JAVA A Matryoshka, or Russian nesting doll is either solid or hollow. If it is hollow, it contains another doll (which can also be hollow or solid). Given the supplied Doll class, implement a recursive method named show that returns a String representing the chain for dolls, for example Natasha contains Tanya contains Peter public class Doll { private String name; private Doll dollInside; /** * Constructs a hollow doll with the doll it contains. */ public Doll(String theName, Doll theDoll) { name = theName; dollInside = theDoll; } /** * Constucts a solid doll. */ public Doll(String theName) { name = theName; dollInside = null; } public boolean isSolid() { return dollInside == null; } public String getName() { return name; } public Doll getDollInside() { return dollInside; } } public class DollViewer { public static String show(Doll d) { /* code goes here */ } public static void main (String [] args) { Doll dolls1 = new Doll("Alina", new Doll("Vera", new Doll("Galina"))); Doll dolls2 = new Doll("Anna", new Doll("Dina")); Doll dolls3 = new Doll("Nika"); Doll dolls4 = new Doll("Martina", new Doll("Olga", new Doll("Sofia", new Doll("Elvira")))); System.out.println(show(dolls1)); System.out.println(show(dolls2)); System.out.println(show(dolls3)); System.out.println(show(dolls4)); } }
JAVA
A Matryoshka, or Russian nesting doll is either solid or hollow. If it is hollow, it contains another doll (which can also be hollow or solid). Given the supplied Doll class, implement a recursive method named show that returns a String representing the chain for dolls, for example
Natasha contains Tanya contains Peter
public class Doll
{
private String name;
private Doll dollInside;
/**
* Constructs a hollow doll with the doll it contains.
*/
public Doll(String theName, Doll theDoll)
{
name = theName;
dollInside = theDoll;
}
/**
* Constucts a solid doll.
*/
public Doll(String theName) { name = theName; dollInside = null; }
public boolean isSolid() { return dollInside == null; }
public String getName() { return name; }
public Doll getDollInside() { return dollInside; }
}
public class DollViewer
{
public static String show(Doll d)
{
/* code goes here */
}
public static void main (String [] args)
{
Doll dolls1 = new Doll("Alina", new Doll("Vera", new Doll("Galina")));
Doll dolls2 = new Doll("Anna", new Doll("Dina"));
Doll dolls3 = new Doll("Nika");
Doll dolls4 = new Doll("Martina", new Doll("Olga", new Doll("Sofia", new Doll("Elvira"))));
System.out.println(show(dolls1));
System.out.println(show(dolls2));
System.out.println(show(dolls3));
System.out.println(show(dolls4));
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

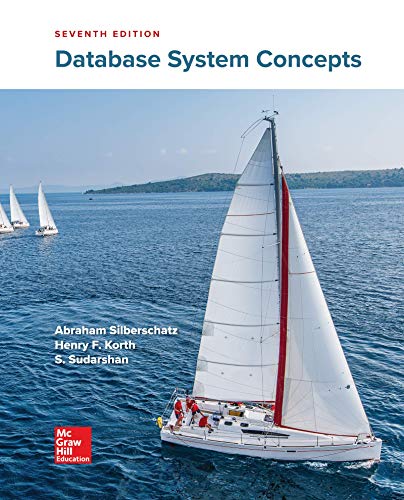
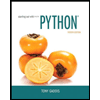
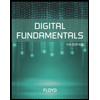
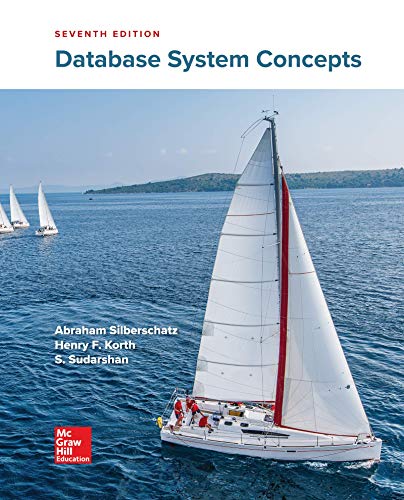
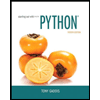
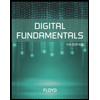
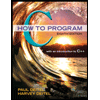
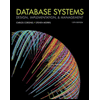
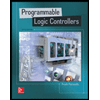