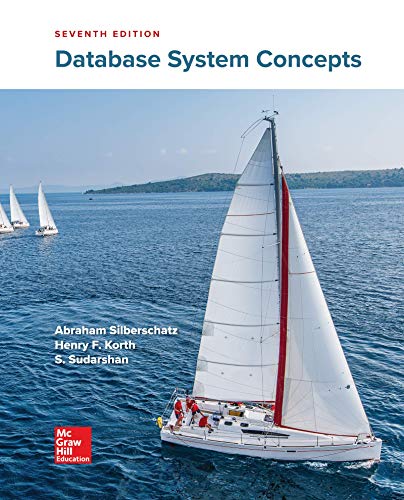
In this exercise you will compare binary search and linear (or sequential) search.
We have included the
What you need to do in this problem is modify each method to instead return the number of times each goes through the loop.
Then you can test out the results on lists of different sizes. We have provided a helper method to generate a list of a certain size.
Be sure to test at least 5 different size arrays!
import java.util.*;
public class CompareSearch
{
public static void main(String[] args)
{
System.out.println("Table of comparison counts");
System.out.println("Length\t\tBinary Search\tLinear Search");
testArrayOfLength(10);
testArrayOfLength(20);
}
// This problem generates an array of length length. Then we select a random
// index of that array and get the element. Then we print out the table row
// entry for how many comparisons it takes on binary search and linear search.
// You'll need to update those methods.
public static void testArrayOfLength(int length)
{
int[] arr = generateArrayOfLength(length);
//System.out.println(Arrays.toString(arr));
int index = (int)(Math.random() * length);
int elem = arr[index];
System.out.println(length + "\t\t" + binarySearch(arr, elem) + "\t\t" + linearSearch(arr, elem));
}
public static int[] generateArrayOfLength(int length)
{
int[] arr = new int[length];
for(int i = 0; i < length; i++)
{
arr[i] = (int)(Math.random() * 100);
}
Arrays.sort(arr);
return arr;
}
// Do a binary search on array to find number. You'll need to modify this
// method to return the number of comparisons done.
public static int binarySearch(int[] array, int number)
{
int low = 0;
int high = array.length - 1;
// Add a counter to count how many times the while loop is executed
while (low <= high)
{
int mid = (low + high) / 2;
if (array[mid] == number)
{
return mid;
}
else if(array[mid] < number)
{
low = mid + 1;
}
else
{
high = mid - 1;
}
}
return -1;
}
// Do a linear search on array to find the index of number. You'll need to modify
// this exercise to return the number of *comparisons* done.
public static int linearSearch(int[] array, int number)
{
// Add a counter to count how many times the for loop is executed
for (int i = 0; i < array.length; i++)
{
if (array[i] == number)
{
return i; // the method returns as soon as the number is found
}
}
return -1; // the code will get here if the number isn't found
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Please provide an explaation and comments and check image for formula . Write approxPI(), a static method using a simple formula to return an approximate value of π. The method has a single int parameter n, the number of terms used to calculate π, and returns a double value. The simple formula (see notation), is expressed as: Step 1: a simple loop (i=0; i<=n) for the summation (Σ), stored to the variable pSum, of the terms: (-1)i / (2i + 1)pSum = 1/1 + -1/3 + 1/5 + -1/7 + 1/9 + -1/11 + 1/13 … (up to, and include, number of terms: n) i=0 i=1 i=2 i=3 i=4 i=5 i=6 … i <= n Step 2: after the summation, multiple by 4 to obtain the final approximation, piApprox = (4 * pSum) Hint: use Math.pow(x,y) (xy) to calculate (-1)iExample calls to the method:displayln ( "n=1: " + approxPI(1) ); // 2.666666666666667displayln ( "n=10: " + approxPI(10) ); // 3.232315809405594displayln ( "n=50: " + approxPI(50) ); // 3.1611986129870506 Test: - test with values of n: 10, 100, 500, 100000arrow_forwardUsing JAVA, write a method that modifies an ArrayList<String>, moving all strings starting with an uppercase letter to the front, without otherwise changing the order of the elements. Write a test program to demonstrate the correct operation of the method.arrow_forwardWe saw that a merge sort performs roughly the same depending on the type of array we have. For this last exercise, we want to see how the merge sort performs against other sorts. We are using the same two classes that we used a couple of lessons ago. This time the Sorter class has three public methods: mergeSort, insertionSort, and selectionSort. You are also given the SortTester class which has three static methods for creating 3 different types of arrays, random, nearly sorted, and reverse sorted. This test is going to be similar to the previous one, but instead of testing three different types of arrays, you are going to test 3 different types of sorts on the same array type. Create an array using the makeRandomArray method, then take a start time using System.currentTimeMillis(). Next, run the array through the one of the sort methods in the Sorter class. Finally, record the end time and print out the results. You will test each of the 3 sorts. Feel free to also test this with…arrow_forward
- how would you do this in a simple way? this is for a non graded practice labarrow_forwardCreate a method that checks if two sets of integers are equivalent by writing a function to compare them. If two lists are drastically different, the method should highlight the greater of their maximum values.arrow_forwardIs there a way to simataniously get both student names' and roll numbers' to be in ascending order at the same time in one block display? What would that look like? Right now I have to call both methods seperatly in order to see both ascending methods in action and it doesn't look good...Also is it possible to separate the sorting logic from the main method and encapsulate it within a separate class or method that takes the ArrayList of students and the two comparators as arguments?... allowing the program to sort students by different criteria without duplicating the sorting logic? The attached image is what my program is displaying right now and I want it to display like: Student [roll number =1, name =Allan, address =620 pioneer st]Student [roll number =2, name =Billy, address =700 ash st]Student [roll number =3, name =Chris, address =801 cheney dr]Student [roll number =4, name =Diana, address =830 washington st]Student [roll number =5, name =Eliza, address =200 main st]Student…arrow_forward
- Calculate the time complexity of the following issue and provide a method. Divide a list of n unique positive numbers into two sublists, each of size n/2, so that the difference in the sums of the integers in the two sublists is as large as possible. You may assume that n is a power of two.arrow_forwardCreate a nested class called DoubleNode that allows you to create doubly-linked lists with each node containing a reference to the item before it and the item after it (or null if neither of those items exist). Then implement static methods for the following operations: insert before a given node, insert after a given node, remove a given node, remove from a given node, insert at the beginning, insert at the end, remove from a given node, and remove a given node.arrow_forwardhow would you do this in a simple way? this is a non graded practice labarrow_forward
- So how fast is the merge sort? Does it depend on the type of array? We saw with some of our previous sorts that it depended on the initial array. For example, an Insertion sort was much faster for a nearly sorted array. In this exercise, you are given the Sorter class, which contains a static mergeSort method that takes an int array and length as an input. You are also given the SortTester class which has three static methods for creating 3 different types of arrays. For this test, create one of the three arrays, then take a start time using System.currentTimeMillis(). Next, run the array through the mergeSort method in the Sorter class. Finally, record the end time and print out the results. Repeat this for the other two array types. Sample Output Random Array: ** Results Hidden ** Almost Sorted Array: ** Results Hidden ** Reverse Array: ** Results Hidden **arrow_forwardMake a function that checks to determine whether two lists of integer values are identical (i.e. contain the same values). The method must provide the maximum value for each list if the lists are not similar.arrow_forwardWithout using the java collections interface (i.e. do not import java.util.List, LinkedList, etc. ) Write a java program that inserts a new String element (String newItem) into a linked list before another specified item (String itemToInsertBefore). For example if items "A", "B", "C" and "D" are in a linked list in that order and the below method is called, insertBefore("E", "C"), then "E" would be inserted before "C", making the final list to be "A", "B", "E", "C" and "D" with no nulls or blank elements or any elements missing or anything. It should work for all lenghths of linkedlists of Strings. public Boolean insertBefore(String newItem, String itemToInsertBefore) { // returns true if done successfully, else returns false if itemToInsertBefore cannot be found or some other error }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
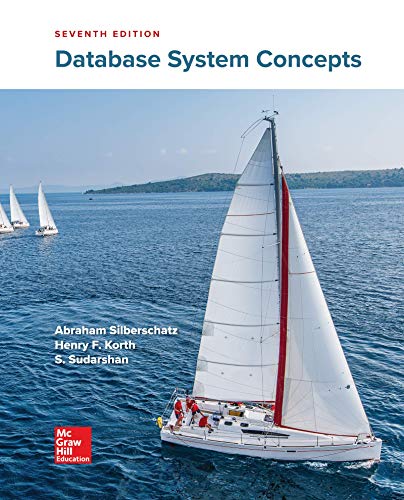
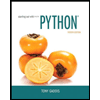
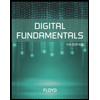
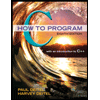
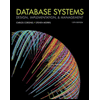
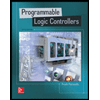