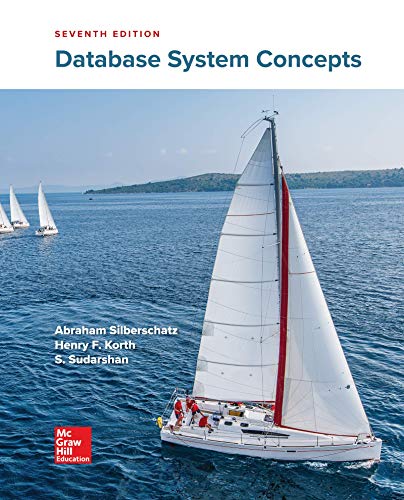
Concept explainers
Here I have a code that displays and gives solution so how can I amke it so I am righting my answer but if its wrong it says incorrect and if I am right it says correct will still showing the solution? import java.util.Scanner; public class Sudoku { public boolean isSafe (int[][] b, int r, int c, int num) { // Check if 'num' is not present in the current row and column for (int i = 0; i < 9; i++) { if (b[r][i] = num || b[i][ c] == num) { return false; } } // Check if 'num' is not present in the 3x3 grid int startRow = r (r% 3); int startCol = c (c% 3); for (int i =0;i<3;i++)\{ for (int j = 0; j < 3; j++) { if (b[i + startRow][j + startCol] == num) { return false; } } } return true; } public boolean solveSudoku (int[][] b) { int n = b.length; // Find an empty location int empty = findEmpty Location(b); int row = empty[0]; int col = empty [1]; // If there is no empty location, the puzzle is solved if (row ==-1~88~col=-1 { return true; } // Try filling the empty location with a number for (int num = 1; num <= 9; num++) { if ( isSafe(b, row, col, num)) { b[row][col] = num; // Recursively try to solve the rest of the puzzle if (solveSudoku(b)) { return true; } // If placing 'num' at the current location doesn't lead to a solution, backtrack b[row][col]=0;\}// If no number can be placed at the current location, backtrack return false; } private int[] findEmptyLocation(int[][] b) { int[] location = new int[]{1, 1); for ( int i = 0; i < 9; i++) { for (int j = 0; j < 9; j++) { if (b[i][j] == 0) { location [0]=i;l location[1] = j; return location; }}} return location; } public void display (int[][] b) { for (int i = 0; i < 9; i++) { for (int j = 0 ;j< 9; j++) { System.out.print(b[i][j]+""); } System.out.println(); } }
public static void main(String[] args) { Scanner scanner = new
Scanner(System.in); // Take user input for the initial Sudoku grid
System.out.println("Enter the Sudoku grid (0 for empty cells):"); int[][]
b = new int[9][9]; for (int~i=0;i<9;i++). for (int~j=0;j<9;j
++) { b[i][j] = scanner.nextInt(); } } Sudoku obj = new Sudoku();
System.out.println("The initial grid is: "); obj.display(b); if (obj.
solveSudoku(b)) { System.out.println("The solution of the grid is: ");
obj.display(b); } else { System.out.println("There is no solution
available."); } scanner.close(); } }

Step by stepSolved in 3 steps with 2 images

- Complete this program to print a table of prices. The first column has width 8 and the second column has width 10. Print the prices with two digits after the decimal point. import java.util.Scanner; public class Table{public static void main(String[] args){Scanner in = new Scanner(System.in);System.out.print("Unit price: ");double price = in.nextDouble(); System.out.println("Quantity Price");int quantity = 1; System.out.printf("%8d%n",quantity, (quantity * price) );quantity = 12;System.out.printf("%8d%n",quantity, (quantity * price));quantity = 100;System.out.printf("%8d%n",quantity, quantity * price);arrow_forwardJava - Insect Growtharrow_forwardCompute the average kids per family. Note that the integers should be type cast to doubles. 427080.2564214.qx3zqy7 1 import java.util.Scanner; N&6 00 2 3 public class TypeCasting { 4 5 7 8 9 10 11 12 13 14 15 16 17 Pun public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int numKidsA; int numKidsB; int numKidsC; int numFamilies; double avgKids; numkidsA scnr.nextInt (); numKidsB = scnr.nextInt (); numkidsC= scnr.nextInt (); numFamilies scnr.nextInt(); * Your solution goes here */ 1 test passed All tests passedarrow_forward
- Implement move the following way. public int[] walk(int... stepCounts) { // Implement the logic for walking the players returnnewint[0]; } public class WalkingBoardWithPlayers extends WalkingBoard{ privatePlayer[] players; privateintround; publicstaticfinalintSCORE_EACH_STEP=13; publicWalkingBoardWithPlayers(int[][] board, intplayerCount) { super(board); initPlayers(playerCount); } publicWalkingBoardWithPlayers(intsize, intplayerCount) { super(size); initPlayers(playerCount); } privatevoidinitPlayers(intplayerCount) { if(playerCount <2){ thrownewIllegalArgumentException("Player count must be at least 2"); } else { this.players=newPlayer[playerCount]; this.players[0] =newMadlyRotatingBuccaneer(); for (inti=1; i < playerCount; i++) { this.players[i] =newPlayer(); } } } package walking.game.player; import walking.game.util.Direction; public class Player{ privateintscore; protectedDirectiondirection=Direction.UP; publicPlayer() {} publicintgetScore() { return score; }…arrow_forwardAssign is Teenager with true if kidAge is 13 to 19 inclusive. Otherwise, assign is Teenager with false. 439894.2564214.qx3zqy7 1 import java.util.Scanner; □NM nor 2 3 public class TeenagerDetector { 4 5 6 7 8 9 10 11 12 13 14 15 16 17 public static void main (String [] args) { Scanner scnr = new Scanner(System.in); boolean isTeenager; int kidAge; kidAge scnr.nextInt(); * Your solution goes here if (isTeenager) { System.out.println("Teen"); } else { System.out.println("Not teen");arrow_forwardIntellij - Java Write a program which does the following: Create a class with the main() method. Take a string array input from the user in main() method. Note: Do not hardcode the string array in code. After the String array input loop, the array should be: words[] = {"today", "is", "a", "lovely", "spring","day", "not", "too", "hot", "not", "too", "cold"}; Print the contents of this array using Arrays.toString() method. Write a method called handleString() that performs the following: Takes a String[] array as method parameter (similar to main method). Loop through the array passed as parameter, and for each word in the array: If the length of the word is even, get the index of the last letter & print that index. Use length() and indexOf() String object methods. If the string length is odd, get the character at the 1st position in the string & print that letter. Use length() and charAt() String object methods.arrow_forward
- import java.util.Arrays; import java.util.Random; public class Board { /** * Construct a puzzle board by beginning with a solved board and then * making a number of random moves. That some of the possible moves * don't actually change what the board looks like. * * @param moves the number of moves to make when generating the board. */ public Board(int moves) { throw new RuntimeException("Not implemented"); } The board is 5 X 5. You can add classes and imports like rand.arrow_forwardUsing the code in the image provided: Implement a method called summation which adds two integers and returns their sum. INPUT: The first line of input contains an integer a. The Second Line of input containes and integer b. OUTPUT: Print the result which is the sum of a and b.arrow_forward*JAVA* complete method Delete the largest valueremoveMax(); Delete the smallest valueremoveMin(); class BinarHeap<T> { int root; static int[] arr; static int size; public BinarHeap() { arr = new int[50]; size = 0; } public void insert(int val) { arr[++size] = val; bubbleUP(size); } public void bubbleUP(int i) { int parent = (i) / 2; while (i > 1 && arr[parent] > arr[i]) { int temp = arr[parent]; arr[parent] = arr[i]; arr[i] = temp; i = parent; } } public int retMin() { return arr[1]; } public void removeMin() { } public void removeMax() { } public void print() { for (int i = 0; i <= size; i++) { System.out.print( arr[i] + " "); } }} public class BinarH { public static void main(String[] args) { BinarHeap Heap1 = new BinarHeap();…arrow_forward
- Please help fastarrow_forwardCorrect my codes in java // Arraysimport java.util.Scanner;public class Assignment1 {public static void main (String args[]){Scanner sc=new Scanner (System.in);System.out.println("Enter mark of student");int n=sc.nextInt();int a[]=new int [n];int i;for(i=0;i<n;i++){System.out.println("Total marks of student in smster");a[i]=sc.nextInt();}int sum=0;for(i=0;i<n;i++){sum=sum+a[i];}System.out.println("Total marks is :");for (i=0;i<n;i++);{System.out.println(a[i]);}System.out.println();}}arrow_forwardimport java.awt.*; public class TestRectangle {public static void main(String[] args) {Rectangle r1 = new Rectangle(5, 4, 10, 17);Rectangle r2 = new Rectangle(10, 10, 20, 3);Rectangle r3 = new Rectangle(0, 1, 12, 15);Rectangle r4 = new Rectangle(10, 10, 20, 3);System.out.println("r1 = " + r1);System.out.println("r2 = " + r2);System.out.println("r3 = " + r3);System.out.println("r2 equals r1? " + r2.equals(r1));System.out.println("r2 equals r2? " + r2.equals(r2));System.out.println("r2 equals r3? " + r2.equals(r3));System.out.println("r2 equals r4? " + r2.equals(r4)); System.out.println("r1 contains (6, 8)? = " + r1.contains(6, 8));System.out.println("r2 contains (6, 8)? = " + r2.contains(6, 8));System.out.println("r3 contains (6, 8)? = " + r3.contains(6, 8));System.out.println("r2 contains (30, 13)? = " + r2.contains(30, 13)); r1.intersect(r3);r2.intersect(r4);System.out.println("r1 intersect r3 = " + r1);System.out.println("r2 intersect r4 = " + r2);…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
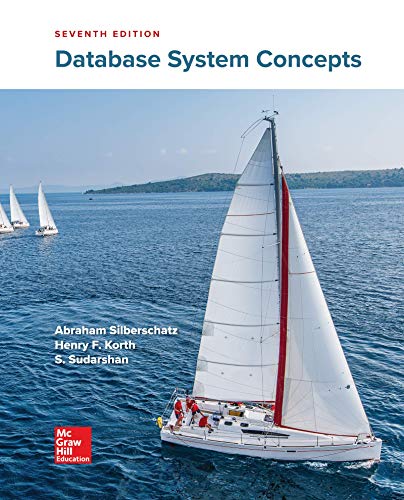
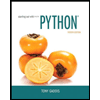
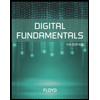
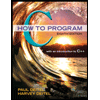
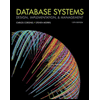
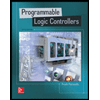