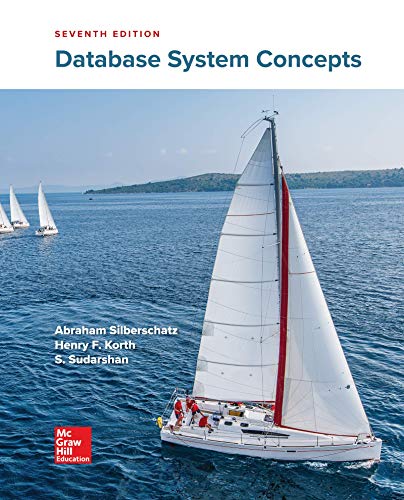
How Do I code in java?
Animal
- name : String
- birthYear : int
- weight : double
- gender : char
+ Animal() // Set name="", birthYear=1900, weight=0.0, gender='u'
+ Animal(String, int, double, char)
+ getName() : String
+ setName(String) : void
+ getBirthYear() : int
+ setBirthYear(int) : void
+ getWeight() : double
+ setWeight(double) : void //if input value is negative, set weight to -1
+ getGender() : char
+ setGender(char) : void // if input value is not 'm' or 'f', set gender to 'u' (unknown)
+ calculateAge(int) : int // use birthYear and the argument of currentYear to calculate age.
// If currentYear < birthYear, return -1.
+ isMale() : boolean // return true if gender is 'm'
+ isFemale() : boolean // return true if gender is 'f'
+ printDetails() : void // prints Animal attributes in the following format:
// "Name: %20s | Year of Birth: %4d | Weight: %10.2f | Gender: %c\n"
+ gainWeight() : void //increase weight by 1.
+ gainWeight(double): void // increase weight by the input amount. Weight cannot drop below zero.
+ loseWeight() : void // decrease by 1. Weight cannot drop below zero.
+ loseWeight(double) : void // decrease weight by the input amount. Weight cannot drop below zero.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- For your homework assignment, build a simple application for use by a local retail store. Your program should have the following classes: Item: Represents an item a customer might buy at the store. It should have two attributes: a String for the item description and a float for the price. This class should also override the __str__ method in a nicely formatted way. Customer: Represents a customer at the store. It should have three attributes: a String for the customer's name, a Boolean for the customer's preferred status, and a list of 5 indexes to hold Item objects. Include the following methods: make_purchase: accepts a String and a double as parameters to represent the name and price of an item this customer is purchasing. Create a new Item object with this info and append it to the internal list. If the customer is a preferred customer based on the Boolean attribute's value, take 10% off the total sale price. __str__: Override this method to print the customer's name and every…arrow_forwardCharge Account ValidationCreate a class with a method that accepts a charge account number as its argument. The method should determine whether the number is valid by comparing it to the following list of valid charge account numbers:5658845 4520125 7895122 8777541 8451277 13028508080152 4562555 5552012 5050552 7825877 12502551005231 6545231 3852085 7576651 7881200 4581002These numbers should be stored in an array. Use a sequential search to locate the number passed as an argument. If the number is in the array, the method should return true, indicating the number is valid. If the number is not in the array, the method should return false, indicating the number is invalid.Write a program that tests the class by asking the user to enter a charge account number. The program should display a message indicating whether the number is valid or invalid.arrow_forwardFlight assignment runs but there is no imput values for the user to interact when running the program. Kindly fix this issue.import java.util.*; class Flight { String airlineCode; int flightNumber; char type; // D for domestic, I for international String departureAirportCode; String departureGate; char departureDay; int departureTime; String arrivalAirportCode; String arrivalGate; char arrivalDay; int arrivalTime; char status; // S for scheduled, A for arrived, D for departed public Flight(String airlineCode, int flightNumber, char type, String departureAirportCode, String departureGate, char departureDay, int departureTime, String arrivalAirportCode, String arrivalGate, char arrivalDay, int arrivalTime) { this.airlineCode = airlineCode; this.flightNumber = flightNumber; this.type = type; this.departureAirportCode = departureAirportCode; this.departureGate = departureGate;…arrow_forward
- tranlate source program in the picture into quadruples tranlate source program in the picture into quadruples tranlate source program in the picture into quadruplesarrow_forwardFor beginning Java, need help with this: " Purpose: Define class using OO approach Understand how to create and manipulate list of objects Design and create a well-structure program using basic programming constructs and classes Description: Write a program to help you to manage courses you are taking this semester. Your program provides the following options: List all courses Add a course Search word: show all the courses that has the word in the course title (ask the user for the word) List >= input-unit: show all the courses that has unit >= input-unit (ask the user for input) Exit Explanation of options: The program prints your course list. Initially, it should say “Your list is empty” The program asks the user input for a course including: course ID, number of units and title. The program asks the user to input the search word. Then, display all courses that has that word in the title. The search must be case-insensitive. For example, “computer” and…arrow_forwardPlease solve this java problem and including the GUI to run it.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
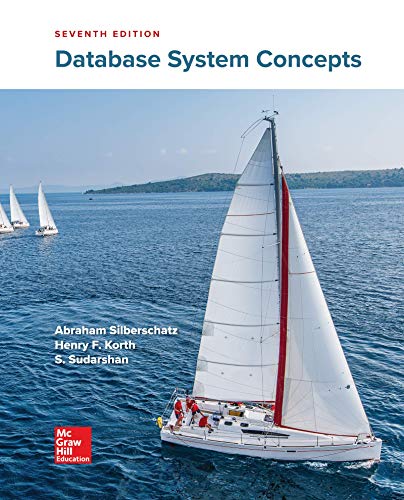
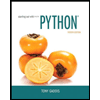
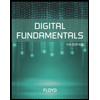
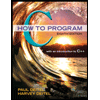
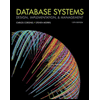
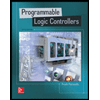