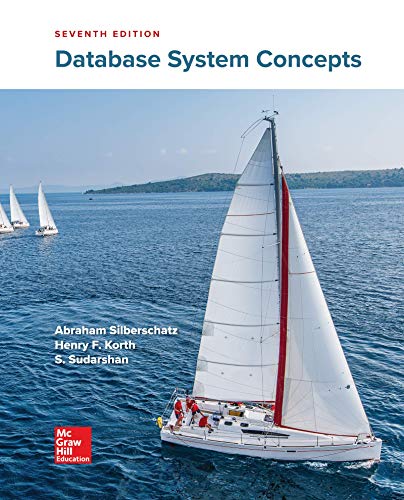
Create a flowchart for the C++ code below
#include <iostream>
using namespace std;
int main ()
{
int first_opt, area_opt, perimeter_opt, length, width, radius, side, height, base, a, b, c, A_Rect, A_Square, P_Rect, P_Square, P_Triangle;
float A_Circle, A_Triangle, P_Circle;
cout<<"Welcome!. Choose what you want to solve"<<"\n";
cout<<"1. AREA"<<"\n";
cout<<"2. PERIMETER"<<"\n";
cin>>first_opt;
cout<<"Choose from the following"<<"\n";
switch(first_opt)
{
case 1:
cout<<"1. Area of the Rectangle"<<"\n";
cout<<"2. Area of the Circle"<<"\n";
cout<<"3. Area of the Square"<<"\n";
cout<<"4. Area of the Triangle"<<"\n";
cin>>area_opt;
switch(area_opt)
{
case 1:
cout<<"Enter length: "<<"\n";
cin>>length;
cout<<"Enter width: "<<"\n";
cin>>width;
A_Rect = length*width;
cout<<"The area of the rectangle is: "<<A_Rect<<"\n";
case 2:
cout<<"Enter radius: "<<"\n";
cin>>radius;
A_Circle = 3.1416*radius*radius;
cout<<"The area of the circle is: "<<A_Circle<<"\n";
case 3:
cout<<"Enter side: "<<"\n";
cin>>side;
A_Square = side*side;
cout<<"The area of the square is: "<<A_Square<<"\n";
case 4:
cout<<"Enter height: "<<"\n";
cin>>height;
cout<<"Enter base: "<<"\n";
cin>>base;
A_Triangle = (height*base)/2;
cout<<"The area of the triangle is: "<<A_Triangle<<"\n";
default:
cout<<"out of options";
break;
}
break;
case 2:
cout<<"1. Perimeter of the Rectangle"<<"\n";
cout<<"2. Perimeter of the Circle"<<"\n";
cout<<"3. Perimeter of the Square"<<"\n";
cout<<"4. Perimeter of the Triangle"<<"\n";
cin>>perimeter_opt;
switch(perimeter_opt)
{
case 1:
cout<<"Enter length: "<<"\n";
cin>>length;
cout<<"Enter width: "<<"\n";
cin>>width;
P_Rect = 2*(length+width);
cout<<"The perimeter of the rectangle is: "<<P_Rect<<"\n";
case 2:
cout<<"Enter radius: "<<"\n";
cin>>radius;
P_Circle = 3.1416*radius*2;
cout<<"The perimeter of the circle is: "<<P_Circle<<"\n";
case 3:
cout<<"Enter side: "<<"\n";
cin>>side;
P_Square = 4*side;
cout<<"The perimeter of the square is: "<<P_Square<<"\n";
case 4:
cout<<"Enter the length of first side: "<<"\n";
cin>>a;
cout<<"Enter the length of second side: "<<"\n";
cin>>b;
cout<<"Enter the length of third side: "<<"\n";
cin>>c;
P_Triangle = a+b+c;
cout<<"The area of the triangle is: "<<P_Triangle<<"\n";
default:
cout<<"out of options";
break;
}
break;
default:
cout<<"out of options";
break;
}
return 0;
}

Step by stepSolved in 2 steps with 1 images

- Convert the follwoing code into a object-oriented programming (OOP) application using secure and efficient C++ code. #include <iostream> #include <iomanip> using namespace std; int main(){ float inital_Inv; float month_Dep; float annual_Int; float num_Years; float total_Ammt; float int_Amunt; float year_Inest; try { cout << "*************************" << endl; cout << "********Data Input*******" << endl; cout << "Initial Investment Amount: $ "; cin >> inital_Inv; cout << "Monthly Deposit: $ "; cin >> month_Dep; cout << "Annual Interest: % "; cin >> annual_Int; cout << "Number of years: "; cin >> num_Years; } catch (float x) { } total_Ammt = inital_Inv; cout << "\tBalance and Interest Without Additional Monthly Deposits" <<…arrow_forwardThis is the C code I have so far #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; struct employees **emps = new employees()[10]; //Added new statement ---- bartleby // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for(int i =0; i <9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to print the employee data to console void display(struct employees…arrow_forwardConsider the following C++ codes: Code a: Code b: int x 8; // global void func () int x 8; // global int age=18; // global void func () { int age=18; cout<<"X is: "<arrow_forward/* Programming concept: structures Program should: be a C program, define a structure to store the year, month, day, and high temperature. write a function get_data that has a parameter that is a pointer to a struct and reads from the user into the struct pointed to by the parameter. write a function print_data that takes the struct pointer as a parameter and prints the data. in main, declare a struct, call the get_data function, call the print_data function. */ #include <stdio.h> int main(int argc, char *argv[]) { return 0;}arrow_forwardWhat is the pseudocode for this? Below is the C++ #include <iostream> using namespace std; int main () { int first_opt, area_opt, perimeter_opt, length, width, radius, side, height, base, a, b, c, A_Rect, A_Square, P_Rect, P_Square, P_Triangle; float A_Circle, A_Triangle, P_Circle; cout<<"Welcome!. Choose what you want to solve"<<"\n"; cout<<"1. AREA"<<"\n"; cout<<"2. PERIMETER"<<"\n"; cin>>first_opt; cout<<"Choose from the following"<<"\n"; switch(first_opt) { case 1: cout<<"1. Area of the Rectangle"<<"\n"; cout<<"2. Area of the Circle"<<"\n"; cout<<"3. Area of the Square"<<"\n"; cout<<"4. Area of the Triangle"<<"\n"; cin>>area_opt; switch(area_opt) { case 1: cout<<"Enter length: "<<"\n"; cin>>length; cout<<"Enter width: "<<"\n"; cin>>width; A_Rect = length*width; cout<<"The area of the…arrow_forwardThis is the C code I have so far #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; struct employees **emps = new employees()[10]; //Added new statement ---- bartleby // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for(int i =0; i <9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to print the employee data to console void display(struct employees…arrow_forwardGiven the following set of code, select all C++ expressions: 4₁ #include #include #include #include "grocery_list_lib.h" // Returns an array of pair objects of item and amount std::array, 5> create_grocery_list (){ std::array, 5 grocery_list; std::string item; std::string item_amount; F3 double amount; for(int i = 0; i > amount grocery_list[i].second = amount; } return grocery_list; } grocery_list[i].first std::string item; item_amount return grocery_list; "Enter item: $ 4 4) R Q Search DII % 5 T F5 6 LO F6 Y F7 & 7 U BO PrtScn F8 8 Home F9 9 End F10 Po 0arrow_forwardC++ Fix this code so that it displays in the format like the image shown where the principal and rate should have a fixed precision of 2 for each of the five percentages 5%,6%,7%,8%,9%,10%. The amount of the deposit should not have the fixed precision and should remain in the decimal format. #include <iostream>#include <iomanip>#include <cmath>using namespace std; int main(){double principal{ 1000 };double rate{ 0.05 }; for (rate; rate <= 0.10; rate += 0.01) {cout << "" << endl;cout << "Initial principal: " << principal << endl;cout << "Interest Rate: " << rate * 100 << "%" << endl;cout << setw(4) << "Year" << setw(20) << "Amount on deposit" << endl;for (unsigned int year{ 1 }; year <= 10; year++) {double amount = principal * pow(1.0 + rate, year); cout << setw(4) << year << setw(20) << amount << endl; }}}arrow_forwardC++arrow_forwardNot C++ Only Carrow_forwardLanguage only c++arrow_forwardC++ shapes.h: #include <iostream>#include <cmath>struct Point2d{double x;double y;void print(){// example: (2.5,3.64)std::cout << "(" << x << "," << y << ")" << std::endl;}double length() const{return std::sqrt(x * x + y * y);}// const Point2D & ==> function can't modify 'other'// the second const ==> function can't modify x, yPoint2d add(const Point2d &other) const{Point2d result;result.x = x + other.x;result.y = y + other.y;return result;}};// example of an interfacestruct Shape{virtual bool contains(Point2d p) = 0;};struct Circle : Shape{Point2d center;double radius;bool contains(Point2d p){Point2d diff;diff.x = p.x - center.x;diff.y = p.y - center.y;double distToCenter = diff.length();return distToCenter <= radius;}};// TODO: replace this with your Rectangle implementationstruct Rectangle : Shape{// TODO: which member variables to model a rectangle?bool contains(Point2d p){return false; // TODO }};//…arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
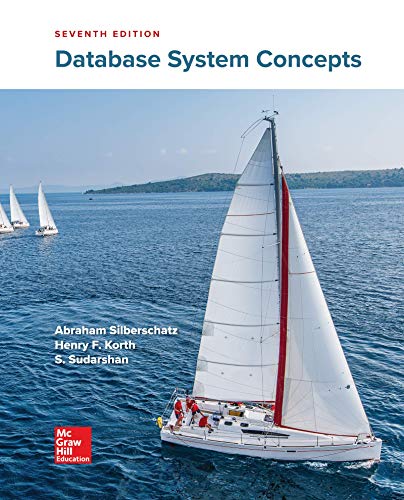
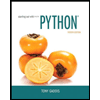
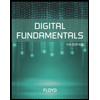
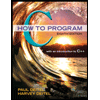
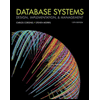
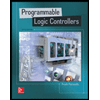