your code here. '''

Transcribed Image Text:### LAB ACTIVITY: Scrabble Points
#### Task Description:
In this lab activity, you will write a function to calculate the Scrabble points for a given word.
#### Code Snippet:
```python
def count_scrabble_points(user_input):
"""
The function ...
"""
tile_dict = {
'A': 1, 'B': 3, 'C': 3, 'D': 2, 'E': 1, 'F': 4, 'G': 2, 'H': 4, 'I': 1, 'J': 8,
'K': 5, 'L': 1, 'M': 3, 'N': 1, 'O': 1, 'P': 3, 'Q': 10, 'R': 1, 'S': 1, 'T': 1,
'U': 1, 'V': 4, 'W': 4, 'X': 8, 'Y': 4, 'Z': 10
}
if __name__ == "__main__":
''' Type your code here. '''
```
#### Instructions:
- Create a function `count_scrabble_points` that takes a string `user_input` as its parameter.
- Utilize `tile_dict` to find the corresponding point value for each letter in `user_input`.
- The function should calculate the total Scrabble score for the input word by summing the point values of each letter.
- Complete the code under `if __name__ == "__main__":` by calling the function and printing the result.
#### Objective:
By completing this task, you will understand how to:
- Use dictionaries to map characters to values.
- Perform operations on strings and iterate through them.
- Implement a simple scoring algorithm similar to the rules of the game Scrabble.

Transcribed Image Text:**Instructions**
Scrabble is a word game in which words are constructed from letter tiles, each letter tile containing a point value. The value of a word is the sum of each tile’s points added to any points provided by the word’s placement on the game board.
Write a program that takes a word as input and outputs the base total value of the word by calling `count_scrabble_points()`. Implement `count_scrabble_points()` to calculate the total points for the given string (the function’s parameter) and return this total.
### Example
If the input is:
`PYTHON`
the output is:
`14`
**Why 14?**
Because we look up the score for each letter and get:
```
'P': 3
'Y': 4
'T': 1
'H': 4
'O': 1
'N': 1
```
When we sum up the respective points, we get 14.
---
The image contains a Scrabble tile point distribution:
- A: 1, B: 3, C: 3, D: 2, E: 1, F: 4, G: 2
- H: 4, I: 1, J: 8, K: 5, L: 1, M: 3, N: 1
- O: 1, P: 3, Q: 10, R: 1, S: 1, T: 1, U: 1
- V: 4, W: 4, X: 8, Y: 4, Z: 10
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
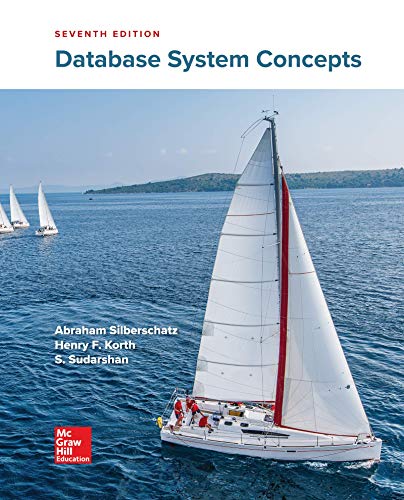
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
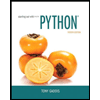
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
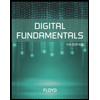
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
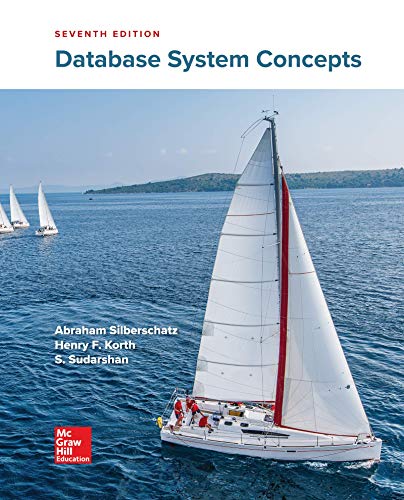
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
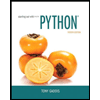
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
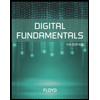
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
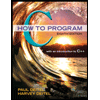
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
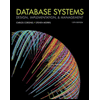
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
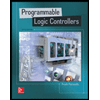
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education