he following code isn't working correctly and I am unable to figure out why. The for loop is never reached in the first if statement and the program never terminates. May someone please explain to me why that is.
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
The following code isn't working correctly and I am unable to figure out why. The for loop is never reached in the first if statement and the program never terminates. May someone please explain to me why that is.
#include <stdio.h>
#include <time.h>
#include <stdlib.h>
#include <mpi.h>
int main(void)
{
int sum, comm_sz, my_rank, i, next, x;
int powTwo = 2;
int diff = 1;
MPI_Init(NULL, NULL);
MPI_Comm_size(MPI_COMM_WORLD, &comm_sz);
MPI_Comm_rank(MPI_COMM_WORLD, &my_rank);
srandom((unsigned)time(NULL) + my_rank);
x = random() % 10;
if (my_rank % powTwo == 0)
{
printf("IF----");
printf("Process %d generates: %d\n", my_rank, x);
for (i = 0; i < comm_sz; i++)
{
MPI_Recv(&x, 1, MPI_INT, i, my_rank , MPI_COMM_WORLD, MPI_STATUS_IGNORE);
sum += x;
printf("Current Sum=: %d\n", sum);
}
printf("The new divisor is:%d\n", powTwo);
powTwo *= 2;
diff *= 2;
} else if (my_rank % powTwo != 0) {
printf("ELSE----");
printf("Process %d generates: %d\n", my_rank, x);
MPI_Send(&x, 1, MPI_INT, 0, 0, MPI_COMM_WORLD);
}else if (my_rank==0){
printf("Sum=: %d\n", sum);
}
MPI_Finalize();
return 0;
}


Step by step
Solved in 2 steps

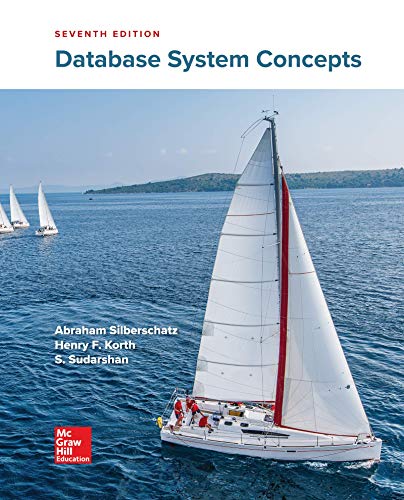
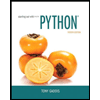
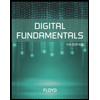
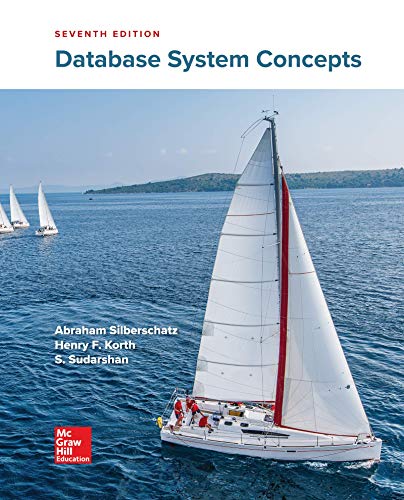
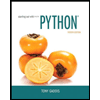
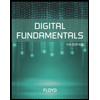
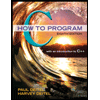
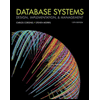
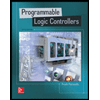