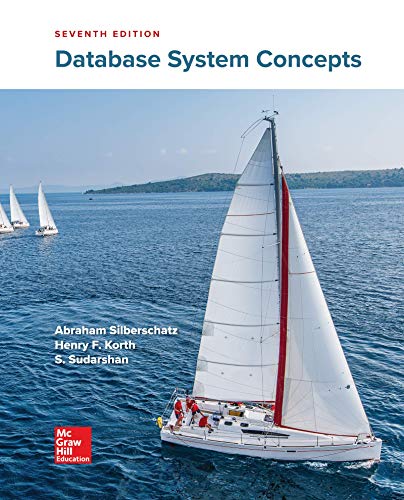
I need help in C
Given structs
#define MAXSIZE 100
#define MAXSLOTS 100
// DO NOT change the following typedef
typedef char String[11];
// date structure data type
struct dateTag {
int month;
int day;
int year;
};
typedef struct dateTag dateType;
// schedule structure data type
struct schedTag {
dateType date;
int n_slots_taken; // number of slots already taken
int VID[MAXSLOTS]; // VID means Vaccinee ID. VID[i] stores the ID number of the person to be vaccinated
// ex. VID[0] = 102
// means that the 1st person to be vaccinated during
// schedule is the person with ID number 102.
};
typedef struct schedTag schedType;
// name structure data type
struct nameTag {
String last;
String first;
};
typedef struct nameTag nameType;
// vaccinee structure data type
struct vaccineeTag {
int ID; // ID number of the vaccinee, note that this number is system-generated (i.e., not a user input)
nameType name; // name of vacinee
int age; // age of vacinee
int bool_frontliner; // 0 means not frontliner, 1 means frontliner
int bool_comorbidity; // 0 means no comorbidity, 1 means with comorbiditity
int priority; // 0 means priority not yet set, 1 to 4 means priority is set
// where 1 is the highest priority and 4 is the lowest priority
};
typedef struct vaccineeTag vaccineeType;
Create this funcion-
TO DO #1: Implement Search_by_Name() Implement a linear search
@param: name_key is the search key (containing the last name and first name of the person to be searched)
@param: VACCINEE[] is the array of structures (containing info about people who will be scheduled for vaccination)
@param: n is the actual number of elements in VACCINEE[] where n <= MAXSIZE.
returns: if name_key is found, return the index where name_key was found in VACCINEE[];
otherwise, return a value of -1.
*/
int
Search_by_Name(nameType name_key, vaccineeType VACCINEE[], int n)
{
/*
Declare your own local variables.
Do NOT call printf() or scanf() in this function.
*/
return 888; // Do NOT forget to return a value. You'll need to change 888.
}

Step by stepSolved in 2 steps

- A member of a structure or of a class is accessed using : c++ A. Comma operator B. The dot operator C. The indexing operator D. The ampersand operatorarrow_forwardIn C Write the function definition of a function named “sale”as follows: The function accepts two arguments of typefloat, one is the item total and the other is the sale taxpercentage (out of 100%). The function should returnthe amount of tax calculated for the purchase.arrow_forwardUSING THE C PROGRAMMING LANGUAGE Define a structure tyve called subscriber_t that contains the componenets name, streed_address, and monthly_bill. Just write the structure definition, No function or other code is requiredarrow_forward
- Programing in Carrow_forwardQUESTION 3 Write a C++ program that takes car information, compares the car prices, and displays the car information with the highest price. In this program, a structure named Car containing three data members which are model, price, and cc must be defined. The two structure variables namely carl and car2 are created to store the car information entered by the user. Then, the prices of the two cars are compared, and the car information with the highest price is stored in a structure variable named carMax. Finally, the program displays the car information (model, price, and cc) from the structure variable carMax.arrow_forwardC++ enum Major {COMPUTER_SCIENCE, COMPUTER_SECURITY_INFO_ASSURANCE, CYBER_SECURITY, ELECTRICAL_ENGINEERING, OTHER}; struct Name { string first; string last; char middleInitial; }; struct StudentRecord { Name studentName; Major major; string email; }; StudentRecord student12; Suppose you are student12. Show the C++ statements used to fill in all of your information for your StudentRecord. Show how would you assign the third letter from the email field to a local char variable called myChar.arrow_forward
- C# helparrow_forwardChoice the correct answerarrow_forwardDefine a struct type that represents an exercise plan. The struct should store the following data: plan name (a character array of size 50) and goal calories to burn (an integer). The type should be renamed from struct exercise_plan to Exercise_plan.arrow_forward
- Programming Skills: Complete the function egg_category which returns a str describing an egg's category given its int weight in grams. Here is a table specifying the weight ranges if an egg's weight is on the boundary between two category ranges, it is assigned the smaller category. Category Small Meduim Weight no more than 50 grams 50-57 grams Category Large Jumbo Weight 57-64 grams more than 64 grams def egg_category (weight): %# Return a str describing the category of an egg of the specified int weight.arrow_forwardtypedef char string[50]; struct a Tag { int a; }; // 1. indicate the data type returned by function Exam() Exam(struct a Tag *ptr) { float b; double C[ 10 ]; string s; } // Access the members of the structure variable indirectly via ptr // You are REQUIRED to use the structure pointer operator; see comments numbered 2 to 5. // 2. input the value of member a scanf("%d", ); // 3. assign 3.1416 as the value of member b = 3.1416; // 4. print the value of the last element of array C. printf("%lf\n", |); // 5. copy "Hello" into string s strcpy( return ptr; , "Hello");arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
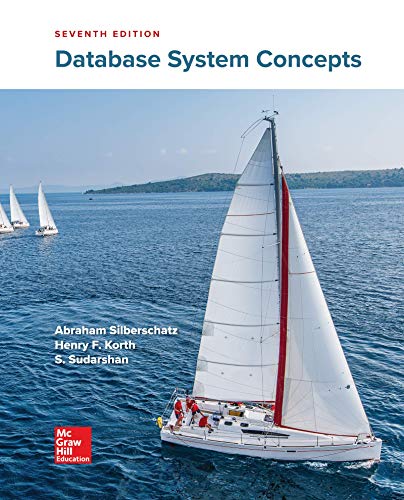
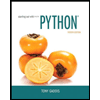
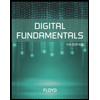
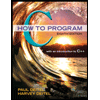
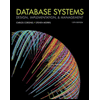
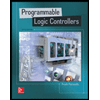