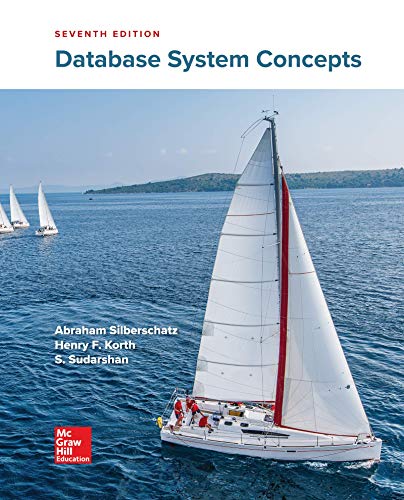
Create three files:
- Payroll class.java -Base class definition
- PayrollOvertime.jave - Derived class definition
- PayrollOvertimeClient.java - contains main() method
Implement the two user define classes with the following specifications:
Payroll class (the base class)
-
5 data fields(protected)
- String name - Initialized in default constructor to "John Doe"
- int ID - Initialized in default constructor to 9999
- doulbe payRate - Initialized in default constructor to 15.0
- doulbe hrWorked - Initialized in default constructor to 40
- static int data field named nOfObjs for tracking the number of employees, (initialized to 0 and updated nOfObjs++ in the constructors)
-
2 constructors (public)
- Default constructor (make sure to update the nOfObjs member)
- A constructor that accepts the employee’s name, ID, and pay rate as arguments. These values should be assigned to the object’s name, ID, and payRate fields, also update the nOfObjs member
-
8 public member methods (mutators, accessors, and other)
- getNOfObjts()
- getName()
- getID()
- setPayRate() & getPayRate()
- setHrWorked() & getHrWorked()
- A method named grossPay that returns employee’s gross pay (=hrWorked * payRate)
- getNOfObjts()
PayrollOvertime class (the derived class)
-
2 private fields
- double overtimePayRate- Initialized in default constructor to 22.5
- double overtimeHrWorked - Initialized in default constructor to 0
-
2 constructors (public)
- Default constructor (use super() to invoke the Payroll constructor )
- A constructor that accepts the employee’s name, ID, and pay rate, over time pay rate as arguments.
These values should be assigned to the object’s name, ID, payRate, and overtimePayRate fields. (use super() to invoke the Payroll constructor )
-
5 public member methods (mutators, accessors, and other)
- getOvertimePayRate() & setOvertimeHrWorked (double)
- getOvertimeHrWorked() & setOvertimePayRate(double)
- Override the grossPay method of Payroll class, that returns employee’s gross pay (hrWorked * payRate+ overtimePayRate *overtimeHrWorked)
ClientClass In main() method, create two PayrollOvertime objects (one use the info inputted by the user and the other use the default constructor); and print the info for each employee
Ex:
Enter the name, ID, pay rate, overtime pay rate, regular hours and overtime worked of an employee:
Linda Smith
1234
20.5
30.25
35
10
Record 1
Name: Linda Smith
ID: 1234
Regular pay rate: $ 20.5
Overtime pay rate: $ 30.25
Regular hours worked: 35.0
Overtime hours worked: 10.0
Gross pay: $1020.00
Record 2
Name: John Doe
ID: 9999
Regular pay rate: $ 15.0
Overtime pay rate: $ 22.5
Regular hours worked: 40.0
Overtime hours worked: 0.0
Gross pay: $600.00

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- fast in pyth pleasearrow_forward1. Create the main method using pythone to test the classes. #Create the class personTypefrom matplotlib.pyplot import phase_spectrum class personType: #create the class constructor def __init__(self,fName,lName): #Initialize the data members self.fName = fName self.lName = lName #Method to access def getFName(self): return self.fName def getLName(self): return self.lName #Method to manipulate the data members def setFName(self,fName): self.fName = fName def setLName(self,lName): self.lName = lName #Create the class Doctor Type inherit from personTypeclass doctorType(personType): #Create the constructor for the doctorType class def __init__(self, fName, lName,speciality="unknown"): super().__init__(fName, lName) self.speciality = speciality #Methods to access def getSpeciality(self): return self.speciality #Methods to manipulate def setSpeciality(self,spc):…arrow_forward// Declare data fields: a String named customerName, // an int named numItems, and // a double named totalCost.// Your code here... // Implement the default contructor.// Set the value of customerName to "no name"// and use zero for the other data fields.// Your code here... // Implement the overloaded constructor that// passes new values to all data fields.// Your code here... // Implement method getTotalCost to return the totalCost.// Your code here... // Implement method buyItem.//// Adds itemCost to the total cost and increments// (adds 1 to) the number of items in the cart.//// Parameter: a double itemCost indicating the cost of the item.public void buyItem(double itemCost){// Your code here... }// Implement method applyCoupon.//// Apply a coupon to the total cost of the cart.// - Normal coupon: the unit discount is subtracted ONCE// from the total cost.// - Bonus coupon: the unit discount is subtracted TWICE// from the total cost.// - HOWEVER, a bonus coupon only applies if the…arrow_forward
- 1 using static System.Console; 2 class ShapesDemo 3 { static void Main() { abstract class GeometricFigure Create an application named ShapesDemo that 4 creates several objects 5 that descend from an abstract class called 7 { GeometricFigure . Each 8 public int Height { 10 11 9 GeometricFigure get includes a height , a { 12 13 width , and an area. return height; } 14 15 Provide get and set set { 16 17 accessors for each field height = value; except area ; the area } 18 is computed and is read- } only. Include an abstract 19 public int Width 20 { 21 22 method called get ComputeArea() that { computes the area of the 23 24 return width; GeometricFigure . 25 26 27 28 29 set Next you will create three { width = value; additional classes derived from the } 30 } 31 GeometricFigure class. Name these derived classes: Rectange , 32 public int Area 33 { 34 Square , and Triangle. get 35 { 36 37 return area; } 38 } 39 40 private void CalArea() 41 { 42 43 } 44 area = Height * Width; 45 } 46arrow_forwardObjectives: Use Javadocs to document a class and methods Design and write a Java class Use fields (instance variables) Write multiple constructors Write accessor methods Write mutator methods Use the this keyword Use string concatenation Round floating point numbers (using Math.round()) Use a Scanner object Description ProduceItem class For this project, you get to design and write a ProduceItem class that stores a description, cost and weight as fields. Include both a constructor without any parameters and one with parameters for the description (String), cost (double) and weight (double) (in that order). For the constructor without parameters, set the description to an empty string, the cost to 0.0 and the weight to 0.0. Include appropriate accessor and mutator methods (and label them with comments including the terms "accessor" or "mutator"). So that the test cases compile, name the accessor methods: getDescription() getCost() getWeight() Additionally, name the mutator methods:…arrow_forwardProject 2: Cash Register Part 1: Write a class named RetailItem that holds data about an item in a retail store. The class should store the following data in attributes: item description, units in inventory, and price. Once you have written the class, write a program that creates three RetailItem objects and stores the following data in them: Description. Units in Inventory PriceItem #1 Jacket 12 59.95Item #2 Designer Jeans 40 34.95 Item #3 Shirt 20 24.95 Part 2: Create a CashRegister class that can be used with the RetailItem class. The CashRegister class should be able to internally keep a list of RetailItem objects. The class should have the following methods:• A method named purchase_item that accepts a RetailItem object as an argument. Each time the purchase_item method is called, the RetailItem object that is passed as…arrow_forward
- Create a Class called Transaction with: instance variables: stock - your stock class type - char -> b or s (buy/sell) quantity - can be fractional price - buy/sell price when - LocalDate constructors: constructor with parameters for stock, type and quantity. - sets the price from the stock price instance variable - sets when from LocalDate getters and setters for each instance variablearrow_forwardCreate three files: Student.java - Abstract Class definition ENGRStudent.java - Derived Class definition Client.java - Contains main() method (1) Implement the abstract Student class with the following specifications: 3 private members String name int ID int yearAdmitted 1 constructors (public) A constructor with that accepts the student’s name, ID, and yearAdmitted as arguments. These values should be assigned to the object’s name, ID, and yearAdmitted fields. 2 public member methods A method named toString() that returns the values of name, ID, and yearAdmitted with String data type A abstract method name getRemainingHrs with int data type (2) Implement the derived class ENGRStudent class with the following specifications: 3 final private members with the following values : int MATHSCHRS=32 int MAJOR_HRS=66 int GenEdHrs=29 3 private members: int mathScHrs; int majorHrs; int GenEdHrs; 1 constructors (public) A constructor that accepts the student’s name, ID, and yearAdmitted…arrow_forwardclass IndexItem { public: virtual int count() = 0; virtual void display()= 0; };class Book : public IndexItem { private: string title; string author; public: Book(string title, string author): title(title), author(author){} virtual int count(){ return 1; } virtual void display(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };class Category: public IndexItem { private: /* fill in the private member variables for the Category class below */ ? int count; public: Category(string name, string code): name(name), code(code){} /* Implement the count function below. Consider the use of the function as depicted in main() */ ? /* Implement the add function which fills the category with contents below. Consider the use of the function as depicted in main() */ ? virtualvoiddisplay(){ /* YOU DO NOT NEED TO IMPLEMENT THIS FUNCTION */ } };arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
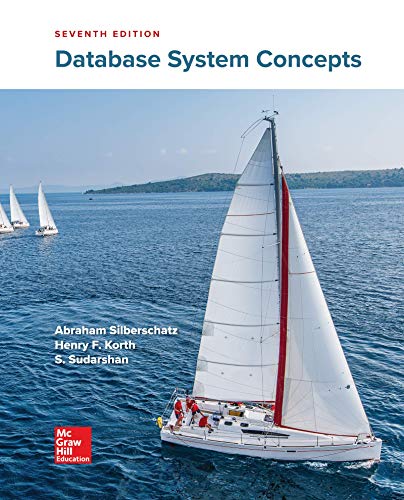
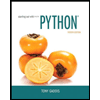
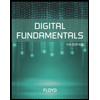
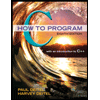
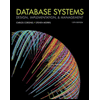
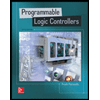