Assignment For assignments 1 and 2, your Big-O notation and justification will be written in the HTML section of JSFiddle. You will be using the Javascript array Programming Assignment Part 1 – Using your JSFiddle account and JavaScript, you will create a program that will instantiate an integer array of a specified size. This means you must create a text box interface and a button “Create Array”. Put a default value of 100 in the Text Box. When the user clicks “Create Array” create an array and fill each array element with a random integer between 1 and 100. You will need to research the random function to do this (the JavaScript function is Math.random()). This array should be a global variable. You will next need an interface (same JSFiddle) with a second and third Text Box and button. The button will say “Insert into Array”. It will trigger a function that will read a number from the second Text Box and insert it into the array at the index of the third Text Box. This will be done by calling a function as specified below. The name of the function will be InsertIntoArray and it will have 3 arguments (function InsertIntoArray(array, index, value)). Argument 1 is the array, argument 2 is the index of where you are going to insert a new number, argument 3 is the number to insert. The program should insert the new number at the index given and shift all the previous entries index up by 1. The highest element of the array will be deleted. Count the number of operations performed on the array and output this to the screen. An operation is if you assign a value or compare a value – only compare or assign operations should be counted. Change the array size to 100 and insert into a different index in the array. State using Big O Notation your time complexity and be prepared to justify your answer. Programming parameters: 1. Create the array in Javascript; 2. Create a loop to assign each array element a random value. 3. Do not use push or splice commands – assign elements by writing the code to perform all operations 4. When inserting you will be pushing the last value off the end of the array (everything shifts right) 5. Use a global counter to count every time you make an assignment (every time you use an = or ==operator). 6. Output the count. Programming Assignment Part 2 – Using the same JSFiddle, we will add one more button and Text Box. The button will be “Search”. Clicking the button will search the array and output the first index where the value entered in the Text Box is found. This will be done by calling a function SearchArray (function SearchArray(array, value)). The name of the function will be SearchArray. Argument 1 is the array, argument 2 is the value you are searching for within the array. Each time the program compares two numbers you must count it as an operation (only count comparisons). Output the total number of operations to find the first occurrence of the number searched or value not found. State using Big O Notation your time complexity and be prepared to justify your answer. This should be output in your actual JSFiddle. Remember: You also need to put in the time complexity. This is NOT HARD – This is not a trick question; you will give a simple Big O notation of the time complexity of the search and the insertion on your HTML page. ( example Complexity: O ___ Information: There should be 4 TextBoxes – I recommend naming them (id = “name”) tbArraySize, tbInsertValue, tbInsertIndex, tbSearchValue. You can use your own naming scheme – but it is important you have a naming scheme. You will have three buttons; “Create Array”, “Insert Into Array”, “Search Array” I recommend naming them btnCreate, btnInsert, and btnSearch. Sample Output
Assignment
For assignments 1 and 2, your Big-O notation and justification will be written in the HTML section of JSFiddle. You will be using the Javascript array
Programming Assignment Part 1 –
Using your JSFiddle account and JavaScript, you will create a program that will instantiate an integer array of a specified size. This means you must create a text box interface and a button “Create Array”. Put a default value of 100 in the Text Box. When the user clicks “Create Array” create an array and fill each array element with a random integer between 1 and 100. You will need to research the random function to do this (the JavaScript function is Math.random()). This array should be a global variable.
You will next need an interface (same JSFiddle) with a second and third Text Box and button. The button will say “Insert into Array”. It will trigger a function that will read a number from the second Text Box and insert it into the array at the index of the third Text Box. This will be done by calling a function as specified below.
The name of the function will be InsertIntoArray and it will have 3 arguments (function InsertIntoArray(array, index, value)). Argument 1 is the array, argument 2 is the index of where you are going to insert a new number, argument 3 is the number to insert. The program should insert the new number at the index given and shift all the previous entries index up by 1. The highest element of the array will be deleted. Count the number of operations performed on the array and output this to the screen. An operation is if you assign a value or compare a value – only compare or assign operations should be counted.
Change the array size to 100 and insert into a different index in the array. State using Big O Notation your time complexity and be prepared to justify your answer.
Programming parameters:
1. Create the array in Javascript;
2. Create a loop to assign each array element a random value.
3. Do not use push or splice commands – assign elements by writing the code to perform all operations
4. When inserting you will be pushing the last value off the end of the array (everything shifts right)
5. Use a global counter to count every time you make an assignment (every time you use an = or ==operator).
6. Output the count.
Programming Assignment Part 2 – Using the same JSFiddle, we will add one more button and Text Box. The button will be “Search”. Clicking the button will search the array and output the first index where the value entered in the Text Box is found. This will be done by calling a function SearchArray (function SearchArray(array, value)).
The name of the function will be SearchArray. Argument 1 is the array, argument 2 is the value you are searching for within the array. Each time the program compares two numbers you must count it as an operation (only count comparisons). Output the total number of operations to find the first occurrence of the number searched or value not found. State using Big O Notation your time complexity and be prepared to justify your answer. This should be output in your actual JSFiddle.
Remember: You also need to put in the time complexity. This is NOT HARD – This is not a trick question; you will give a simple Big O notation of the time complexity of the search and the insertion on your HTML page. ( example
Complexity: O ___
Information:
There should be 4 TextBoxes – I recommend naming them (id = “name”) tbArraySize, tbInsertValue, tbInsertIndex, tbSearchValue. You can use your own naming scheme – but it is important you have a naming scheme. You will have three buttons; “Create Array”, “Insert Into Array”, “Search Array” I recommend naming them btnCreate, btnInsert, and btnSearch.
Sample Output


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

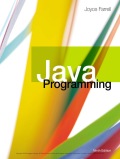
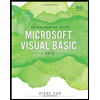
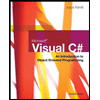
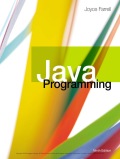
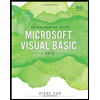
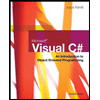