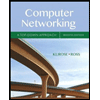
Every cylinder has a base and height, where the base is a circle. Design the class Cylinder that can capture the properties of a cylinder and perform the usual operations on a cylinder. Derive this class from the class Circle (done through Problem 3.2). Please do the following:
- Some of the operations that can be performed on a cylinder are as follows: calculate and print the volume, calculate and print the surface area, set the height, set the radius of the base, and set the center of the base.
- Write a test program to test your program.
Note: Java programming. Please also send the test program so I can run it in dr java and learn. Use given code and design the class cylinder that can capture the properties of a cylinder and perform the usual operations on a cylinder.
Point code:
// class Point
public class Point
{
protected double x, y; // coordinates of the Point
//Default constructor
public Point()
{
setPoint( 0, 0 );
}
//Constructor with parameters
public Point(double xValue, double yValue )
{
setPoint(xValue, yValue );
}
// set x and y coordinates of Point
public void setPoint(double xValue, double yValue )
{
x = xValue;
y = yValue;
}
// get x coordinate
public double getX()
{
return x;
}
// get y coordinate
public double getY()
{
return y;
}
// convert point into String representation
public String toString()
{
return "[" + String.format("%.2f", x)
+ ", " + String.format("%.2f", y) + "]";
}
//Method to compare two points
public boolean equals(Point otherPoint)
{
return(x == otherPoint.x &&
y == otherPoint.y);
}
//Method to compare two points
public void makeCopy(Point otherPoint)
{
x = otherPoint.x;
y = otherPoint.y;
}
public Point getCopy()
{
Point temp = new Point();
temp.x = x;
temp.y = y;
return temp;
}
// print method
public void printPoint()
{
System.out.print("[" + String.format("%.2f", x)
+ ", " + String.format("%.2f", y) + "]");
}
} // end class Point
Circle code:
public class Circle
{
//declaring data members
protected double radius;
protected Point center;
//constructor
public Circle()
{
radius=0;
center=new Point();
}
//parameterized constructor
public Circle(Point p, double radius)
{
center=p;
this.radius=radius;
}
//getters and setters for radius
public double getRadius()
{
return radius;
}
public void setRadius(double radius)
{
this.radius=radius;
}
//getters and setters for center
public void setCenter(Point p)
{
center=p;
}
public Point getPoint()
{
return center;
}
//finding area
public double getArea()
{
return 3.14*radius*radius;
}
//finding circumference
public double getCircumference()
{
return 2*3.14*radius;
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- An airport van shuttles passengers between the terminal and the parking garage. After 4 trips, the tank is empty, and the van needs to drive to the gas station. After it has been refilled, it will drive to the terminal. The behavior of the van depends on two states: its location (terminal, garage, or gas station), and the tank level (empty or not). Complete the following class. The constructor yields a van in the garage with a full tank.arrow_forwardYou need to perform following things to demonstrate the concept of abstract class. Step 1: Create the abstract class that has following methods. • Calculate the area of circle(Abstract method) • Calculate the area of triangle(Abstract method) • Calculate the area of rectangle(Method with implementation) Step 2: Create another class that extends above class and provide implementation of abstract methods. Step 3: Create the main method inside above class and display output of area of circle, area of triangle and area of rectangle. You need to submit the following things: • Java program with both the classes An output screenshot created using Microsoft Wordarrow_forwardCorrect and detailed answer will be Upvoted else downvoted. Thank you!arrow_forward
- 1. Define a class named Randomwalker. A Randomwalker object should keep track of its (x, y) location. All walkers start at the coordinates (0, 0). When a walker is asked to move, it randomly moves either left, right, up or down. Each of these four moves should occur with equal probability. The resulting behavior is known as a "random walk." (A 2-dimensional random walk example is pictured at right.) Each Randomwal ker object should have the following public methods. You may add whatever fields or methods you feel are necessary to implement these methods: move () Instructs this random walker to randomly make one of the 4 possible moves (up, down, left, or right). getX () Returns this random walker's current x-coordinate. getY () Returns this random walker's current y-coordinate. getsters () Returns the number of steps this random walker has taken.arrow_forwardDesign and implement the class Day that implements the day of the week in a program. The program should be able to perform the following operations on an object of the type Day: Set the day. Print the day. Return the day. Return the next day. Return the previous day. Add a comment with your full name in it in any part of the program.arrow_forwardConsider the definition of the following class: public class CC { private int u; private int v; private double w; public CC( ) //Line 1 {…} public CC(int a) //Line 2 {…} public CC(int a, int b) //Line 3 {…} public CC(int a, int b, double d) //Line 4 {…} A. Give the line number containing the constructor that is executed in each of the following declarations: (i) CC one = new CC( ); _________________ (ii) CC two = new CC(5, 6); _________________ (iii) CC three = new CC(2, 8, 3.5); _________________ B. Write the definition of the constructor in Line 1 so that the instance variables are initialized to 0. C.Write the definition of the constructor in Line 4 so that the instance variables u, v, and w are initialized according to the values of the parameters a, b, and d,…arrow_forward
- Java programarrow_forwarda:) Define a Polygon interface that has methods area() and perimeter(). b:) Then implement classes for Triangle, Quadrilateral, Pentagon, Hexagon, and Octagon, which implement this interface, with the obvious meanings for the area() and perimeter() methods. c:) Implement classes, IsoscelesTriangle, Equilateral-Triangle, Rectangle, and Square, which have the appropriate inheritance relationships. d:) Write a simple user interface, which allows users to create polygons of the various types, input their geometric dimensions, and then output their area and perimeter. e:) For extra effort, allow users to input polygons by specifying their vertex coordinates and be able to test if two such polygons are similar. using all a to e parts develop a programarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
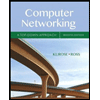
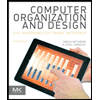
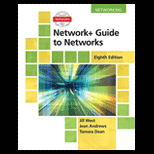
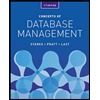
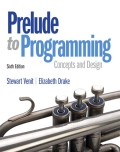
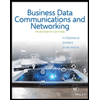