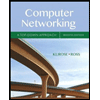
//below is the code I need help with answering the questions in the image//
#include <stdio.h>
#include <stdlib.h>
struct employees
{
char name[20];
int ssn[9];
int yearBorn, salary;
};
// function to read the employee data from the user
void readEmployee(struct employees *emp)
{
printf("Enter name: ");
gets(emp->name);
printf("Enter ssn: ");
for (int i = 0; i < 9; i++)
scanf("%d", &emp->ssn[i]);
printf("Enter birth year: ");
scanf("%d", &emp->yearBorn);
printf("Enter salary: ");
scanf("%d", &emp->salary);
}
// function to create a pointer of employee type
struct employees *createEmployee()
{
// creating the pointer
struct employees *emp = malloc(sizeof(struct employees));
// function to read the data
readEmployee(emp);
// returning the data
return emp;
}
// function to print the employee data to console
void display(struct employees *e)
{
printf("%s", e->name);
printf(" %d%d%d-%d%d-%d%d%d%d", e->ssn[0], e->ssn[1], e->ssn[2], e->ssn[3], e->ssn[4], e->ssn[5], e->ssn[6], e->ssn[7], e->ssn[8]);
printf(" %d", e->yearBorn);
printf("\n$%d.", e->salary);
}
// function to free the memory of the employee pointer
void releaseEmployee(struct employees *e)
{
free(e);
}
// main method
int main()
{
// creating the employee
struct employees *emp = createEmployee();
// printing the information
display(emp);
// free the memory
releaseEmployee(emp);
return 0;
}
![Q1: How many total calls to malloc are made during createEmployee (including
readEmployee and anything it calls)?
Q2: If the employee's name is Joe Smith, how much total memory is allocated
for the structure and the name (not just for the name)?
Q3: Many people put the SSN in a char[9] field (I kind of encouraged it). Is that
big enough to hold the ssn string? Why didn't it create a problem?
Q4: How many calls to free do you need to make? Does it agree with the number
of calls to malloc that were made?
Q5: What would happen if you passed in the address of the initialized global
variable into releaseEmployee? Would anything different happen if you passed in
the address of a local variable (on the stack)?](https://content.bartleby.com/qna-images/question/2c4d07ff-5ab1-477f-b7b6-63a497af629e/f1c917c9-dbea-4ab4-9911-5c9bb3f5fe5f/z0qe8w_thumbnail.png)

Step by stepSolved in 2 steps

- //below is the code I need help with answering the questions in the image// #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for (int i = 0; i < 9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to print the employee data to console void display(struct employees *e) { printf("%s",…arrow_forward// MichiganCities.cpp - This program prints a message for invalid cities in Michigan. // Input: Interactive // Output: Error message or nothing #include <iostream> #include <string> using namespace std; int main() { // Declare variables string inCity; // name of city to look up in array const int NUM_CITIES = 10; // Initialized array of cities string citiesInMichigan[] = {"Acme", "Albion", "Detroit", "Watervliet", "Coloma", "Saginaw", "Richland", "Glenn", "Midland", "Brooklyn"}; bool foundIt = false; // Flag variable int x; // Loop control variable // Get user input cout << "Enter name of city: "; cin >> inCity; // Write your loop here // Write your test statement here to see if there is // a match. Set the flag to true if city is found. // Test to see if city was not found to determine if // "Not a city in Michigan" message should be printed.…arrow_forwardAssign pizzasInStore's first element's numCalories with the value in pizzasInStore's second element's numCalories. #include <stdio.h>#include <string.h> typedef struct Pizza_struct { char pizzaName[75]; int numCalories;} Pizza; int main(void) { Pizza pizzasInStore[2]; scanf("%s", pizzasInStore[0].pizzaName); scanf("%d", &pizzasInStore[0].numCalories); scanf("%s", pizzasInStore[1].pizzaName); scanf("%d", &pizzasInStore[1].numCalories); /* Your code goes here */ printf("A %s slice contains %d calories.\n", pizzasInStore[0].pizzaName, pizzasInStore[0].numCalories); printf("A %s slice contains %d calories.\n", pizzasInStore[1].pizzaName, pizzasInStore[1].numCalories); return 0;} I tried 10 time but I cant solve it so please help mearrow_forward
- #include #include #include "Product.h" using namespace std; int main() { vector productList; Product currProduct; int currPrice; string currName; unsigned int i; Product resultProduct; cin>> currPrice; while (currPrice > 0) { } cin>> currPrice; main.cpp cin>> currName; currProduct.SetPriceAndName (currPrice, currName); productList.push_back(currProduct); resultProduct = productList.at (0); for (i = 0; i < productList.size(); ++i) { Type the program's output Product.h 1 CSE Scanned Product.cpp if (productList.at (i).GetPrice () < resultProduct.GetPrice ()) { resultProduct = productList.at(i); } AM cout << "$" << resultProduct.GetPrice() << " " << resultProduct. GetName() << endl; return 0; Input 10 Cheese 6 Foil 7 Socks -1 Outputarrow_forwardC PROGRAMMING HELP I need help fixing an old assignment of mine. The point of the script is to read the pasted/attached Number_new.txt file's contents, meant to represent a classes' final grades out of 100 percent, and print an .exe screen as well as another .txt file that repeats the grade number with a letter grade and a PASS or FAIL next to it. At the end it needs to say how many students passed and how many failed. Nothing too complicated with the grading, just 90.0 and up is an A, 80.0 to 89.99 is a B, etc. And of course, anything below 60.0 is an F/FAIL. Please NO IOSTREAM.H OR CACIO.H! I cant use those header files. Below is the script: #include<stdio.h>#include<math.h>#define THEFILE "Number_new.txt" int main(){ float r0[10]; float rr[10]; FILE *fp; char line[81]; fp = fopen(THEFILE, "Number_new.txt"); if (fp != NULL) { /* The file is open. */ printf("%7s %7s %7s %7s %7s %7s %7s %7s %7s %7s\n", "0", "0.01",…arrow_forwardWrite a function getNeighbors which will accept an integer array, size of the array and an index as parameters. This function will return a new array of size 2 which stores the neighbors of the value at index in the original array. If this function would result in returning garbage values the new array should be set to values {0,0} instead of values from the array.arrow_forward
- Programming Language: C++ Please use the resources included and provide notes for understanding. Thanks in advance. Code two functions to fill an array with the names of every World Series-winning team from 1903 to 2020, then output each World Series winner with the number of times the team won the championship as well as the years they won them. The input file is attached, along with the main function and screenprint. Please note team names that include two words, such as Red Sox, have an underscore in place of the space. This enables you to use the extraction operator with a single string variable. The following resources are included: Here is main. #include <iostream>#include <fstream>#include<string> using namespace std; // Add function declarations and documentation here void fill(string teams[], int size);void findWinner(string teams[], int size); int main(){ const int SIZE = 118;int lastIndex;string team[SIZE]; fill(team, SIZE);findWinner(team, SIZE); return…arrow_forwardIn C++Using the code provided belowDo the Following: Modify the Insert Tool Function to ask the user if they want to expand the tool holder to accommodate additional tools Add the code to the insert tool function to increase the capacity of the toolbox (Dynamic Array) USE THE FOLLOWING CODE and MODIFY IT: #define _SECURE_SCL_DEPRECATE 0 #include <iostream> #include <string> #include <cstdlib> using namespace std; class GChar { public: static const int DEFAULT_CAPACITY = 5; //constructor GChar(string name = "john", int capacity = DEFAULT_CAPACITY); //copy constructor GChar(const GChar& source); //Overload Assignment GChar& operator=(const GChar& source); //Destructor ~GChar(); //Insert a New Tool void insert(const std::string& toolName); private: //data members string name; int capacity; int used; string* toolHolder; }; //constructor GChar::GChar(string n, int cap) { name = n; capacity = cap; used = 0; toolHolder = new…arrow_forwardpython: numpy def purchases(transactions): """ QUESTION 7 - A high-end store is trying to evaluate the total amount that customer's spend per transaction. They want customers to spend anywhere between $130 and $150 on average. - You need to determine whether the average number spent on each transaction per month is above, between, or below the desired amount. - Transactions is a numpy array containing a date, total amount earned each month, and total number of transactions each month. - Above: month's average amount spent per transaction > 150 - Within Range: 150 >= month's average amount spent per transaction >= 130 - Below: month's average amount spent per transaction < 130 - Return a numpy array with "Above", "Within Range" and "Below" for the average amount spent per transaction per month - THIS MUST BE DONE IN ONE LINE HINT: use np.where() and convert the type of each column to float Args: transactions…arrow_forward
- C# application that will allow users to enter values store all the expenses on that month in an array clothes, food, cool drinks, water, meatarrow_forwardWhen traversing an array, which of the following statements does not need to keep track of the individual array subscripts: Do...Loop, For...Next, or For Each...Next?arrow_forwardC++ Programming Code two functions to fill an array with the names of every World Series-winning team from 1903 to 2020, then output each World Series winner with the number of times the team won the championship as well as the years they won them. The input file is attached, along with the main function and screenprint. Please note team names that include two words, such as Red Sox, have an underscore in place of the space. This enables you to use the extraction operator with a single string variable. The following resources are included: Here is main. #include <iostream>#include <fstream>#include<string> using namespace std; // Add function declarations and documentation here void fill(string teams[], int size);void findWinner(string teams[], int size); int main(){ const int SIZE = 118;int lastIndex;string team[SIZE]; fill(team, SIZE);findWinner(team, SIZE); return 0;} // Add function definitions here WorldSeriesChampions.txt…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
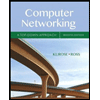
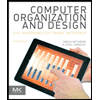
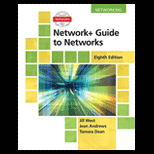
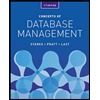
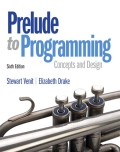
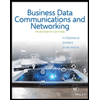