For beginning Java, need help with this: " Purpose: Define class using OO approach Understand how to create and manipulate list of objects Design and create a well-structure program using basic programming constructs and classes Description: Write a program to help you to manage courses you are taking this semester. Your program provides the following options: List all courses Add a course Search word: show all the courses that has the word in the course title (ask the user for the word) List >= input-unit: show all the courses that has unit >= input-unit (ask the user for input) Exit Explanation of options: The program prints your course list. Initially, it should say “Your list is empty” The program asks the user input for a course including: course ID, number of units and title. The program asks the user to input the search word. Then, display all courses that has that word in the title. The search must be case-insensitive. For example, “computer” and “Computer” are considered a match. List courses that have the number of unit >= the user input Terminate the program Requirements: Here is the minimum list of classes that you must have: “java” class - Manages a course (id, number of units, and title) - Keep track of course count in a static variable. You will need this count in other places. “java” program: Implement the main program that perform the following tasks: Declare a course array to keep track of courses. For each course, create one Course object and store it into the course array. You must declare and array of 20, but you may not need to use all elements. It processes all the commands to manage the courses in your list. It should have a method to search for unit, for example: public static void searchCourseUnit( Course[] courseArray, int unitValue) Or public static void searchCourseUnit( Course[] courseArray, int size, int unitValue) It should have another method to search for word in title, for example: public static void searchCourseTitle( Course[] courseArray, String target) Or public static void searchCourseTitle( Course[] courseArray, int size, String target) You must have comments in your class and methods to describe what the class provide and what the methods do. Here’s an example of a method comment: /** Create an array of Circle objects */ public static CircleWithPrivateDataFields[] createCircleArray() You must have the block comment at the beginning of each your source file: /***************************************************************************** * Name: Alexa Amazon * Project 2: Manage a list of courses * Date: Oct. 21, 2021 * Description: This program provides a menu for user to manage … ****************************************************************************/" Here is the sample: " Sample run is attached.
For beginning Java, need help with this:
"
Purpose:
- Define class using OO approach
- Understand how to create and manipulate list of objects
- Design and create a well-structure program using basic
programming constructs and classes
Description:
Write a program to help you to manage courses you are taking this semester.
Your program provides the following options:
- List all courses
- Add a course
- Search word: show all the courses that has the word in the course title (ask the user for the word)
- List >= input-unit: show all the courses that has unit >= input-unit (ask the user for input)
- Exit
Explanation of options:
- The program prints your course list. Initially, it should say “Your list is empty”
- The program asks the user input for a course including: course ID, number of units and title.
- The program asks the user to input the search word. Then, display all courses that has that word in the title. The search must be case-insensitive. For example, “computer” and “Computer” are considered a match.
- List courses that have the number of unit >= the user input
- Terminate the program
Requirements:
- Here is the minimum list of classes that you must have:
- “java” class
- Manages a course (id, number of units, and title)
- Keep track of course count in a static variable. You will need this count in other places.
- “java” program: Implement the main program that perform the following tasks:
- Declare a course array to keep track of courses. For each course, create one Course object and store it into the course array. You must declare and array of 20, but you may not need to use all elements.
- It processes all the commands to manage the courses in your list.
- It should have a method to search for unit, for example:
public static void searchCourseUnit(
Course[] courseArray, int unitValue)
Or
public static void searchCourseUnit(
Course[] courseArray, int size, int unitValue)
- It should have another method to search for word in title, for example:
public static void searchCourseTitle(
Course[] courseArray, String target)
Or
public static void searchCourseTitle(
Course[] courseArray, int size, String target)
- You must have comments in your class and methods to describe what the class provide and what the methods do. Here’s an example of a method comment:
/** Create an array of Circle objects */
public static CircleWithPrivateDataFields[] createCircleArray()
- You must have the block comment at the beginning of each your source file:
/*****************************************************************************
* Name: Alexa Amazon
* Project 2: Manage a list of courses
* Date: Oct. 21, 2021
* Description: This program provides a menu for user to manage …
****************************************************************************/"
Here is the sample:
"
Sample run is attached.



Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

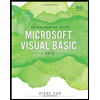
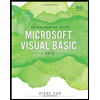