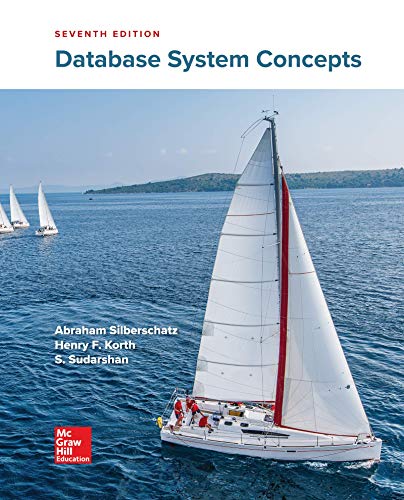
Concept explainers
Given the following definitions that we have used in class to represent a doubly-linked, circular List with a dummy-header node that contains data of type "struct Student".
typedef struct { char *name; char *major; } Student;
typedef struct node { Student *data; struct node *next; struct node *prev; } Node;
typedef struct { Node *header; int size; } LinkedList;
LinkedList *roster;
Here is a picture of what the Linked List might look like, although you should answer the following question for a general list (ie, that does not necessarily contain 3 data items).
What TYPE of data is the following: *roster
Group of answer choices
int
int *
Node
Node *
Student
Student *
char
char *
This is legal code, but it does not match any of the types listed above.
This is illegal code, and would produce a syntax error No hand written solution and no image

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- template <class T> class List; template <class T> class Node{ friend class List<T>; private: T data; Node* link; }; template <class T> class List{ public: List(){first = 0;} void InsertBack(const T& e); void Concatenate(List<T>& b); void Reverse(); class Iterator{ …. }; Iterator Begin(); Iterator End(); private: Node* first; }; I need algorithm , I think it may use iterator to complile. The question shows on below photo.arrow_forwardCould you help me the following question? The Node structure is defined as follows (we will use integers for data elements): public class Node { public int data; public Node next; public Node previous; } The Double Ended Doubly Linked List (DEDLL) class definition is as follows: public class DEDLL { public int currentSize; public Node head; public Node tail; } This design is a modification of the standard linked list. In the standard that we discussed in class, there is only a head node that represents the first node in the list and each node only contains a reference to the next node in the list until the chain of nodes reach null. In this DEDLL, each node also has a reference to the previous node. The DEDLL also contains a reference to the tail of the list that represents the last node in the list. For example, a DEDLL with 4 elements may have nodes with values 5, 2, 6, and 8 from head to tail. This…arrow_forwardwrite in c++ Define the 3 bolded functions for the Queue (circular array): class Queue { private: double array[10000]; int front, rear, numItems; public: Queue() {front = numItems = 0; rear = -1;} bool isEmpty(); bool isFull(); void enqueue(double d); int dequeue(); void dequeueMany(int n); //remove n values from the front void enqueueMany(double values[], int n); //add n values from the array}; Hints: void dequeueMany(int n) (hint 6 lines of code, no loops, if there are more than n elements in the queue, use math to re-compute front. Otherwise, make the queue empty. Don’t forget to update numItems. void enqueueMany(int values[], int n) (hint 3 lines of code, use a for loop, call another function, but make sure there’s enough room in the array first!! )arrow_forward
- Assume you have a class SLNode representing a node in a singly-linked list and a variable called list referencing the first element on a list of integers, as shown below: public class SLNode { private E data; private SLNode next; public SLNode( E e){ data = e; next = null; } public SLNode getNext() { return next; } public void setNext( SLNoden){ next = n; } } SLNode list; Write a fragment of Java code that would append a new node with data value 21 at the end of the list. Assume that you don't know if the list has any elements in it or not (i.e., it may be empty). Do not write a complete method, but just show a necessary fragment of code.arrow_forwardQuestions: There needs to be a dynamic array of Child that opposes with SCALE and is assigned to "familyTree". The createFamilyTree() function needs to be defined. Please see the C++ code.arrow_forwardComputer science helparrow_forward
- Given an IntNode struct and the operating functions for a linked list, complete the following functions to extend the functionality of the linked list(this is not graded).arrow_forwardIf you need to announce a Linked List Node structure for recording two data member with Name, Save Money, and a link pointer for next node in C language. The logical view is shown as attached figure. The requirement of data member record is following: Name is spelled by Character with maximal length 16; Save_Money is a positive integer number. Please filled the announcement statements for the Node of Linked List. struct Node { } node_Member; Save_Money; *next;arrow_forwardComputer science JAVA programming question i need help with this entire question pleasearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
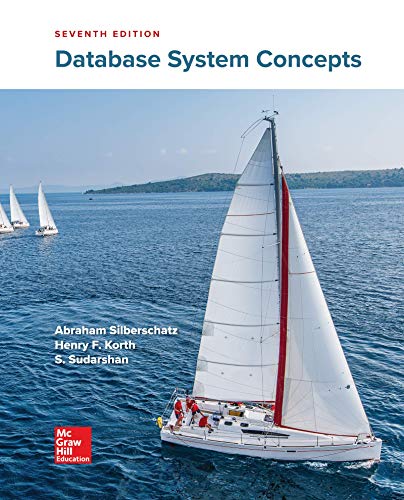
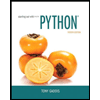
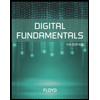
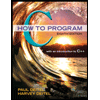
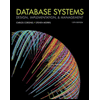
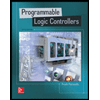