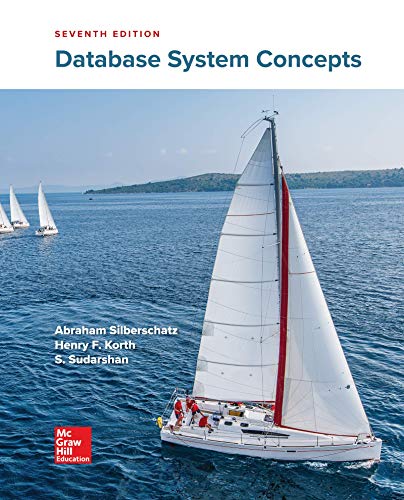
Concept explainers
How do I make the code run properly?
Code:
import java.util.*;
import java.util.Arrays;
public class Movie
{
private String movieName;
private int numMinutes;
private boolean isKidFriendly;
private int numCastMembers;
private String[] castMembers;
// default constructor
public Movie()
{
this.movieName = "Flick";
this.numMinutes = 0;
this.isKidFriendly = false;
this.numCastMembers = 0;
this.castMembers = new String[10];
}
// overloaded parameterized constructor
class movie{
public static void main(String args[]){
Movie obj=new Movie();
Movie obj1=new Movie(obj.getMovieName(),obj.getNumMinutes(),obj.isKidFriendly(),obj.getCastMembers());
System.out.println("Default Constructor: "+obj.getNumCastMembers());
System.out.println("Overloaded Constructor: "+obj1.getNumCastMembers());
}
}
// set the number of minutes
public void setNumMinutes(int numMinutes)
{
this.numMinutes = numMinutes;
}
// set the movie name
public void setMovieName(String movieName)
{
this.movieName = movieName;
}
// set if the movie is kid friendly or not
public void setIsKidFriendly(boolean isKidFriendly)
{
this.isKidFriendly = isKidFriendly;
}
// return the movie name
public String getMovieName()
{
return this.movieName;
}
// return the number of minutes
public int getNumMinutes()
{
return this.numMinutes;
}
// return true if movie is kid friendly else false
public boolean isKidFriendly()
{
return this.isKidFriendly;
}
// return the array of cast members
public String[] getCastMembers()
{
// create a deep copy of the array
String[] copyCastMembers = new String[this.castMembers.length];
// copy the strings from the array to the copy
for (int i = 0; i < this.castMembers.length; i++)
{
copyCastMembers[i] = this.castMembers[i];
}
return copyCastMembers;
}
// return the number of cast members
public int getNumCastMembers()
{
return this.numCastMembers;
}
// method that allows the name of a castMember at an index in the castMembers
// array to be changed
public boolean replaceCastMember(int index, String castMemberName)
{
if (index < 0 || index >= numCastMembers)
return false;
castMembers[index] = castMemberName;
return true;
}
// method that determines the equality of two String arrays and returns a
// boolean, by comparing the value at each index location.
// Return true if all elements of both arrays match, return false if there is
// any mismatch.
public boolean doArraysMatch(String[] arr1, String[] arr2)
{
if (arr1 == null && arr2 == null)
return true;
else if (arr1 == null || arr2 == null) // one of the array is null
return false;
else if (arr1.length != arr2.length) // length of arrays do not match
return false;
for (int i = 0; i < arr1.length; i++)
{
if (!arr1[i].equalsIgnoreCase(arr2[i]))
return false;
}
return true;
}
public String getCastMemberNamesAsString()
{
if (numCastMembers == 0)
{
return "none";
}
String names = castMembers[0];
for (int i = 1; i < numCastMembers; i++)
{
names += ", " + castMembers[i];
}
return names;
}
public String toString() {
String movie = "Movie: [ Minutes "+String.format("%03d", numMinutes)+" | Movie Name: %21s |";
if (isKidFriendly)
movie +=" is kid friendly |";
else
movie +=" not kid friendly |";
movie +=" Number of Cast Members: "+ numCastMembers+ " | Cast Members: "+getCastMemberNamesAsString()+" ]";
return String.format(movie,movieName);
}
public boolean equals(Object o)
{
// check if o is an instance of Movie
if(o instanceof Movie)
{
Movie other = (Movie)o;
// returns true if all the fields are equal
return((movieName.equalsIgnoreCase(other.movieName)) && (isKidFriendly == other.isKidFriendly) && (numMinutes == other.numMinutes)
&& (numCastMembers == other.numCastMembers) && (doArraysMatch(castMembers,other.castMembers)));
}
return false; // movies are not equal or o is not an object of Movie
}
public static void main(String args[])
{
}
}

Step by stepSolved in 3 steps with 1 images

- 2. The MyInteger Class Problem Description: Design a class named MyInteger. The class contains: n [] [] A private int data field named value that stores the int value represented by this object. A constructor that creates a MyInteger object for the specified int value. A get method that returns the int value. Methods isEven () and isOdd () that return true if the value is even or odd respectively. Static methods isEven (int) and isOdd (int) that return true if the specified value is even or odd respectively. Static methods isEven (MyInteger) and isOdd (MyInteger) that return true if the specified value is even or odd respectively. Methods equals (int) and equals (MyInteger) that return true if the value in the object is equal to the specified value. Implement the class. Write a client program that tests all methods in the class.arrow_forwardQ1. amount the same, y, n, why? public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { balance = balance + amount ; } // modified toString() method public String toString() { return "Account: " + actNum + "\tName: " + nameOnAct + "\tBalance: " + amount ; } } Q2. amount the same, y, n, why? public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { // scope of amount starts here balance = balance + amount ; // scope of amount ends here } public void processCheck( int amount ) { // scope of amount starts here int charge; incrementUse(); if ( balance < 100000 ) charge = 15; else charge = 0; balance = balance - amount - charge ; // scope of amount ends here }…arrow_forwardpublic class KnowledgeCheckTrek { public static final String TNG = "The Next Generation"; public static final String DS9 = "Deep Space Nine"; public static final String VOYAGER = "Voyager"; public static String trek(String character) { if (character != null && (character.equalsIgnoreCase("Picard") || character.equalsIgnoreCase("Data"))) { // IF ONE return TNG; } else if (character != null && character.contains("7")) { // IF TWO return VOYAGER; } else if ((character.contains("Quark") || character.contains("Odo"))) { // IF THREE return DS9; } return null; } public static void main(String[] args) { System.out.println(trek("Captain Picard")); System.out.println(trek("7 of Nine")); System.out.println(trek("Odo")); System.out.println(trek("Quark")); System.out.println(trek("Data").equalsIgnoreCase(TNG));…arrow_forward
- public class ItemToPurchase { private String itemName; private int itemPrice; private int itemQuantity; // Default constructor public ItemToPurchase() { itemName = "none"; itemPrice = 0; itemQuantity = 0; } // Mutator for itemName public void setName(String itemName) { this.itemName = itemName; } // Accessor for itemName public String getName() { return itemName; } // Mutator for itemPrice public void setPrice(int itemPrice) { this.itemPrice = itemPrice; } // Accessor for itemPrice public int getPrice() { return itemPrice; } // Mutator for itemQuantity public void setQuantity(int itemQuantity) { this.itemQuantity = itemQuantity; } // Accessor for itemQuantity public int getQuantity() { return itemQuantity; }} import java.util.Scanner; public class ShoppingCartPrinter { public static void main(String[] args) { Scanner scnr = new…arrow_forwardHow do I fix the Java code errors? Code: //import java.util.Arrays;//Movie.java package movie; public class Movie { private String movieName; private int numMinutes; private boolean isKidFriendly; private int numCastMembers; private String[] castMembers; public Movie() { movieName = "Flick"; numMinutes = 0; isKidFriendly = false; numCastMembers = 0; castMembers = new String[10]; } public Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) { this.movieName = movieName; this.numMinutes = numMinutes; this.isKidFriendly = isKidFriendly; this.numCastMembers = castMembers.length; this.castMembers = castMembers; } public String getMovieName() { return this.movieName; } public int getNumMinutes() { return this.numMinutes; } public boolean getIsKidFriendly() { return this.isKidFriendly; } public boolean isKidFriendly() {…arrow_forwardI have the following code: public class Length{private int feet;private int inches;// Your code goes here Length(){feet = inches = 0;} Length(int newFeet, int newInches){feet = newFeet;inches = newInches;} public int getFeet() { return feet; }public void setFeet(int newFeet) { feet = newFeet; } public int getInches() { return inches; }public void setInches(int newInches) { inches = newInches; } public Length add(Length otherLength){int newFeet = feet + otherLength.feet;int newInches = inches + otherLength.inches; if(newInches >= 12){newFeet++;newInches -= 12;} return (new Length(newFeet,newInches));} public Length subtract(Length otherLength){if(this.feet > otherLength.feet){int newFeet = feet - otherLength.feet;int newInches = inches - otherLength.inches;if(newInches < 0){newFeet--;newInches += 12;} return (new Length(newFeet,newInches));}else{int newFeet = otherLength.feet - feet;int newInches = otherLength.inches - inches;if(newInches < 0){newFeet--;newInches += 12;}…arrow_forward
- Complete the missing programming steps correctly:1. class Base {public void Print() {System.out.println("Base");}}class Derived_______ Base {public void Print() {i. System.out.println("Derived");}}class Main{public static void DoPrint( Base o ) {____________}public static void main(String[] args) {Base x = new Base();Base y = new Derived();___________________DoPrint(x);DoPrint(y);DoPrint(z);} }Output: BaseDerivedDerived2. Consider the following example code and generate the output. Give your explanation aboutgenerated output.class Base {public void foo() { System.out.println("Base"); }}class Derived extends Base {private void foo() { System.out.println("Derived"); }}public class Main {public static void main(String args[]) {Base b = new Derived();b.foo();}}3. Look at the below programming code and complete the missing parts to generate the givenoutput. Output: “Interface Method Implemented”interface Pet{_____________}class Dog implements Pet{public void…arrow_forward1. Insert the missing part of the code below to output the string. public class MyClass { public static void main(String[] args) { ("Hello, I am a Computer Engineer");arrow_forwardpublic class Plant { protected String plantName; protected String plantCost; public void setPlantName(String userPlantName) { plantName = userPlantName; } public String getPlantName() { return plantName; } public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; } public String getPlantCost() { return plantCost; } public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }} public class Flower extends Plant { private boolean isAnnual; private String colorOfFlowers; public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; } public boolean getPlantType(){ return isAnnual; } public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…arrow_forward
- import java.util.*; class Money { private int dollar; private int cent; final static int MIN_CENT_VALUE = 0; final static int MAX_CENT_VALUE = 99; public Money() { this.dollar = 0; this.cent = 0; } public Money(int dollar, int cent) { this.dollar = dollar; if (cent > MIN_CENT_VALUE && cent <= MAX_CENT_VALUE) { this.cent = cent; } else { cent = 0; } } public int getDollar() { return this.dollar; } public void setDollar(int dol) { this.dollar = dol; } public int getCent() { return this.cent; } public void setCent(int c) { if (c >= MIN_CENT_VALUE && c <= MAX_CENT_VALUE) { this.cent = c; } } public Money add(Money otherMoney) { Money m = new Money(); m.dollar = this.dollar + otherMoney.dollar; m.cent = this.cent + otherMoney.cent; if (m.cent >= 100) {…arrow_forwardHow do I fix the errors? Code: import java.util.Scanner;public class ReceiptMaker { public static final String SENTINEL = "checkout";public final int MAX_NUM_ITEMS;public final double TAX_RATE;private String[] itemNames;private double[] itemPrices;private int numItemsPurchased; public ReceiptMaker() {MAX_NUM_ITEMS = 10;TAX_RATE = 0.0875;itemNames = new String[MAX_NUM_ITEMS];itemPrices = new double[MAX_NUM_ITEMS];numItemsPurchased = 0;}public ReceiptMaker(int maxNumItems, double taxRate) {MAX_NUM_ITEMS = maxNumItems;TAX_RATE = taxRate;itemNames = new String[MAX_NUM_ITEMS];itemPrices = new double[MAX_NUM_ITEMS];numItemsPurchased = 0;} public void greetUser() {System.out.println("Welcome to the " + MAX_NUM_ITEMS + " items or less checkout line");}public void promptUserForProductEntry() {System.out.println("Enter item #" + (numItemsPurchased + 1) + "'s name and price separated by a space, or enter \"checkout\" to end transaction early");}public void addNextPurchaseItemFromUser(String…arrow_forwardSubclass toString should call include super.toString(); re-submit these codes. public class Vehicle { private String registrationNumber; private String ownerName; private double price; private int yearManufactured; Vehicle [] myVehicles = new Vehicle[100]; public Vehicle() { registrationNumber=""; ownerName=""; price=0.0; yearManufactured=0; } public Vehicle(String registrationNumber, String ownerName, double price, int yearManufactured) { this.registrationNumber=registrationNumber; this.ownerName=ownerName; this.price=price; this.yearManufactured=yearManufactured; } public String getRegistrationNumber() { return registrationNumber; } public String getOwnerName() { return ownerName; } public double getPrice() { return price; } public int getYearManufactured() { return yearManufactured; }…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
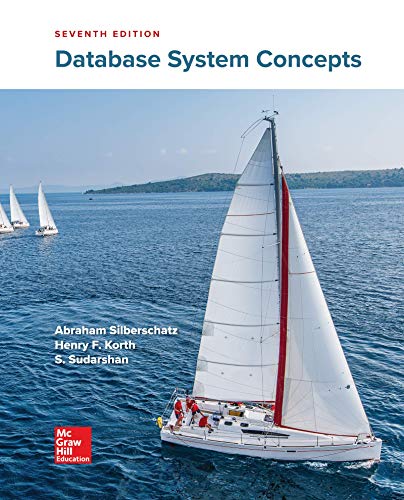
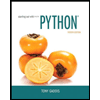
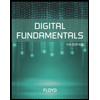
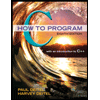
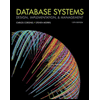
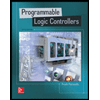