function removeErrMsgs() { var errMessages = document.getElementsByClassName('msg'); for (let msg of errMessages) { msg.innerHTML = ""; } } function validateValues() { var toBeReturned = true; removeErrMsgs(); var fname = document.getElementById("fname").value; if (fname.length > 30) { document.getElementsByClassName('msg')[0].innerHTML = "First name can not be longer than 30 characters"; toBeReturned = false; } var lname = document.getElementById("lname").value; if (lname.length > 30) { document.getElementsByClassName('msg')[1].innerHTML = "Last name can not be longer than 30 characters"; toBeReturned = false; } var phone = document.getElementById('num').value; if (phone.length > 8) { document.getElementsByClassName('msg')[2].innerHTML = "Phone number can not be greater than 8 numbers"; toBeReturned = false; } var items = document.getElementById('items').value; if (items > 4 || items < 2) { document.getElementsByClassName('msg')[3].innerHTML = "Item numbers should be between 1 and 5"; toBeReturned = false; } var regex = /^[A-Za-z0-9 ]+$/; var regex2 = /^[A-Za-z ]+$/ ; var testFirstName = regex2.test(fname); if (!testFirstName) { document.getElementsByClassName('msg')[0].innerHTML = "First name can not be empty and can not have numeric and special characters"; toBeReturned = false; } var testLastName = regex2.test(lname); if (!testLastName) { document.getElementsByClassName('msg')[1].innerHTML = "Last name can not be empty and can not have numeric and special characters"; toBeReturned = false; } var testPhone = regex.test(phone); if (!testPhone) { document.getElementsByClassName('msg')[2].innerHTML = "Phone number can not be empty and can not have special characters"; toBeReturned = false; } var testItemNum = regex.test(items); if (!testItemNum) { document.getElementsByClassName('msg')[3].innerHTML = "Item number can not be empty and can not have special characters"; toBeReturned = false; } return toBeReturned; } **explaine the code of javascript step by step
function removeErrMsgs() {
var errMessages = document.getElementsByClassName('msg');
for (let msg of errMessages) {
msg.innerHTML = "";
}
}
function validateValues() {
var toBeReturned = true;
removeErrMsgs();
var fname = document.getElementById("fname").value;
if (fname.length > 30) {
document.getElementsByClassName('msg')[0].innerHTML = "First name can not be longer than 30 characters";
toBeReturned = false;
}
var lname = document.getElementById("lname").value;
if (lname.length > 30) {
document.getElementsByClassName('msg')[1].innerHTML = "Last name can not be longer than 30 characters";
toBeReturned = false;
}
var phone = document.getElementById('num').value;
if (phone.length > 8) {
document.getElementsByClassName('msg')[2].innerHTML = "Phone number can not be greater than 8 numbers";
toBeReturned = false;
}
var items = document.getElementById('items').value;
if (items > 4 || items < 2) {
document.getElementsByClassName('msg')[3].innerHTML = "Item numbers should be between 1 and 5";
toBeReturned = false;
}
var regex = /^[A-Za-z0-9 ]+$/;
var regex2 = /^[A-Za-z ]+$/ ;
var testFirstName = regex2.test(fname);
if (!testFirstName) {
document.getElementsByClassName('msg')[0].innerHTML = "First name can not be empty and can not have numeric and special characters";
toBeReturned = false;
}
var testLastName = regex2.test(lname);
if (!testLastName) {
document.getElementsByClassName('msg')[1].innerHTML = "Last name can not be empty and can not have numeric and special characters";
toBeReturned = false;
}
var testPhone = regex.test(phone);
if (!testPhone) {
document.getElementsByClassName('msg')[2].innerHTML = "Phone number can not be empty and can not have special characters";
toBeReturned = false;
}
var testItemNum = regex.test(items);
if (!testItemNum) {
document.getElementsByClassName('msg')[3].innerHTML = "Item number can not be empty and can not have special characters";
toBeReturned = false;
}
return toBeReturned;
}
**explaine the code of javascript step by step

Step by step
Solved in 3 steps

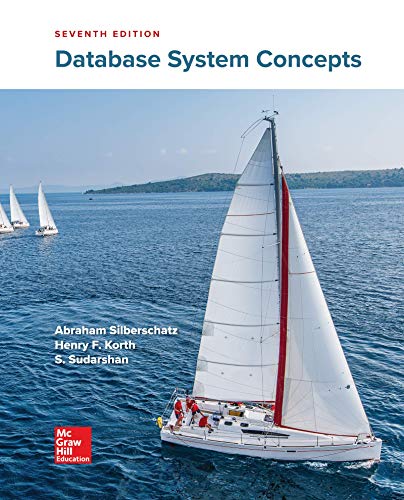
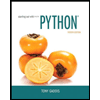
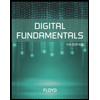
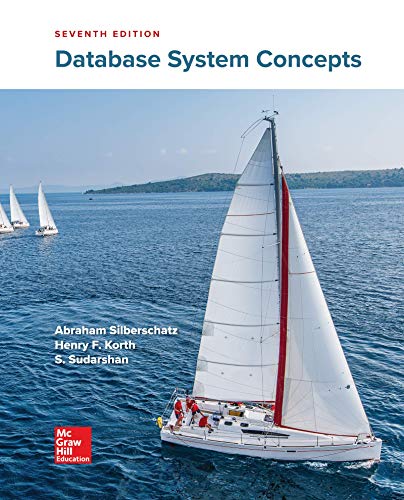
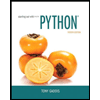
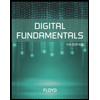
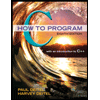
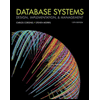
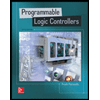