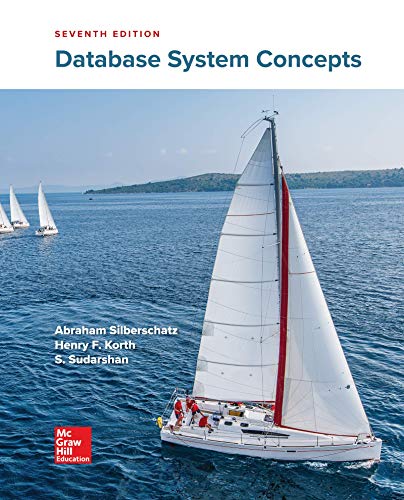
In Java
Write a Fraction class that implements these methods:
• add ─ This method receives a Fraction parameter and adds the parameter fraction to the
calling object fraction.
• multiply ─ This method receives a Fraction parameter and multiplies the parameter
fraction by the calling object fraction.
• print ─ This method prints the fraction using fraction notation (1/4, 21/14, etc.)
• printAsDouble ─ This method prints the fraction as a double (0.25, 1.5, etc.)
• Separate accessor methods for each instance variable (numerator , denominator ) in the
Fraction class
Provide a driver class (FractionDemo) that demonstrates this Fraction class. The driver class should
contain this main method :
public static void main(String[] args)
{
Scanner stdIn = new Scanner(System.in);
Fraction c, d, x; // Fraction objects
System.out.println("Enter numerator; then denominator.");
c = new Fraction(stdIn.nextInt(), stdIn.nextInt());
c.print();
System.out.println("Enter numerator; then denominator.");
d = new Fraction(stdIn.nextInt(), stdIn.nextInt());
d.print();
x = new Fraction(); // create a fraction for number 0
System.out.println("Sum:");
x.add(c).add(d);
x.print();
x.printAsDouble();
x = new Fraction(1, 1); // create a fraction for number 1
System.out.println("Product:");
x.multiply(c).multiply(d);
x.print();
x.printAsDouble();
System.out.println("Enter numerator; then denominator.");
x = new Fraction(stdIn.nextInt(), stdIn.nextInt());
x.printAsDouble();
} // end main
Please study this driver carefully. Note that this driver does not call the accessor methods--that’s OK.
Accessor methods are often implemented regardless of whether there is an immediate need; they are
handy methods in general, and providing them means that future code can use them when needed.
Sample session:
Enter numerator; then denominator.
5
8
5/8
Enter numerator; then denominator.
4
10
4/10
Sum:
82/80
1.025
Product:
20/80
0.25
Enter numerator; then denominator.
6
0
infinity

Step by stepSolved in 4 steps

- IN JAVA "New Employee Gross Salary Calculator" Write a method that accepts an employee's yearly salary and start date and calculates their gross salary from the provided start date up through the end of the year Employees always start on the first of the month Throw an IllegalArgumentException if the provided start date is not the first of the month Employees qualify for a 3% pay raise 3 months after their start date and another 6% 3 months after that Use appropriate data typearrow_forwardAg 1- Random Prime Generator Add a new method to the Primes class called genRandPrime. It should take as input two int values: 1owerBound and upperBound. It should return a random prime number in between TowerBound (inclusive) and upperBound (exclusive) values. Test the functionality inside the main method. Implementation steps: a) Start by adding the method header. Remember to start with public static keywords, then the return type, method name, formal parameter list in parenthesis, and open brace. The return type will be int. We will have two formal parameters, so separate those by a comma. b) Now, add the method body code that generates a random prime number in the specified range. The simplest way to do this is to just keep trying different random numbers in the range, until we get one that is a prime. So, generate a random int using: int randNum = lowerBound + gen.nextInt(upperBound); Then enter put a while loop that will keep going while randNum is not a prime number - you can…arrow_forwardComplete the convert() method that casts the parameter from a double to an integer and returns the result.Note that the main() method prints out the returned value of the convert() method. Ex: If the double value is 19.9, then the output is: 19 Ex: If the double value is 3.1, then the output is: 3 code: public class LabProgram { public static int convert(double d){ /* Type your code here */ } public static void main(String[] args) { System.out.println(convert(19.9)); System.out.println(convert(3.1)); }}arrow_forward
- JAVA PROGRAM Chapter 7. PC #1. Rainfall Class Write a RainFall class that stores the total rainfall for each of 12 months into an array of doubles. The program should have methods that return the following: • the total rainfall for the year • the average monthly rainfall • the month with the most rain • the month with the least rain Demonstrate the class in a complete program. Main class name: RainFall (no package name) est Case 1 Enter the rainfall amount for month 1:\n1.2ENTEREnter the rainfall amount for month 2:\n2.3ENTEREnter the rainfall amount for month 3:\n3.4ENTEREnter the rainfall amount for month 4:\n5.1ENTEREnter the rainfall amount for month 5:\n1.7ENTEREnter the rainfall amount for month 6:\n6.5ENTEREnter the rainfall amount for month 7:\n2.5ENTEREnter the rainfall amount for month 8:\n3.3ENTEREnter the rainfall amount for month 9:\n1.1ENTEREnter the rainfall amount for month 10:\n5.5ENTEREnter the rainfall amount for month 11:\n6.6ENTEREnter…arrow_forwardA method to find a set of value of instance variable Choose one Accessor method Reference method Getter method Mutator methodarrow_forwardChoose the correct answer: You can pass in multiple parameters with different data types into a method. You can pass in only parameters with the same data type into a method. You can receive multiple return values from a method. You can receive a return value with multiple data types from a method.arrow_forward
- In Java Write a Fraction class that implements these methods:• add ─ This method receives a Fraction parameter and adds the parameter fraction to thecalling object fraction.• multiply ─ This method receives a Fraction parameter and multiplies the parameterfraction by the calling object fraction.• print ─ This method prints the fraction using fraction notation (1/4, 21/14, etc.)• printAsDouble ─ This method prints the fraction as a double (0.25, 1.5, etc.)• Separate accessor methods for each instance variable (numerator , denominator ) in theFraction classProvide a driver class (FractionDemo) that demonstrates this Fraction class. The driver class shouldcontain this main method : public static void main(String[] args) { Scanner stdIn = new Scanner(System.in); Fraction c, d, x; // Fraction objects System.out.println("Enter numerator; then denominator."); c = new Fraction(stdIn.nextInt(), stdIn.nextInt()); c.print(); System.out.println("Enter numerator; then denominator."); d = new…arrow_forwardFor your homework assignment, build a simple application for use by a local retail store. Your program should have the following classes: Item: Represents an item a customer might buy at the store. It should have two attributes: a String for the item description and a float for the price. This class should also override the __str__ method in a nicely formatted way. Customer: Represents a customer at the store. It should have three attributes: a String for the customer's name, a Boolean for the customer's preferred status, and a list of 5 indexes to hold Item objects. Include the following methods: make_purchase: accepts a String and a double as parameters to represent the name and price of an item this customer is purchasing. Create a new Item object with this info and append it to the internal list. If the customer is a preferred customer based on the Boolean attribute's value, take 10% off the total sale price. __str__: Override this method to print the customer's name and every…arrow_forwardInteger userValue is read from input. Assume userValue is greater than 1000 and less than 99999. Assign tensDigit with userValue's tens place value. Ex: If the input is 15876, then the output is: The value in the tens place is: 7 2 3 public class ValueFinder { 4 5 6 7 8 9 10 11 12 13 GHE 14 15 16} public static void main(String[] args) { new Scanner(System.in); } Scanner scnr int userValue; int tensDigit; int tempVal; userValue = scnr.nextInt(); Your code goes here */ 11 System.out.println("The value in the tens place is: + tensDigit);arrow_forward
- Design a class named CustomerRecord that holds a customer number, name, and address. Include separate methods to 'set' the value for each data field using the parameter being passed. Include separate methods to 'get' the value for each data field, i.e. "return" the field's value. Pseudocode:arrow_forwardjava programming: pleas see attachment as well***** In this task, you are asked to write a program called Swap.java, including at least 3 following methods: - Main() swapDigitPairs ( parameter) - swapLetterPairs (parameter) In the main method, - Use your Panther Number as the input argument, call swapDigitPairs ( parameter) - Ask user to input a number and call a method swapDigitPairs to swap the number as shown in the example of the following figure. - Ask the user to input a string of letters/numbers and call a method swapLetterPairs to swap the letters as shown in the following example. - swapDigitPairs ( parameter) - swapLetterPairs (parameter) In the main method, - Use your Panther Number as the input argument, call swapDigitPairs ( parameter) - Ask user to input a number and call a method swapDigitPairs to swap the number as shown in the example of the following figure. - Ask the user to input a string of letters/numbers and call a method swapLetterPairs to swap the letters…arrow_forwardWhat is the purpose of a constructor? It creates an array of data values associated with an object. It prints all of the information about our object. It returns all data members from an object back to the main method. It allows us to set our own default values when we create an object. in javaarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
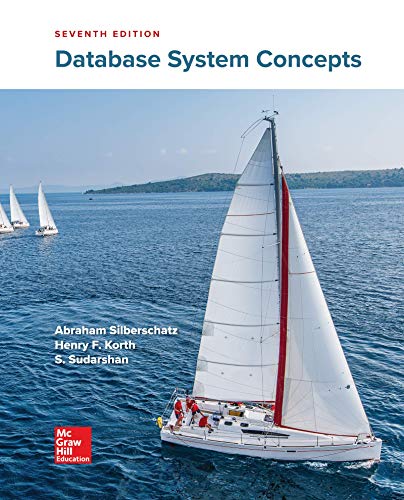
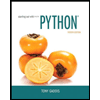
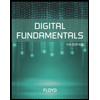
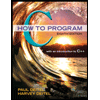
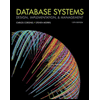
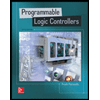