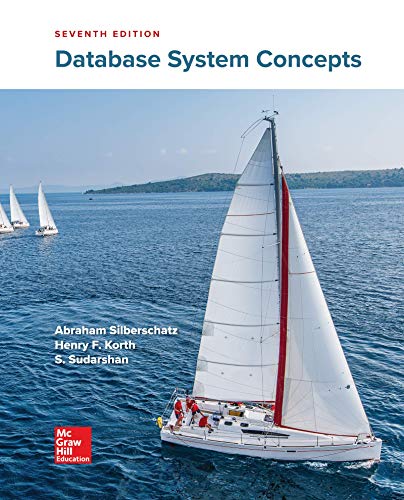
Concept explainers
Create a method multiply(), that receives the 2D array you created in the program below as as an input, multiplies all of its elements and displays the product.
Hint: your method won’t return anything
import java.util.*;
public class Two_D_Ragged {
public static void Using_array_class(int R_array[][]) {
System.out.println("");
System.out.println("using Arrays class Through the Ragged Array");
//Array.toString method is used to show array elements in R_array[0]
System.out.println(Arrays.toString(R_array[0]));
//Array.toString method is used to show array elements in R_array[1]
System.out.println(Arrays.toString(R_array[1]));
//Array.toString method is used to show array elements in R_array[2]
System.out.println(Arrays.toString(R_array[2]));
} //end of Using_array_class method
public static void Using_for_each(int R_array[][]) {
System.out.println("for-each-loop Through the Ragged Array");
int k = 0;
//prints elements in R_array[0]
for (int i:R_array[0]) {
System.out.print(R_array[0][k] +" ");
k++;
}
System.out.println("");
int l = 0;
//prints elements in R_array[1]
for (int i:R_array[1]) {
System.out.print(R_array[1][l] +" ");
l++;
}
System.out.println("");
int m = 0;
//prints elements in R_array[2]
for (int i:R_array[2]) {
System.out.print(R_array[2][m] +" ");
m++;
}
} //end of Using_for_each method
public static void Using_for_loop(int R_array[][]) {
System.out.println("for-loop Through the Ragged Array");
for (int i = 0; i < R_array.length; i++) {
for (int j = 0; j < R_array[i].length; j++) {
System.out.print(R_array[i][j]+ " ");
}
System.out.println("");
}
}
public static void main(String[] args) {
int[][] R_array = new int [3][];
R_array[0] = new int[]{10, 20, 30};
R_array[1] = new int[]{40, 50};
R_array[2] = new int[]{60};
Using_for_loop(R_array);//calling method to print array elements using for loop
Using_for_each(R_array); //calling method to print array elements using for-each loop
Using_array_class(R_array); //calling method to print array elements using Array class
}//end of main method
}//end of Two_D_Ragged class

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Please help with this java problemarrow_forwardpublic class arrayOutput ( public static void main (String [] args) { final int NUM ELEMENTS = 3; int[] userVals = new int [NUM_ELEMENTS]; int i; } Type the program's output userVals [0] = 2; userVals [1] = 6; userVals [2] = 8; for (i = userVals.length - 1; i >= 0; −−1) { System.out.println(userVals [1]); } C.C. ? ? ??arrow_forwardWrite a Java method (do not write a class, only the method) with the following signature: Return type: void Parameters: 2D integer array Name: printDiagonal Implement the method code that will print out the values of the 2D array along the Diagonal from left to right. For example, if the array had the following values: 56 78 98 21 33 55 98 44 11 The method should print to the console: 56,33,11 The above 2D array example is only given for you to understand the problem Do not hard code the values in your solution or assume the array is the same as the given data. Your method should work for all 2D integer arrays.arrow_forward
- Javaarrow_forwardJAVA PROGRAM Write a program that asks the user to input two integers a and b. Then create an array that has all integer from a to b. Print out the array. (Don’t forget to consider the situation of a=b, a<b, and a>b.) Example 1: Input two numbers: 6 2 Array is [6, 5, 4, 3, 2] Example 2: Input two numbers: 4 8 Array is [4, 5, 6, 7, 8] Pass the above array as a parameter to a method called average and return the average and print it out in the main method. (Do not use Math library to get the average.)arrow_forwardJavaarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
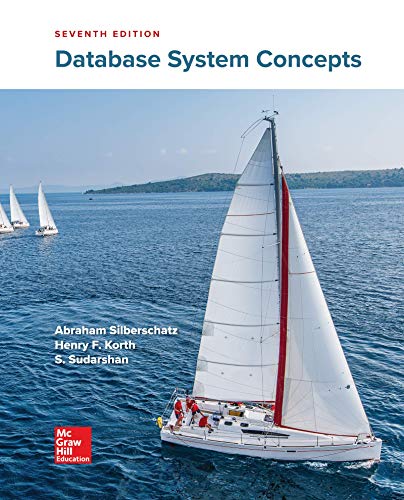
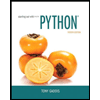
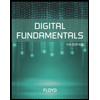
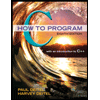
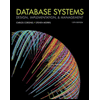
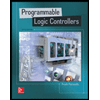