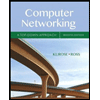
Hospital Employee
Hospital Java
//********************************************************************
// Hospital.java Authors: Lewis/Loftus
//
// Solution to Programming Project 9.2
//********************************************************************
public class Hospital
{
//-----------------------------------------------------------------
// Creates several objects from classes derived from
// HospitalEmployee.
//-----------------------------------------------------------------
public static void main(String[] args)
{
HospitalEmployee vito = new HospitalEmployee("Vito", 123);
Doctor michael = new Doctor("Michael", 234, "Heart");
Nurse sonny = new Nurse("Sonny", 789, 6);
// print the employees
System.out.println(vito);
System.out.println(michael);
System.out.println(sonny);
// invoke the specific methods of the objects
vito.work();
michael.diagnose();
sonny.assist();
}
}
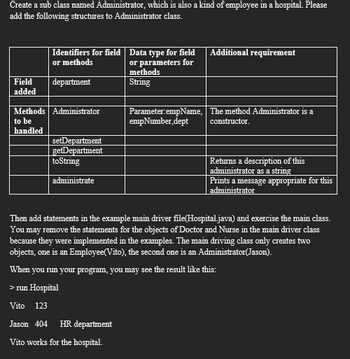
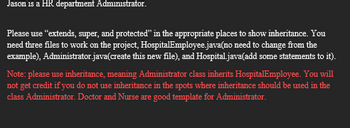

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- 29 JAVA JAVA onlyarrow_forwardJava Programming Please help me with this Java Progamming project NOT GRADED. Practice homework Please comment the code well and organize the classes so I can understand. Thank you! If solution if the one that is desirable, I will upvote! I really appreciate this, Bartleby!arrow_forwardimport java.util.Arrays; import java.util.Random; public class Board { /** * Construct a puzzle board by beginning with a solved board and then * making a number of random moves. That some of the possible moves * don't actually change what the board looks like. * * @param moves the number of moves to make when generating the board. */ public Board(int moves) { throw new RuntimeException("Not implemented"); } The board is 5 X 5. You can add classes and imports like rand.arrow_forward
- using java maze1.txt (file being referenced) 4 4*************.....*******.***.....***.***.*******.**....*****.**.**.*****....**.....***.*********..........*************arrow_forwardJava programming: What's the output of the following program... a.) Compilation Error b.) 97~B~C~D~E~102~G~ c.) 65~b~C~d~E~70~G~ d.) a~66~C~68~E~f~G~arrow_forwardJavaarrow_forward
- Java. Code: public class Person{// PLEASE START YOUR CODE HERE// *********************************************************// *********************************************************// PLEASE END YOUR CODE HERE//constructorpublic Person(String firstName, String lastName){this.firstName = firstName;this.lastName = lastName;}public String toString(){return firstName + " " + lastName;}}arrow_forwardBAGEL FILES import javax.swing.JFrame; public class Bagel{//-----------------------------------------------------------------// Creates and displays the controls for a bagel shop.//-----------------------------------------------------------------public static void main (String[] args){JFrame frame = new JFrame ("Bagel Shop");frame.setDefaultCloseOperation (JFrame.EXIT_ON_CLOSE); frame.getContentPane().add(new BagelControls()); frame.pack();frame.setVisible(true);}} import java.awt.*;import java.awt.event.*; import javax.swing.*; public class BagelControls extends JPanel{private JComboBox bagelCombo;private JButton calcButton;private JLabel cost;private double bagelCost;public BagelControls(){String[] types = {"Make A Selection...", "Plain","Asiago Cheese", "Cranberry"};bagelCombo = new JComboBox(types);calcButton = new JButton("Calc");cost = new JLabel("Cost = " + bagelCost);setPreferredSize (new Dimension (400,…arrow_forwardUsing p5* JavaScript on "openprocessing" websitearrow_forward
- //********************************************************************//EvenOdd.java Java Foundations////Demonstrates the use of the JOptionPane class.//******************************************************************** import javax.swing.JOptionPane; public class EvenOdd{//-----------------------------------------------------------------// Determines if the value input by the user is even or odd.// Uses multiple dialog boxes for user interaction.//-----------------------------------------------------------------public static void main (String[] args){String numStr, result;int num, again; do {numStr = JOptionPane.showInputDialog ("Enter an integer: "); num = Integer.parseInt(numStr); result = "That number is " + ((num%2 == 0) ? "even" : "odd"); JOptionPane.showMessageDialog (null, result); again = JOptionPane.showConfirmDialog (null, "Do Another?");}while (again == JOptionPane.YES_OPTION);System.out.println("here");}}arrow_forwardwrite a code in java (using recursion)arrow_forwardJAVA PROGRAM ASAP ************* THE PROGRAM MUST WORK IN HYPERGRADE AND PASS ALL THE TEST CASES.**************** Chapter 16. PC #5. Palindrome Detector (page 1073) A palindrome is any word, phrase, or sentence that reads the same forward and backward. Here are some well-known palindromes: Able was I, ere I saw Elba A man, a plan, a canal, Panama Desserts, I stressed Kayak Write a boolean method that uses recursion to determine whether a String argument is a palindrome. The method should return true if the argument reads the same forward and backward. Demonstrate the method in a program. The program should ask the user to enter a string, which is checked for palindrome property. The program displays whether the given input is a palindrome or not, then prompts the user to enter another string. If the user enters QUIT (case insensitive, then exit the program). Test Case 1 Please enter a string to test for palindrome or type QUIT to exit:\nDesserts, I…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
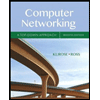
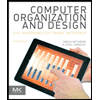
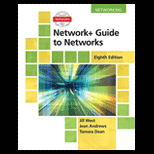
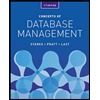
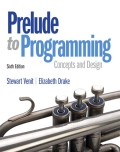
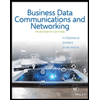