How do I create this in Java? Create the class Employee with int id, String name, double salary and int numberOfDependents as private attributes. Add the Setters and Getters for each of those attributes, and override the toString method to print an employee in the format [id,name,net salary], where: Net salary = salary*0.91 + (numberOfDependent *0.01*salary) In this program, two employee objects are equal when they have the same net salary. For that, override the equals method (the one inherited from Object) so that emp1.equals(emp2) is true when emp1 and emp2 are two employee objects that are equal. In the main method, declare list to be an ArrayList of type Employee, and add at least 3 employees. Sort and print the list in ascending order (with respect to the net salary). The code may use the Java sort static method from the Collections class. If so, you may either implement the Java Comparable interface or the Java Comparator interface. Below can be used as the code of your main method: Employee emp1 = new Employee(111, "Jimmy Dean", 5256.32, 0), emp2 = new Employee(598, "Jen Johnson", 47370, 5), emp3 = new Employee(920, "Jan Jones", 47834.25, 1); System.out.println(emp1.equals(emp3)); ArrayList list = new ArrayList<>(); list.add(emp1); list.add(emp2); list.add(emp3); //sort call goes here... for (Employee e : list) System.out.println(e);
How do I create this in Java?
Create the class Employee with int id, String name, double salary and int numberOfDependents as
private attributes. Add the Setters and Getters for each of those attributes, and override the toString
method to print an employee in the format [id,name,net salary], where:
Net salary = salary*0.91 + (numberOfDependent *0.01*salary)
In this program, two employee objects are equal when they have the same net salary. For that,
override the equals method (the one inherited from Object) so that emp1.equals(emp2) is true when
emp1 and emp2 are two employee objects that are equal.
In the main method, declare list to be an ArrayList of type Employee, and add at least 3 employees. Sort
and print the list in ascending order (with respect to the net salary). The code may use the Java sort
static method from the Collections class. If so, you may either implement the Java Comparable interface
or the Java Comparator interface.
Below can be used as the code of your main method:
emp2 = new Employee(598, "Jen Johnson", 47370, 5),
emp3 = new Employee(920, "Jan Jones", 47834.25, 1);
System.out.println(emp1.equals(emp3));
ArrayList<Employee> list = new ArrayList<>();
list.add(emp1);
list.add(emp2);
list.add(emp3);
//sort call goes here...
for (Employee e : list)
System.out.println(e);

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

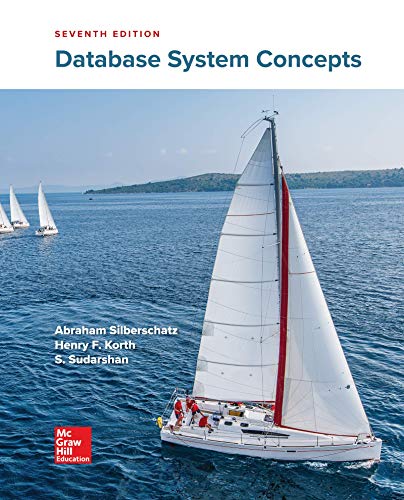
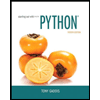
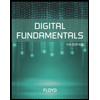
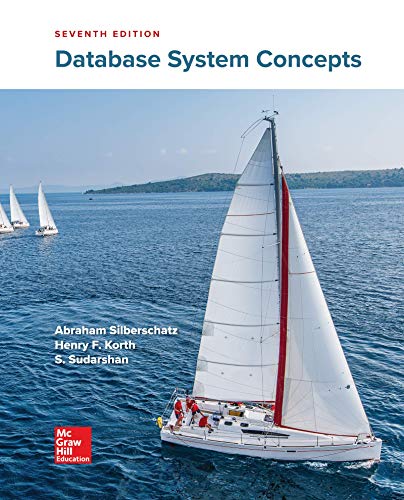
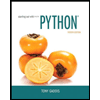
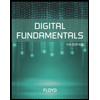
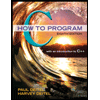
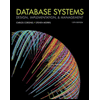
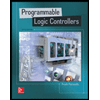