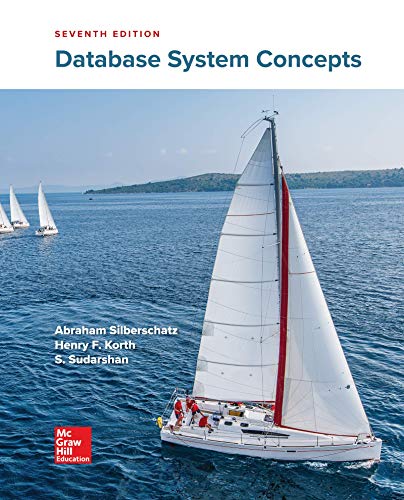
Java
Overview
In this task, you will try to create a class called Course and a test class that creates objects of the Course class. The purpose is to teach you to write classes, create objects of classes and call public methods on the created object.
Task
Write a class that represents a course given here at San University. The class must contain information about the course course code, name, number of credits and a description.
This should be stored as instance variables in the class. Use English names for all variables and methods. All instance variables must use the String class as the data type. The instance variables may only be accessible within the own class.
It must be possible to set new values for all instance variables in the class. This must be implemented with public set methods for all instance variables. There should also be a method that prints all information about the course on the screen in a "nice" and structured way.
You must also write a "test class" that creates two different courses (objects of the Course class). For one object, you must "hardcode" values directly in the test class. Ex: java.setName ("Java I");
For the second object, ask the user for the course data. This should be done using the JOptionPane class and the showInputDialog method. Data returned from showInputDialog must be saved in local variables in the main method and then sent as arguments to other object's set methods.
Along with this assignment, you must submit a Word document (or equivalent) where you have drawn a class diagram (UML) over the Course class. Name the document uml.docx (or equivalent)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 5 images

- python code: 1.Create an empty class called Car. 2.Create a method called initialize in the class, which takes a name as input and saves it as a data attribute. 3.Create an instance of the class, and save it in a variable called car. 4.Call the initialize method with the string “Ford".arrow_forwardJava code Write the header for a public method named doStuff that has no parameters and no return value. Remember to include the opening brace for the method. Assume that the method will be in the main class and called directly by the main method.arrow_forwardHelp me With this Java Taskarrow_forward
- CLASS NAME List the instance variables in the constructor. List the methods in the class. - indicates private CLASS NAME List the instance variables in the constructor. List the methods in the class. The second row of the UML diagram lists the instance variables in the constructor. For example -name: string o o name is the variable name o string is the data type of name + indicates public o getName is the method name o o string is the return data type Java.util. The third row of the diagram lists the methods in the class. For example, +getName(): string empty parenthesie indicate nothing is sent to the method.arrow_forwardCreate a function in Python named sumListDiv2 which takes as input a list of integers and returns the sum of the elements of the list which are divided by 2. Don't forget to add the type contract as comments.arrow_forwardHelp me solve this in Java, don't make it to complicated.arrow_forward
- Objectives By completing this assignment students should be able to: • Write programs using classes Instructions Write a program called passport.cpp. You also need to create a class to hold the passport itself. This class should be broken into .epp and .h files as we have done in class. The license class should hold the following information about a license: • The passport number • The first and last name of the person • Their nationality • Their birthdate You will then write a program that will prompt for information about a person's passport and then print out that license to the command line. Sample Output what is the person's first name: James What is the person's last name: Bond what is the passport number: 28128555 what is the person's nationality: UK What is the person's birthdate: 01/01/1970 UK Passport: 28128555 James Bond Born 01/01/1970arrow_forwardProblem Description and Given Info For this assignment you are given the following Java source code files: IStack.java (This file is complete – make no changes to this file) MyStack.java (You must complete this file) Main.java (You may use this file to write code to test your MyStack) You must complete the public class named MyStack.java with fields and methods as defined below. Your MyStack.java will implement the IStack interface that is provided in the IStack.java file. You must implement your MyStack class as either a linked list or an array list (refer to your MyArrayList and MyLinkedList work). Your MyStack must not be arbitrarily limited to any fixed size at run-time. UML UML CLass Diagram: MyStack Structure of the Fields While there are no required fields for your MyStack class, you will need to decide what fields to implement. This decision will be largely based on your choice to implement this MyStack as either an array list or a linked list. Structure of the Methods As…arrow_forwardPortfolio Instructions: You are working for a financial advisor who creates portfolios of financial securities for his clients. A portfolio is a conglomeration of various financial assets, such as stocks and bonds, that together create a balanced collection of investments. When the financial advisor makes a purchase of securities on behalf of a client, a single transaction can include multiple shares of stock or multiple bonds. It is your job to create an object-oriented application that will allow the financial advisor to maintain the portfolios for his/her clients. You will need to create several classes to maintain this information: Security, Stock, Bond, Portfolio, and Date. The characteristics of stocks and bonds in a portfolio are shown below: Stocks: Bonds: Purchase date (Date) Purchase date (Date) Purchase price (double)…arrow_forward
- PLEASE help with the following C#.NET using the following class make a driver class which outputs the patient health record Write a driver class (app) that prompts for the person’s data input, instantiates an object of class HeartRates and displays the patient’s information from that object by calling the DisplayPatientRecord, method. essentially make a main method which asks the user to manually enter the data using System;namespace A1Question2{public class HealthProfile{ // attibutes which holds the following valueprivate String _FirstName;private String _LastName;private int _BirthYear;private double _Height;private double _Weigth;private int _CurrentYear;public HealthProfile(string firstName, string lastName, int birthYear, double height, double wt, int currentYear){_FirstName = firstName;_LastName = lastName;_BirthYear = birthYear;_Height = height;_Weigth = wt;_CurrentYear = currentYear;}public string firstName { get; set; }public string lastName { get; set; }public int birthYear…arrow_forwardUSE BEGINNER LEVEL JAVA!!!! BASIC JAVA!!!arrow_forwardtrue of false: a) The first step in any programming task is to start coding. b) The only places we would ever want to put a comment in our code are above a method and above a class.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
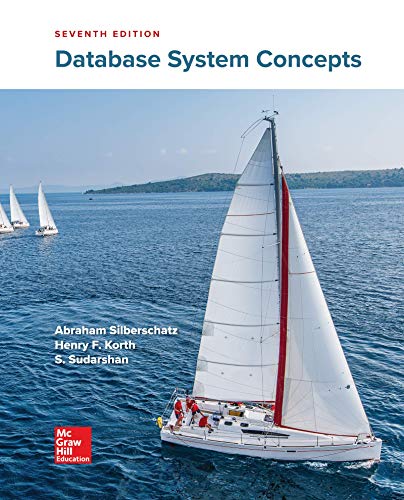
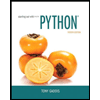
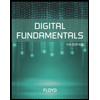
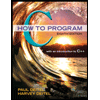
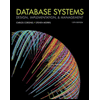
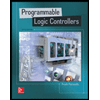