ome up with a nested loop that prints the following table of numbers out. Don’t worry about getting the columns lined up properly (you can use \t after each number to help). 1 2 3 4 5 2 4 6 8 10 3 6 9 12 15 4 8 12 16 20 5 10 15 20 25 This is effectively a multiplication table, where each number is its row number * its column number. To get this, you can take the 1 2 3 4 example pattern from the slides and modify it. Make it 5 rows instead of 4 and adjust the columns to print 1-5 instead of 1-4. Lastly, modify the print statement to print the row number * the column number instead.
Come up with a nested loop that prints the following table of numbers out. Don’t worry about getting the columns lined up properly (you can use \t after each number to help).
1 2 3 4 5
2 4 6 8 10
3 6 9 12 15
4 8 12 16 20
5 10 15 20 25
This is effectively a multiplication table, where each number is its row number * its column number. To get this, you can take the 1 2 3 4 example pattern from the slides and modify it. Make it 5 rows instead of 4 and adjust the columns to print 1-5 instead of 1-4. Lastly, modify the print statement to print the row number * the column number instead.

A nested loop is defined as a loop where one loop resides inside another loop.
The inner loop gets executed first. Once all the conditions within the inner loop are satisfied and it becomes true, then it moves to the search of the outer loop.
This is also as called a “loop within a loop”.
Syntax of Nested for Loop
for (initialization; condition; increment)
{
for (initialization; condition; increment)
{
// set of statements for inner loop
}
//set of statements for outer loop
}
Step by step
Solved in 4 steps with 2 images

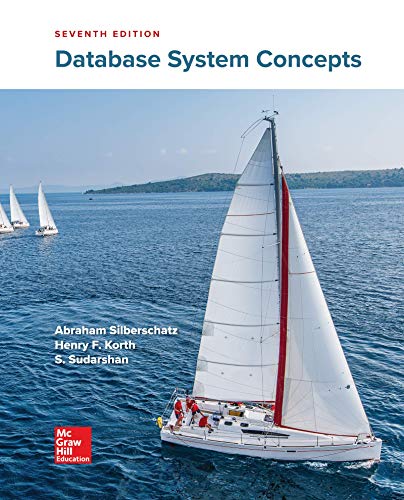
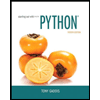
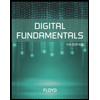
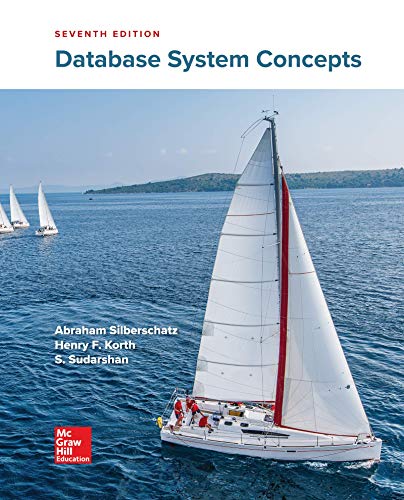
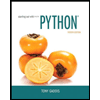
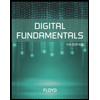
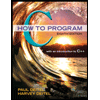
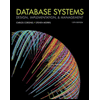
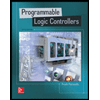