Create a new javascript file called cart.js and make a product object for each of your products and store it in a products array when the script loads. Create an empty array to store the items added to the cart. Program the "add to cart button" to take the product id, finds the product object in the products array and adds it to the items added to cart array. Program the "remove from cart button" to take the product id and remove the project object from the items purchased array.
Create a new javascript file called cart.js and make a product object for each of your products and store it in a products array when the script loads. Create an empty array to store the items added to the cart.
Program the "add to cart button" to take the product id, finds the product object in the products array and adds it to the items added to cart array.
Program the "remove from cart button" to take the product id and remove the project object from the items purchased array.
Existing code:
<!DOCTYPE html>
<html>
<head>
<div id="product1" class="card">
<img src="img/clorox.jpg">
<h1>Clorox</h1>
<p class="price">$20.00</p>
<p>Household Cleaner</p>
<p><button id="product1Btn1">Add to Cart</button></p>
<p><button id="product1Btn2">Remove from Cart</button></p>
</div>
<div id="product2" class="card">
<img src="img/oxiclean.jpg">
<h1>Oxiclean</h1>
<p class="price">$8.00</p>
<p>Household Cleaner</p>
<p><button id="product2Btn1">Add to Cart</button></p>
<p><button id="product2Btn2">Remove from Cart</button></p>
</div>
<div id="product3" class="card">
<img src="img/pinesol.jpg">
<h1>PineSol</h1>
<p class="price">$11.00</p>
<p>Household Cleaner</p>
<p><button id="product3Btn1">Add to Cart</button></p>
<p><button id="product3Btn2">Remove from Cart</button></p>
</div>
<div id="product4" class="card">
<img src="img/mrclean.jpg">
<h1>Mr Clean</h1>
<p class="price">$12.00</p>
<p>Household Cleaner</p>
<p><button id="product4Btn1">Add to Cart</button></p>
<p><button id="product4Btn2">Remove from Cart</button></p>
</div>
<div id="product5" class="card">
<img src="img/windex.jpg">
<h1>Windex</h1>
<p class="price">$15.00</p>
<p>Household Cleaner</p>
<p><button id="product5Btn1">Add to Cart</button></p>
<p><button id="product5Btn2">Remove from Cart</button></p>
</div>
</head>
</html>

Step by step
Solved in 3 steps with 1 images

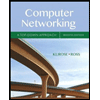
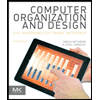
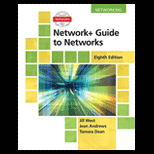
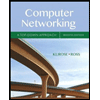
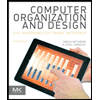
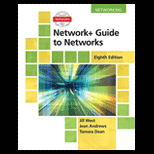
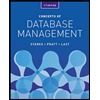
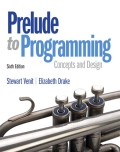
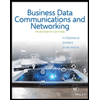