For this milestone, you will be making a more complicated pattern in each cell. You will be splitting the cell into several shapes and individually filling/coloring them in. You can still: decide whether the cells and its patterns are filled or unfilled choose the fill and pen colors using the user inputs Like the previous milestone, you will be asking for the fill colors for each individual shape/section as well. Sample output: Grid size (n): (input) Pen color: (input) Fill color: (input) Rectangle color: (input) Triangle color: (input) Each cell should have this drawing. The specific of this drawing is: A rectangle with a width 1/4 of the cell width and height the same as the cell Two triangles created by a diagonal line starting from the top right corner of the rectangle and ending on the bottom right of the cell You can simplify drawing the triangles by only drawing and filling in one of them, leaving the other as part of the cell background Here is a full grid with the cell drawing: import turtle import random def draw_rectangle(width, height, color): turtle.color(color) turtle.begin_fill() for _ in range(2): turtle.forward(width) turtle.left(90) turtle.forward(height) turtle.left(90) turtle.end_fill() def draw_triangle(side, color): turtle.color(color) turtle.begin_fill() for _ in range(2): turtle.forward(side) turtle.left(135) turtle.end_fill() def draw_cell(cell_size, rect_color, tri_color): rect_width = cell_size / 4 draw_rectangle(rect_width, cell_size, rect_color) turtle.forward(rect_width) draw_triangle(cell_size * (3 ** 0.5) / 2, tri_color) def draw_grid(n, cell_size, pen_color, fill_color, rect_color, tri_color): turtle.color(pen_color, fill_color) for i in range(n): for j in range(n): turtle.up() turtle.goto(-n * cell_size / 2 + j * cell_size, n * cell_size / 2 - i * cell_size) turtle.down() turtle.begin_fill() for _ in range(4): turtle.forward(cell_size) turtle.right(90) turtle.end_fill() draw_cell(cell_size, rect_color, tri_color) def main(): # turtle.tracer(False) n = int(input("Grid size (n): \n")) pen_color = input("Pen color: \n") fill_color = input("Fill color: \n") rect_color = input("Rectangle color: \n") tri_color = input("Triangle color: \n") size_for_canvas = 500 cell_size = size_for_canvas / n s = turtle.Screen() s.setup(width=size_for_canvas + 10, height=size_for_canvas + 10) s.screensize(size_for_canvas, size_for_canvas) draw_grid(n, cell_size, pen_color, fill_color, rect_color, tri_color) turtle.tracer(True) turtle.mainloop() main() it not doing the grid of the shape is all over the place
For this milestone, you will be making a more complicated pattern in each cell. You will be splitting the cell into several shapes and individually filling/coloring them in.
You can still:
- decide whether the cells and its patterns are filled or unfilled
- choose the fill and pen colors using the user inputs
Like the previous milestone, you will be asking for the fill colors for each individual shape/section as well.
Sample output:
Grid size (n): (input) Pen color: (input) Fill color: (input) Rectangle color: (input) Triangle color: (input)
Each cell should have this drawing. The specific of this drawing is:
- A rectangle with a width 1/4 of the cell width and height the same as the cell
- Two triangles created by a diagonal line starting from the top right corner of the rectangle and ending on the bottom right of the cell
You can simplify drawing the triangles by only drawing and filling in one of them, leaving the other as part of the cell background
Here is a full grid with the cell drawing:
import turtleimport random
def draw_rectangle(width, height, color):turtle.color(color)turtle.begin_fill()for _ in range(2):turtle.forward(width)turtle.left(90)turtle.forward(height)turtle.left(90)turtle.end_fill()
def draw_triangle(side, color):turtle.color(color)turtle.begin_fill()for _ in range(2):turtle.forward(side)turtle.left(135)turtle.end_fill()
def draw_cell(cell_size, rect_color, tri_color):rect_width = cell_size / 4draw_rectangle(rect_width, cell_size, rect_color)
turtle.forward(rect_width)draw_triangle(cell_size * (3 ** 0.5) / 2, tri_color)
def draw_grid(n, cell_size, pen_color, fill_color, rect_color, tri_color):turtle.color(pen_color, fill_color)for i in range(n):for j in range(n):turtle.up()turtle.goto(-n * cell_size / 2 + j * cell_size, n * cell_size / 2 - i * cell_size)turtle.down()turtle.begin_fill()for _ in range(4):turtle.forward(cell_size)turtle.right(90)turtle.end_fill()
draw_cell(cell_size, rect_color, tri_color)
def main():# turtle.tracer(False)
n = int(input("Grid size (n): \n"))pen_color = input("Pen color: \n")fill_color = input("Fill color: \n")rect_color = input("Rectangle color: \n")tri_color = input("Triangle color: \n")
size_for_canvas = 500cell_size = size_for_canvas / n
s = turtle.Screen()s.setup(width=size_for_canvas + 10, height=size_for_canvas + 10)s.screensize(size_for_canvas, size_for_canvas)
draw_grid(n, cell_size, pen_color, fill_color, rect_color, tri_color)
turtle.tracer(True)turtle.mainloop()
main()it not doing the grid of the shape is all over the place

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Tip: You can simplify drawing the triangles by only drawing and filling in one of them, leaving the other as part of the cell background
Here is a full grid with the cell drawing:
Each cell should have this drawing. The specific of this drawing is:
- A rectangle with a width 1/4 of the cell width and height the same as the cell
- Two triangles created by a diagonal line starting from the top right corner of the rectangle and ending on the bottom right of the cell
Here is what the cell should look like if colored, each section should be a different color (if filled):
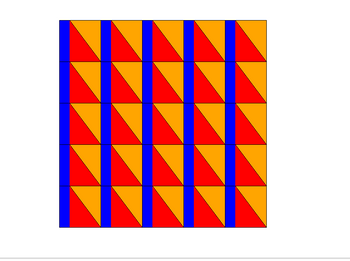
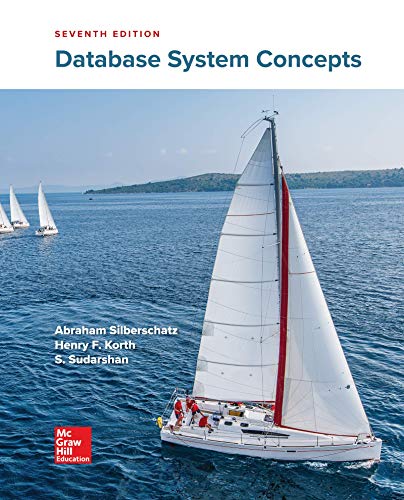
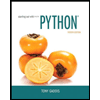
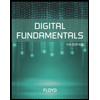
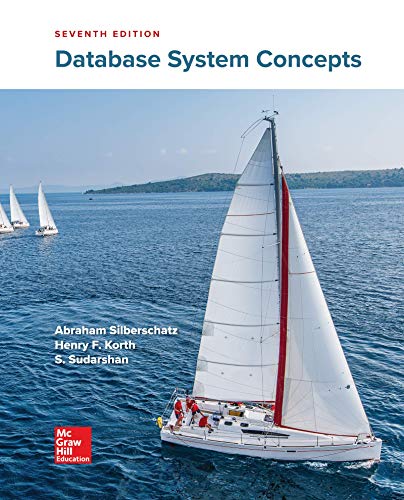
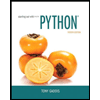
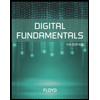
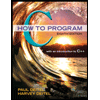
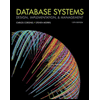
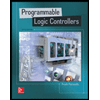