Can you help me with this code becuase I only need help with one of the parts. I have attached my code below and task the needs to answered but you have to use my code and the variables which I have provided in the photo. question that i need help with: the Eight Puzzle consists of a 3 x 3 board of sliding tiles with a single empty space. For each configuration, the only possible moves are to swap the empty tile with one of its neighboring tiles. The goal state for the puzzle consists of tiles 1-3 in the top row, tiles 4-6 in the middle row, and tiles 7 and 8 in the bottom row, with the empty space in the lower-right corner.you will develop two solvers for a generalized version of the Eight Puzzle, in which the board can have any number of rows and columns. A natural representation for this puzzle is a two-dimensional list of integer values between 0 and r · c -1 (inclusive), where r and c are the number of rows and columns in the board, respectively. In this problem, we will adhere to the convention that the 0-tile represents the empty space. here is my code: import random class TilePuzzle(object): def __init__(self, board): self.board = board self.rows = len(board) self.cols = len(board[0]) self.empty_row, self.empty_col = self.find_empty_tile() def get_board(self): return self.board def find_empty_tile(self): for row in range(self.rows): for col in range(self.cols): if self.board[row][col] == 0: return row, col def perform_move(self, direction): row, col = self.empty_row, self.empty_col if direction == 'up': new_row, new_col = row - 1, col elif direction == 'down': new_row, new_col = row + 1, col elif direction == 'left': new_row, new_col = row, col - 1 elif direction == 'right': new_row, new_col = row, col + 1 else: return False # Invalid move direction. if 0 <= new_row < self.rows and 0 <= new_col < self.cols: self.board[row][col], self.board[new_row][new_col] = \ self.board[new_row][new_col], self.board[row][col] self.empty_row, self.empty_col = new_row, new_col return True else: return False def scramble(self, num_moves): directions = ['up', 'down', 'left', 'right'] for _ in range(num_moves): moved = False while not moved: # Ensure the move is successful moved = self.perform_move(random.choice(directions)) def is_solved(self): current = 1 for r in range(self.rows): for c in range(self.cols): if self.board[r][c] != current % (self.rows * self.cols): return False current += 1 return True def copy(self): copied_board = [row[:] for row in self.board] return TilePuzzle(copied_board) def successors(self): directions = ['up', 'down', 'left', 'right'] for direction in directions: successor = self.copy() if successor.perform_move(direction): yield direction, successor def find_solutions_iddfs(self): for depth in range(100): # You can adjust the depth limit as needed solutions = self.iddfs_helper(depth, []) for solution in solutions: yield solution def iddfs_helper(self, limit, moves): if limit == 0: if self.is_solved(): yield moves return for direction, successor in self.successors(): yield from successor.iddfs_helper(limit - 1, moves + [direction]) b = [[4, 1, 2], [0, 5, 3], [7, 8, 6]] p = TilePuzzle(b) print(p.get_board()) # Should print the initial board p.scramble(10) # Scramble the puzzle print(p.get_board()) # Should print the scrambled board solutions = p.find_solutions_iddfs() print(next(solutions)) # Should print a solution sequence
Can you help me with this code becuase I only need help with one of the parts. I have attached my code below and task the needs to answered but you have to use my code and the variables which I have provided in the photo.
question that i need help with:
the Eight Puzzle consists of a 3 x 3 board of sliding tiles with a single empty space. For each configuration, the only possible moves are to swap the empty tile with one of its neighboring tiles. The goal state for the puzzle consists of tiles 1-3 in the top row, tiles 4-6 in the middle row, and tiles 7 and 8 in the bottom row, with the empty space in the lower-right corner.you will develop two solvers for a generalized version of the Eight Puzzle, in which the board can have any number of rows and columns.
A natural representation for this puzzle is a two-dimensional list of integer values between 0 and r · c -1 (inclusive), where r and c are the number of rows and columns in the board, respectively. In this problem, we will adhere to the convention that the 0-tile represents the empty space.
here is my code:
class TilePuzzle(object):
def __init__(self, board):
self.board = board
self.rows = len(board)
self.cols = len(board[0])
self.empty_row, self.empty_col = self.find_empty_tile()
def get_board(self):
return self.board
def find_empty_tile(self):
for row in range(self.rows):
for col in range(self.cols):
if self.board[row][col] == 0:
return row, col
def perform_move(self, direction):
row, col = self.empty_row, self.empty_col
if direction == 'up':
new_row, new_col = row - 1, col
elif direction == 'down':
new_row, new_col = row + 1, col
elif direction == 'left':
new_row, new_col = row, col - 1
elif direction == 'right':
new_row, new_col = row, col + 1
else:
return False # Invalid move direction.
if 0 <= new_row < self.rows and 0 <= new_col < self.cols:
self.board[row][col], self.board[new_row][new_col] = \
self.board[new_row][new_col], self.board[row][col]
self.empty_row, self.empty_col = new_row, new_col
return True
else:
return False
def scramble(self, num_moves):
directions = ['up', 'down', 'left', 'right']
for _ in range(num_moves):
moved = False
while not moved:
# Ensure the move is successful
moved = self.perform_move(random.choice(directions))
def is_solved(self):
current = 1
for r in range(self.rows):
for c in range(self.cols):
if self.board[r][c] != current % (self.rows * self.cols):
return False
current += 1
return True
def copy(self):
copied_board = [row[:] for row in self.board]
return TilePuzzle(copied_board)
def successors(self):
directions = ['up', 'down', 'left', 'right']
for direction in directions:
successor = self.copy()
if successor.perform_move(direction):
yield direction, successor
def find_solutions_iddfs(self):
for depth in range(100): # You can adjust the depth limit as needed
solutions = self.iddfs_helper(depth, [])
for solution in solutions:
yield solution
def iddfs_helper(self, limit, moves):
if limit == 0:
if self.is_solved():
yield moves
return
for direction, successor in self.successors():
yield from successor.iddfs_helper(limit - 1, moves + [direction])
b = [[4, 1, 2], [0, 5, 3], [7, 8, 6]]
p = TilePuzzle(b)
print(p.get_board()) # Should print the initial board
p.scramble(10) # Scramble the puzzle
print(p.get_board()) # Should print the scrambled board
solutions = p.find_solutions_iddfs()
print(next(solutions)) # Should print a solution sequence

![In the TilePuzzle class, write a method find_solution_a_star(self) that returns an optimal solution to
the current board, represented as a list of direction strings. If multiple optimal solutions exist, any of
them may be returned. Your solver should be implemented as an A* search using the Manhattan
distance heuristic, which is reviewed below. You may assume that the board is solvable. During your
search, you should take care not to add positions to the queue that have already been visited. It is
recommended that you use the PriorityQueue class from the Queue module.
Recall that the Manhattan distance between two locations (r_1, c_1) and (r_2, c_2) on a board is
defined to be the sum of the component wise distances: Ir_1 - r_2| + |c_1 - c_21. The Manhattan
distance heuristic for an entire puzzle is then the sum of the Manhattan distances between each tile
and its solved location.
>>> b = [[4,1,2], [0,5,3], [7,8,6]]
>>>p = TilePuzzle(b)
>>> p.find_solution_a_star()
['up', 'right', 'right', 'down', 'down']
>>> b = [[1,2,3], [4,0,5], [6,7,8]]
>>>p = TilePuzzle(b)
>>> p.find_solution_a_star()
['right', 'down', 'left', 'left', 'up', 'right', 'down', 'right', 'up', 'left', 'left', 'dow
n', 'right', 'right']](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5f31059a-d37f-4dd3-a3ca-fdc1fdc311c0%2Faada7421-c415-47ca-9025-828fc473b426%2Fmr5zjvf_processed.jpeg&w=3840&q=75)

Step by step
Solved in 5 steps with 2 images

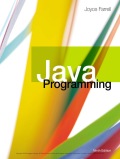
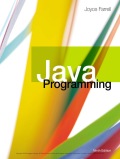