from copy import deepcopy class Stack: def__init__(self): """ ------------------------------------------------------- Initializes an is_empty stack. Data is stored in a Python list. Use: s = Stack() ------------------------------------------------------- Returns: a new Stack object (Stack) ------------------------------------------------------- """ self._values = [] defis_empty(self): """ ------------------------------------------------------- Determines if the stack is empty. Use: b = s.is_empty() ------------------------------------------------------- Returns: True if the stack is empty, False otherwise ------------------------------------------------------- """ empty = True if len(self._values) > 0: empty = False return empty defpush(self, value): """ ------------------------------------------------------- Pushes a copy of value onto the top of the stack. Use: s.push(value) ------------------------------------------------------- Parameters: value - a data element (?) Returns: None ------------------------------------------------------- """ self._values.append(deepcopy(value)) return defpop(self): """ ------------------------------------------------------- Pops and returns the top of stack. The value is removed from the stack. Attempting to pop from an empty stack throws an exception. Use: value = s.pop() ------------------------------------------------------- Returns: value - the value at the top of the stack (?) ------------------------------------------------------- """ assert len(self._values) > 0, "Cannot pop from an empty stack" value = self._values.pop() return value defpeek(self): """ ------------------------------------------------------- Returns a copy of the value at the top of the stack. Attempting to peek at an empty stack throws an exception. Use: value = s.peek() ------------------------------------------------------- Returns: value - a copy of the value at the top of the stack (?) ------------------------------------------------------- """ assert len(self._values) > 0, "Cannot peek at an empty stack" value = deepcopy(self._values[-1]) return value def__iter__(self): """ FOR TESTING ONLY ------------------------------------------------------- Generates a Python iterator. Iterates through the stack from top to bottom. Use: for v in s: ------------------------------------------------------- Returns: value - the next value in the stack (?) ------------------------------------------------------- """ for value inself._values[::-1]: yield value defsplit_alt(self): """ ------------------------------------------------------- Splits the source stack into separate target stacks with values alternating into the targets. At finish source stack is empty. Use: target1, target2 = source.split_alt() ------------------------------------------------------- Returns: target1 - contains alternating values from source (Stack) target2 - contains other alternating values from source (Stack) ------------------------------------------------------- """ i = 0 length = len(self._values) target1 = Stack() target2 = Stack() while i < length: if (i % 2) == 0: target1.push(self._values[(len(self._values) - 1)]) self._values.pop() else: target2.push(self._values[(len(self._values) - 1)]) self._values.pop() i += 1 return target1, target2 defreverse(self): """ ------------------------------------------------------- Reverses the contents of the source stack. Use: source.reverse() ------------------------------------------------------- Returns: None ------------------------------------------------------- """ i = 0 list = [] length = len(self._values) while i < length: list.append(self._values[0]) self._values.pop(0) i += 1 i = 0 length = len(list) while i < length: self._values.append(list[(len(list) - 1)]) list.pop(len(list) - 1) i += 1 return This is for stack array
from copy import deepcopy
class Stack:
def__init__(self):
"""
-------------------------------------------------------
Initializes an is_empty stack. Data is stored in a Python list.
Use: s = Stack()
-------------------------------------------------------
Returns:
a new Stack object (Stack)
-------------------------------------------------------
"""
self._values = []
defis_empty(self):
"""
-------------------------------------------------------
Determines if the stack is empty.
Use: b = s.is_empty()
-------------------------------------------------------
Returns:
True if the stack is empty, False otherwise
-------------------------------------------------------
"""
empty = True
if len(self._values) > 0:
empty = False
return empty
defpush(self, value):
"""
-------------------------------------------------------
Pushes a copy of value onto the top of the stack.
Use: s.push(value)
-------------------------------------------------------
Parameters:
value - a data element (?)
Returns:
None
-------------------------------------------------------
"""
self._values.append(deepcopy(value))
return
defpop(self):
"""
-------------------------------------------------------
Pops and returns the top of stack. The value is removed
from the stack. Attempting to pop from an empty stack
throws an exception.
Use: value = s.pop()
-------------------------------------------------------
Returns:
value - the value at the top of the stack (?)
-------------------------------------------------------
"""
assert len(self._values) > 0, "Cannot pop from an empty stack"
value = self._values.pop()
return value
defpeek(self):
"""
-------------------------------------------------------
Returns a copy of the value at the top of the stack.
Attempting to peek at an empty stack throws an exception.
Use: value = s.peek()
-------------------------------------------------------
Returns:
value - a copy of the value at the top of the stack (?)
-------------------------------------------------------
"""
assert len(self._values) > 0, "Cannot peek at an empty stack"
value = deepcopy(self._values[-1])
return value
def__iter__(self):
"""
FOR TESTING ONLY
-------------------------------------------------------
Generates a Python iterator. Iterates through the stack
from top to bottom.
Use: for v in s:
-------------------------------------------------------
Returns:
value - the next value in the stack (?)
-------------------------------------------------------
"""
for value inself._values[::-1]:
yield value
defsplit_alt(self):
"""
-------------------------------------------------------
Splits the source stack into separate target stacks with values
alternating into the targets. At finish source stack is empty.
Use: target1, target2 = source.split_alt()
-------------------------------------------------------
Returns:
target1 - contains alternating values from source (Stack)
target2 - contains other alternating values from source (Stack)
-------------------------------------------------------
"""
i = 0
length = len(self._values)
target1 = Stack()
target2 = Stack()
while i < length:
if (i % 2) == 0:
target1.push(self._values[(len(self._values) - 1)])
self._values.pop()
else:
target2.push(self._values[(len(self._values) - 1)])
self._values.pop()
i += 1
return target1, target2
defreverse(self):
"""
-------------------------------------------------------
Reverses the contents of the source stack.
Use: source.reverse()
-------------------------------------------------------
Returns:
None
-------------------------------------------------------
"""
i = 0
list = []
length = len(self._values)
while i < length:
list.append(self._values[0])
self._values.pop(0)
i += 1
i = 0
length = len(list)
while i < length:
self._values.append(list[(len(list) - 1)])
list.pop(len(list) - 1)
i += 1
return
This is for stack array
![-
# Imports
from Stack_array import Stack
# Constants
BAL = 'is balanced'
NO_MATCH = 'mismatched brackets'
M_RIGHT = 'too many right brackets'
M_LEFT = 'too many left brackets'
def balanced (string):
Determines if a string contains has balanced brackets or not. Non-bracket
characters are ignored. Uses a Stack. Brackets include {}, [], (), ↔.
Use: is_balanced balanced (string)
Parameters:
Returns:
||||||
string the string to test (str)
=
is_balanced (string)
BALANCED if the brackets in string are is_balanced
NO_MATCH if the brackets in string are mismatched
MORE_RIGHT if there are more right brackets than left in string
MORE_LEFT if there are more left brackets than right in string
return](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffa21f7d2-4dd0-4238-8547-d147ae559015%2F8bbaa562-3df6-4df2-9d0c-9c538b50c2ba%2Fnzjjyfx_processed.png&w=3840&q=75)

Step by step
Solved in 4 steps with 2 images

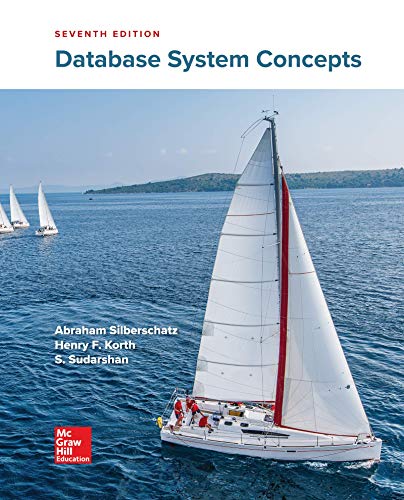
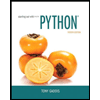
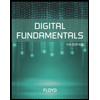
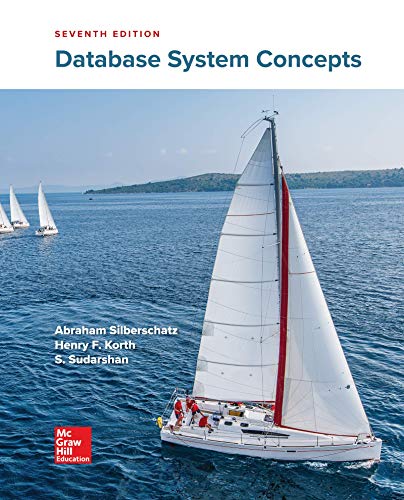
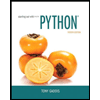
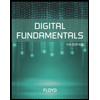
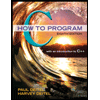
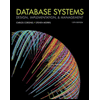
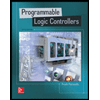