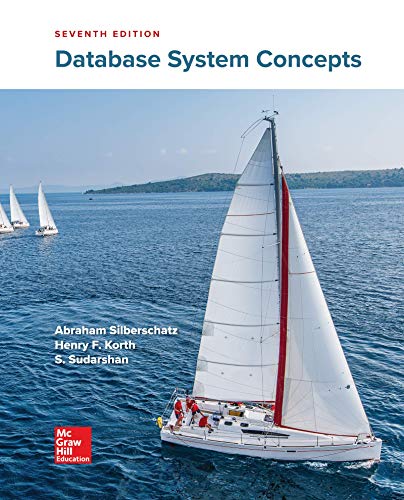
def__init__(self):
"""
-------------------------------------------------------
Initializes an empty Queue. Data is stored in a Python list.
Use: source = Queue()
-------------------------------------------------------
Returns:
a new Queue object (Queue)
-------------------------------------------------------
"""
self._values = []
defis_empty(self):
"""
-------------------------------------------------------
Determines if source is empty.
Use: b = source.is_empty()
-------------------------------------------------------
Returns:
True if source is empty, False otherwise.
-------------------------------------------------------
"""
return len(self._values) == 0
def__len__(self):
"""
-------------------------------------------------------
Returns the length of source.
Use: n = len(source)
-------------------------------------------------------
Returns:
the number of values in source (int >= 0)
-------------------------------------------------------
"""
return len(self._values)
definsert(self, value):
"""
-------------------------------------------------------
Adds a copy of value to the rear of source.
Use: source.insert(value)
-------------------------------------------------------
Parameters:
value - data (*)
Returns:
None
-------------------------------------------------------
"""
self._values.append(deepcopy(value))
return
defremove(self):
"""
-------------------------------------------------------
Removes and returns the front value from source.
Use: value = source.remove()
-------------------------------------------------------
Returns:
value - the value at the front of source, the value is
removed from source (*)
-------------------------------------------------------
"""
assert len(self._values) > 0, "Cannot remove from an empty queue"
value = self._values.pop(0)
return value
defpeek(self):
"""
-------------------------------------------------------
Peeks at the front of source.
Use: value = source.peek()
-------------------------------------------------------
Returns:
value - a copy of the value at the front of source,
the value is not removed from source (*)
-------------------------------------------------------
"""
assert len(self._values) > 0, "Cannot peek at an empty queue"
value = deepcopy(self._values[0])
return value

Step by stepSolved in 4 steps with 4 images

- from copy import deepcopy class Stack: def__init__(self): """ ------------------------------------------------------- Initializes an is_empty stack. Data is stored in a Python list. Use: s = Stack() ------------------------------------------------------- Returns: a new Stack object (Stack) ------------------------------------------------------- """ self._values = [] defis_empty(self): """ ------------------------------------------------------- Determines if the stack is empty. Use: b = s.is_empty() ------------------------------------------------------- Returns: True if the stack is empty, False otherwise ------------------------------------------------------- """ empty = True if len(self._values) > 0: empty = False return empty defpush(self, value): """ ------------------------------------------------------- Pushes a copy of value onto the top of the stack. Use: s.push(value) ------------------------------------------------------- Parameters: value - a data element (?)…arrow_forwardComputer Science lab3.h ------------- #include<stdio.h> #include<stdlib.h> #ifndef LAB3_H #define LAB3_H // A linked list node struct Node { int data; //Data struct Node *next; // Address to the next node }; //initialize: create an empty head node (whose "data" is intentionally missing); This head node will not be used to store any data; struct Node *init () { //create head node struct Node *head = (struct Node*)malloc(sizeof(struct Node)); } //Create a new node to store data and insert it to the end of current linked list; the head node will still be empty and data in the array in "main.c" are not stored in head node void insert(struct node *head, int data) { struct Node *newNode = (struct Node*)malloc(sizeof(struct Node)); new_node->data = data; new_node->next= head; } //print data for all nodes in the linked list except the head node (which is empty) void display (struct Node *head) { struct Node *current_node = head; while ( current_node != NULL) { printf("%d ",…arrow_forwardAssume the Queue is initially empty. class Queue { public: int size () ; bool empty (); chará front () throw (Exception); void enqueue (char& e); void dequeue () throw (Exception); Function call Output Queue Contents (back -> front) enqueue ('A') enqueue ('B') front ( ) size ( ) dequeue () enqueue ('C') empty( ) dequeue ( ) size( ) dequeue () empty ( ) front ( ) enqueue ('D') front () dequeue () dequeue ( )arrow_forward
- In Java, a linked list always terminates with a node that is null. True O Falsearrow_forwardInput : 1->2->3->2->1->NULL Output: It's a palindrome !!! Create a palindrome of your student ID and then push the element to the stack and queue. Pop each element from the stack and the queue and then check for the mismatch.arrow_forwardNote : addqueue works like Enqueue and deleteQueue works like Dequeue Consider the following statements: (8, 9) queueType queue; int num; Show what is output by the following segment of code num = 7; queue.addQueue (6); queue.addQueue (num); num = queue.front (); queue.deleteQueue(); queue.addQueue (num + 5); queue.addQueue (14); queue.addQueue (num queue.addQueue (25); queue.deleteQueue (); 2); cout <« "Queue elements: "; while (!queue.isEmptyQueue ()) { cout <« queue.front () << " "; queue.deleteQueue(); } cout <« endl; Queue elements: 14 14 4 25 Queue elements: 11 14 4 4 Queue elements: 11 14 4 25 Queue elements: 11 14 25 25arrow_forward
- Please written by computer source In java please!arrow_forwardQueueArray.java This file implements QueueInterface.java This file has * attributes of an array holding queue elements. An integer represents front * index, an integer for rear index, and an integer to keep track number of * elements in the queue. An overloaded constructor accepts an integer for * initializing the queue size, e.g. array size. An enqueue method receives an * object and place the object into the queue. The enqueue method will throw * exception with message "Overflow" when the queue is full. A dequeue method * returns and removes an object from front of the queue. The dequeue method * will throw exception with message "Underflow" when the queue is empty. A size * method returns number of elements in the queue. A toString method returns a * String showing size and all elements in the queue.arrow_forwardBankCustomerQueueADT Implement in C++ a BankCustomer QueueADT that does the following: 1. gets the input about a customer (first name, last name, Bank Account Number, Money to be withdrawn/deposited, etc.) from the user (from the keyboard) 2. inserts (enqueues) the Customer as a record into the BankCustomerQueueADT (your Queue ADT must be able to have a maximum of 20 customer records) 3. removes (dequeues) the Customer record from the BankCustomerQueueADT 4. your BankCustomerQueueADT must also have the behavior of retrieving and displaying the Queue info on the screen after each event (either entry of a Bank Customer into the queue, OR leaving of the Bank customer from the queue). NOTE: Please make sure you debug, run, test and see the program working correctly before submitting. Make sure all the code statements are commented. Make sure your program is indented properly.arrow_forward
- Data Structures/Algorithms in Javaarrow_forwarddetermine if the statement is true or false If N represents the number of elements in the queue, then the size method of the ArrayBoundedQueue class is O(N).arrow_forwardC++ Hello im trying to make a list for my code but i cant use the <list> library, how would i make a list for my linked list array based hashtable code wordlist: incase you want to test the entire code a aaa hello goodbye #pragma once //hashtable.h #include <iostream> #include <string> #include <list> #include <cstdlib> #include "word.h" using namespace std; class HashTable { int BUCKET; list<Word>* table; public: HashTable(int W); //to check if the table is empty bool isEmpty() const; //to insert a key into the hashtable void insertItem(string& x); //to delete a key from the hashtable void deleteItem(int key); int hashFunction(Word x); //to look for the key in the table bool searchTable(string& key); void displayHash(); }; //hashtable.cpp #include "hashtable.h" #include <iostream> HashTable::HashTable(int W) { table = new list < Word >[W]; BUCKET = W; return; } //to check if the table is empty bool…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
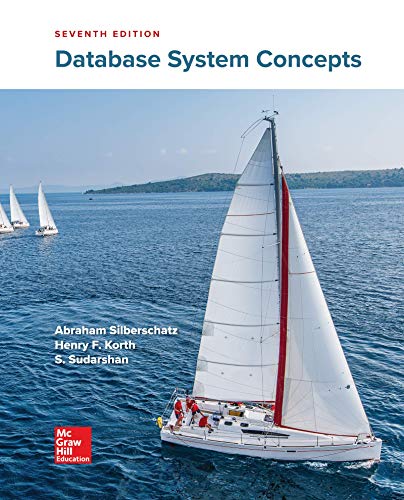
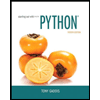
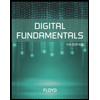
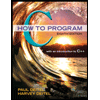
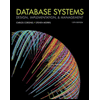
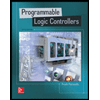