Exempel c:\>java labb2 Enter the word to search for>hello The file 'wordlistl.txt' contains 55899 words The word 'hello' exists in this file The search took 108 ms c:\>java labb2 wordlist2.txt Enter the word to search for>hello The file 'wordlist2.txt' contains l09583 words The word 'hello' exists in this file The search took 169 ms c:\>java labb2 wordlist3.txt Enter the word to search for>hello The file 'wordlist3.txt' contains 354986 words The word 'hello' exists in this file The search took 296 ms c:\>java labb2 Enter the word to search for>xyz The file 'wordlistl.txt' contains 55899 words The word 'xyz' does not exists in this file The search took 116 ms c:\>java labb2 Demo.txt Enter the word to search for>java Exception in thread "main" javai0.FileNotFoundException: finns- inte.txt (We don’t find this file) at java.base/java.io.FileInputStream.open0 (Native Method) at java baseLiavaie:Filelnputstream.open (FileInputStream.java:216) at java.base/java.io.FileInputStream.(FileInputStream.java:157) at java kaseLiava:util:Seanner-Sinit> (Scanner.java:639) at labb2.main(labb2.java:21)
Java Program /The Output attached by image
Overview
You should enter this task, ask the user for a word and then search for a file to see if that word is among the words in the file.
Task
This exercise comes with three text files named wordlist1.txt, wordlist2.txt and wordlist3.txt. These files contain a large amount of words in English. Each file contains more and more words. Each word in these files is on a separate line and the words contain only lowercase letters. Your task is to write a program that reads all these words from the file and checks if a keyword, which the user entered, is among the entered words. The program should also count the total number of words contained in the file used. The program should measure how long it takes to complete the search, ie how long it takes to read all the words and check if the keyword is among the words or not.
The program should start by asking the user for the word to be searched for. Then the words in the file should be counted and compared if the keyword exists or not. A printout should be made where the user is informed if the keyword existed or not, how long (in milliseconds) the search took and how many words the file contains in total.
As an argument to the program, the name of the file to be searched should be specified. If no argument is specified to the program, the file wordlist1.txt is to be used.
Tip
• The search does not have to take into account the exact matching of the keyword parts of words go well. If e.g. the user wants to search for only the letter a, it is enough that a word contains the letter a.
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter filename, inluding file ending> ");
String userInput = input.nextLine();
while (userInput.length() > 0) {
int dotIndex = userInput.lastIndexOf('.');
if (dotIndex == -1) {
System.out.println("Wrong filename format (missing .)\n");
}
else {
String suffix = userInput.substring(dotIndex + 1);
String filename = userInput.substring(0, dotIndex);
System.out.println("\nFilename: " + filename);
System.out.println("File ending: " + suffix + "\n");
}
System.out.print("Enter a new filename (only enter will exit)> ");
userInput = input.nextLine();
}
}
}


Step by step
Solved in 3 steps with 3 images

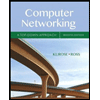
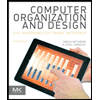
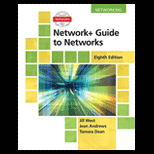
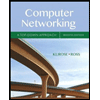
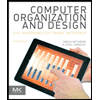
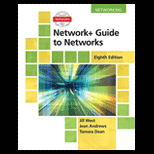
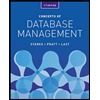
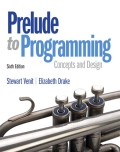
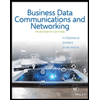