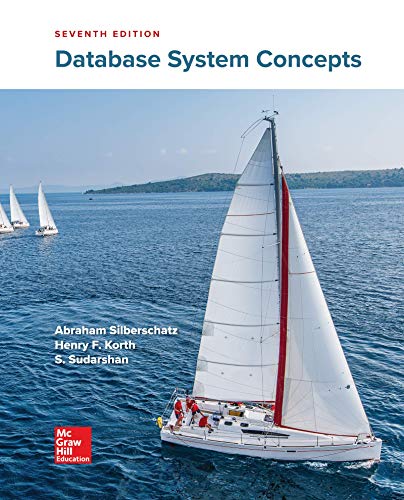
JAVA
Given class Triangle , complete main() to read and set the base and height of triangle1 and of triangle2, determine which triangle's area is smaller, and output that triangle's info, making use of Triangle's relevant methods.
/****************************************************************
import java.util.Scanner;
class TriangleArea {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
**************************************************************************************/
finish code in Java.

The below program uses class Triangle to compare the area between two triangles and outputs which triangle has the smaller area. The user is prompted to input the base and height for both triangles. Objects of type Triangle are then created, and the areas of both triangles are calculated. An if-else statement is used to compare the two areas and output the smaller triangle's info.
Step by stepSolved in 4 steps with 2 images

- Java. Code: public class Person{// PLEASE START YOUR CODE HERE// *********************************************************// *********************************************************// PLEASE END YOUR CODE HERE//constructorpublic Person(String firstName, String lastName){this.firstName = firstName;this.lastName = lastName;}public String toString(){return firstName + " " + lastName;}}arrow_forwardFor the code in java below it shows a deck of 52 cards and asks the name of the two human players and makes both players draw five cards from the deck. What I want to added into the code is for Player A to manually pick a card from his/her 5 cards and have Player B pick two cards that equal the value of Player A's card if possible. Main class code and Player class code shown below: Main class code: import java.util.ArrayList; import java.util.Scanner; import java.util.List; import java.util.Random; class Main { publicstaticvoid main(String[] args) { // card game, two players, take turns. String[] suits = {"Hearts", "Clubs", "Spades", "Diamonds"}; String[] numbers = {"A", "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K"}; for(String oneSuit : suits){ for(String num : numbers){ System.out.println(oneSuit + " " + num); } } List<Player> listOfPlayers = new ArrayList<>(); Scanner sc = new Scanner(System.in); System.out.println("Name of Player 1");…arrow_forwardUse Java.arrow_forward
- code in java Download the file boxer.java write the code for determining the boxer’s weight class as described in the comments. Compile your code and remove any errors, submit the file named boxer java Please use the most simplest and basic methodarrow_forwardOOP JAVAarrow_forwardUse java See the image for requirementsarrow_forward
- //Todo write test cases for SimpleCalculator Class // No need to implement the actual Calculator class just write Test cases as per TDD. // you need to just write test cases no mocking // test should cover all methods from calculator and all scenarios, so a minimum of 5 test // 1 for add, 1 for subtract, 1 for multiply, 2 for divide (1 for normal division, 1 for division by 0) // make sure all these test cases fail public class CalculatorTest { //Declare variable here private Calculator calculator; //Add before each here //write test cases here }arrow_forwardCreate another class TestCarwhich creates array of n number of cars, uses appropriate set methods to set the values of parametersof every car. Display the details of each car. (If max speed is between 120 and 150 then category is“High speed” otherwise “Normal”) (JAVA)arrow_forwardFibonacci A fibonacci sequence is a series of numbers in which each number is the sum of the two preceding numbers. For example: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, and so on… For this, you will implement a class that takes a positive integer (n) and returns the number in the nth position in the sequence. Examples When n is 1, the returned value will be 0. When n is 4, the returned value will be 2. When n is 9, the returned value will be 21. come up with the formula and base cases Implementation Create a class Fibonacci with a public static method getValue. Create a Main class to test and run your Fibonacci class.arrow_forward
- Java Program for a Secret Santa gift exchange - NO GUI ** Please include ULM and comments in code. ** Minimum requirements are: At least 1 loop An Array or ArrayList At least 3 Java classes Use methods I am trying to make it so that the program will: Prompt to enter a participant's name (first and last) Prompt to enter their address (including city, state, and zip) Prompt to identify gender Prompt to see if the participant is a child, teen, or adult. Make sure there are an even amount of participants, if not it errors. If even amount of participants then, match each participant with another (identifying each as "X buys give for X") and print out the information.arrow_forwardCreate a class named RecusiveMethod having a recursive method. The recursive method accepts an integer argument and displays all even numbers from 1 up to the number passed as an argument. For example, if 25 is passed as an argument, the method will display 2, 4, 6, 8, 10, 12, 14, ……24. Demonstrate the method.arrow_forwardcode in java Download the file boxer.java write the code for determining the boxer’s weight class as described in the comments. Compile your code and remove any errors, submit the file named boxer javaarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
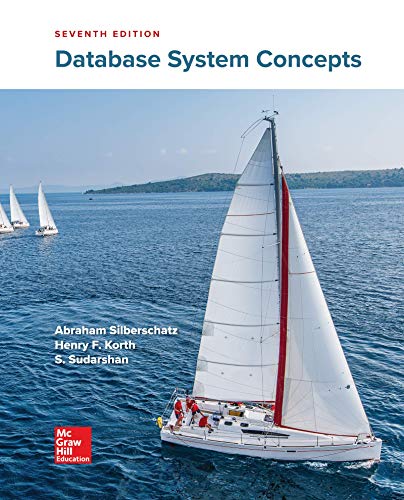
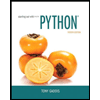
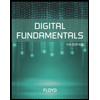
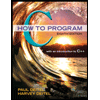
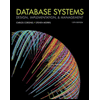
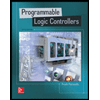