Data structure/ C language / Graph / Dijkstra’s algorithm implement a solution of a very common issue: how to get from one town to another using the shortest route. * design a solution that will let you find the shortest paths between two input points in a graph, representing cities and towns, using Dijkstra’s algorithm. Your program should allow the user to enter the input file containing information of roads connecting cities/towns. The program should then construct a graph based on the information provided from the file. The user should then be able to enter pairs of cities/towns and the algorithm should compute the shortest path between the two cities/towns entered. Attached a file containing a list of cities/towns with the following data: Field 1: Vertex ID of the 1st end of the segment Field 2: Vertex ID of the 2nd of the segment Field 3: Name of the town Field 4: Distance in Kilometer Please note that all roads are two-ways. Meaning, a record may represent both the roads from feild1 to field2 and from the road from feild2 to feild1. You are required to implement a program to help finding the shortest path between 2 points in the provided file as follows: Read the file segments.txt and load the data Enter 2 points to compute the shortest path between them Print the route of the shortest distance to a file called “route.txt” Exit
Data structure/ C language / Graph / Dijkstra’s
implement a solution of a very common issue: how
to get from one town to another using the shortest route.
* design a solution that will let you find the shortest paths between
two input points in a graph, representing cities and towns, using Dijkstra’s
algorithm. Your program should allow the user to enter the input file
containing information of roads connecting cities/towns. The program
should then construct a graph based on the information provided from the
file. The user should then be able to enter pairs of cities/towns and the
algorithm should compute the shortest path between the two cities/towns
entered.
Attached a file containing a list of cities/towns with the following data:
Field 1: Vertex ID of the 1st end of the segment
Field 2: Vertex ID of the 2nd of the segment
Field 3: Name of the town
Field 4: Distance in Kilometer
Please note that all roads are two-ways. Meaning, a record may represent
both the roads from feild1 to field2 and from the road from feild2 to feild1.
You are required to implement a program to help finding the shortest path
between 2 points in the provided file as follows: Read the file segments.txt and load the data
Enter 2 points to compute the shortest path between them
Print the route of the shortest distance to a file called “route.txt”
Exit
the file contents:
18 6 Rummana 88
13 19 Tiinnik 34
5 20 At Tayba 94
1 20 Arabbuna 43
2 5 Zububa 50
3 21 Al Jalama 88
22 19 Silat al Harithiya al 94
22 14 As Saaida 90
4 25 Anin 7
25 24 Arrana 31
26 22 Deir Ghazala 66
12 26 Faqqua 72
24 27 Khirbet Suruj 12
28 25 Al Yamun 34
30 29 Umm arRihan 4
21 30 Kafr Dan 9
31 20 Khirbet AbdallahalYunis 32
15 32 Dhaher alMalih 45
32 33 Bartaa ashSharqiya 63
34 28 Al Araqa 74
29 34 Al Jameelat 38
10 35 Beit Qad 67
37 30 Tura alGharbiya 91
38 37 Tura ashSharqiya 55
20 38 Al Hashimiya 83
39 17 Nazlat ashSheikhZeid 66
26 39 At Tarem 52
18 40 Khirbet alMuntaralGharbiya 72
27 40 Jenin 54
39 36 Jenin Camp 8
38 42 Jalbun 45
36 23 Aba 47
43 34 Khirbet Masud 78
29 43 Khirbet alMuntarashSharqiya 46
9 44 Kafr Qud 91
16 45 Deir AbuDaif 71
40 46 Birqin 67
27 47 Umm Dar 56
48 44 Al Khuljan 86
34 49 Wad adDabi 4
35 50 Dhaher alAbed 41
43 51 Zabda 90
50 48 Yabad 14
46 52 Kufeirit 84
33 53 Imreiha 51
52 53 Umm atTut 35
42 51 Ash Shuhada 6
33 54 Jalqamus 87
53 54 Al Mughayyir 19
51 55 Al Mutilla 45
54 56 Bir alBasha 33
50 59 Al Hafira 84
60 31 Qabatiya 75
57 60 Arraba 51
55 62 Telfit 32
61 62 Mirka 6
49 63 Wadi Duoq 4
62 63 Fahma alJadida 46
64 58 Raba 52
59 64 Misliya 11
63 65 Al Jarba 55
65 66 Az Zababida 43
47 67 Fahma 75
66 67 Az Zawiya 97
56 68 Kafr Rai 7
68 69 Al Kufeir 81
36 70 Sir 8
69 71 Ajja 91
59 72 Anza 83
73 64 Sanur 59
72 73 Ar Rama 61
74 72 Meithalun 48
45 74 Al Judeida 31
71 74 al Asaasa 57
75 73 Al Attara 27
72 75 Siris 52
54 76 Jaba 21
77 75 Al Fandaqumiya 71
75 78 Silat adhDhahr 98
77 78 Bardala 31
79 77 Ein elBeida 1
74 79 Kardala 31
80 77 Ibziq 35
79 80 Salhab 19
63 81 Aqqaba 58
62 83 Tayasir 95
11 84 Al Farisiya 9
60 85 Al Aqaba 75
85 83 Ath Thaghra 62
71 87 Al Malih 31
87 79 Tubas 96
88 80 Kashda 54
87 88 Khirbet Yarza 53
83 89 Ras alFara 8
83 90 El FaraCamp 13
91 26 Khirbet arRasalAhmar 23
81 92 Wadi alFara 12
89 92 Tammun 15
41 93 Khirbet Atuf 35
70 93 Khirbet Humsa 27
53 94 Akkaba 65
58 95 Qaffin 86
94 96 Nazlat Isa 6
78 97 An NazlaashSharqiya 74
84 98 Baqa ashSharqiya 21
92 99 An NazlaalWusta 26
100 98 An NazlaalGharbiya 60
92 101 Zeita 32
99 101 Seida 39
67 102 Illar 86
101 102 Attil 83
88 103 Deir alGhusun 14
104 88 Al Jarushiya 10
105 87 Al Masqufa 43
91 106 Bala 10
98 106 Iktaba 47
107 105 Nur ShamsCamp 29
102 108 Tulkarm Camp 94
109 107 Tulkarm 54
110 104 Anabta 22
111 110 Kafr alLabad 19
112 111 Kafa 31
109 112 Al Haffasi 11
96 113 Ramin 14
76 114 Farun 37
115 109 Shufa 23
116 115 Khirbet Jubara 18
117 116 Saffarin 97
118 117 Beit Lid 8
119 118 Ar Ras 91
120 109 Kafr Sur 15
114 119 Kur 85
121 120 Kafr Zibad 84
110 122 Kafr Jammal 91
123 106 Kafr Abbush 97
124 121 Bizzariya 4
60 125 Burqa 13
126 124 Yasid 87
106 127 Beit Imrin 71
104 128 Nisf Jubeil 38
99 129 Sabastiya 4
108 131 Ijnisinya 45
127 132 Talluza 26
132 133 An Naqura 22
129 134 Al Badhan 39
135 126 Deir Sharaf 65
113 136 Asira ashShamaliya 19
114 137 An Nassariya 26
137 138 Zawata 21
136 138 Al Aqrabaniya 3
138 139 Qusin 77
139 135 Beit Iba 28
140 93 Beit Hasan 18
130 140 Beit Wazan 98
132 130 Ein BeitelMaCamp 39
141 123 Ein Shibli 93
98 141 Azmut 66
131 142 Nablus 49
95 143 Askar Camp 45
144 141 Deir alHatab 77
143 100 Sarra 59
145 136 Salim 76
93 146 Balata Camp 90
123 147 Iraq Burin 73
141 147 Tell 63
148 145 Beit Dajan 22
142 148 Rujeib 7
143 144 Kafr Qallil 93
140 146 Furush BeitDajan 24
149 142 Madama 68
148 150 Burin 85
149 150 Beit Furik 89
82 151 Asira alQibliya 11
146 151 Awarta 85
102 152 Urif 13
134 152 Odala 88
78 153 Huwwara 96
103 153 Einabus 88
86 154 Yanun 51
151 154 Beita 57
155 147 Ar Rajman 18
138 156 Zeita Jammain 40
154 157 Jammain 48
153 159 Osarin 44
90 160 Aqraba 78
161 149 Zatara 51
157 162 Tall alKhashaba 45
150 163 Yatma 79
164 161 Qabalan 84
149 163 Jurish 20
162 158 Qusra 53
97 165 Talfit 33
160 166 As Sawiya 44
.....
......

Step by step
Solved in 2 steps

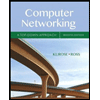
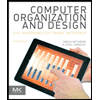
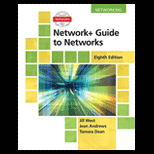
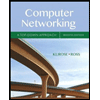
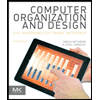
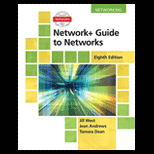
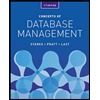
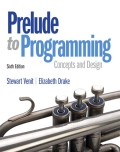
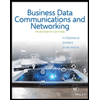