using System; public static class Lab8 { public static void Main() { int[] compStats = new int[25]; int n = 0, large, small; double avg = 0; // Input values into the array InpArray(compStats, ref n); // Find the average of the elements in the array // *** Insert the code for the call to the FindAverage method // Find the largest element in the array // *** Insert the code for the call to the FindLarge method // Find the largest element in the array // *** Insert the code of the call to the FindSmall method // Print out the results Console.WriteLine("\nThe Average of the array is {0:F}", avg); // *** Insert the code to print out the largest and smallest values // Pause until user is done Console.ReadLine(); } // Method: InpArray // Description: Input values into an array. // Parameters: arrValues: the array to fill. // num: number of elements in the array. // Returns: void public static void InpArray(int[] arrValues, ref int num) { // input the number of data values to put in the array do { Console.Write("Enter the number of elements (<= 25) => "); num = Convert.ToInt32(Console.ReadLine()); } while (num < 0 || num > 25); // loop to enter the values for (int i = 0; i < num; ++i) { Console.Write("Enter the Element {0} => ", i); arrValues[i] = Convert.ToInt32(Console.ReadLine()); } } // Method: FindAverage // Description: Computes the average of the elements in the array. // Parameters: arrVals: array to be processed // num: number of elements in the array. // Returns: the average of the elements in the array public static double FindAverage(int[] arrVals, int num) { // *** Insert the details of the FindAverage Method } // Method: FindLarge // Description: Determines the largest element in the array. // Parameters: arrVals: array to be processed // num: number of elements in the array. // Returns: the largest element in the array public static int FindLarge(int[] arrVals, int num) { // *** Insert the details of the FindLarge Method //**Do not use built in C# array functions (like Sort or Max) to do this, it should be done using iteration. */ } // Method: FindSmall // Description: Determines the smallest element in the array. // Parameters: arrVals: array to be processed // num: number of elements in the array. // Returns: the smallest element in the array public static int FindSmall(int[] arrVals, int num) { // *** Insert the details of the FindSmall Method //**Do not use built in C# array functions (like Sort or Max) to do this, it should be done using iteration. */ } }
using System;
public static class Lab8
{
public static void Main()
{
int[] compStats = new int[25];
int n = 0, large, small;
double avg = 0;
// Input values into the array
InpArray(compStats, ref n);
// Find the average of the elements in the array
// *** Insert the code for the call to the FindAverage method
// Find the largest element in the array
// *** Insert the code for the call to the FindLarge method
// Find the largest element in the array
// *** Insert the code of the call to the FindSmall method
// Print out the results
Console.WriteLine("\nThe Average of the array is {0:F}", avg);
// *** Insert the code to print out the largest and smallest values
// Pause until user is done
Console.ReadLine();
}
// Method: InpArray
// Description: Input values into an array.
// Parameters: arrValues: the array to fill.
// num: number of elements in the array.
// Returns: void
public static void InpArray(int[] arrValues, ref int num)
{
// input the number of data values to put in the array
do
{
Console.Write("Enter the number of elements (<= 25) => ");
num = Convert.ToInt32(Console.ReadLine());
} while (num < 0 || num > 25);
// loop to enter the values
for (int i = 0; i < num; ++i)
{
Console.Write("Enter the Element {0} => ", i);
arrValues[i] = Convert.ToInt32(Console.ReadLine());
}
}
// Method: FindAverage
// Description: Computes the average of the elements in the array.
// Parameters: arrVals: array to be processed
// num: number of elements in the array.
// Returns: the average of the elements in the array
public static double FindAverage(int[] arrVals, int num)
{
// *** Insert the details of the FindAverage Method
}
// Method: FindLarge
// Description: Determines the largest element in the array.
// Parameters: arrVals: array to be processed
// num: number of elements in the array.
// Returns: the largest element in the array
public static int FindLarge(int[] arrVals, int num)
{
// *** Insert the details of the FindLarge Method
//**Do not use built in C# array functions (like Sort or Max) to do this, it should be done using iteration. */
}
// Method: FindSmall
// Description: Determines the smallest element in the array.
// Parameters: arrVals: array to be processed
// num: number of elements in the array.
// Returns: the smallest element in the array
public static int FindSmall(int[] arrVals, int num)
{
// *** Insert the details of the FindSmall Method
//**Do not use built in C# array functions (like Sort or Max) to do this, it should be done using iteration. */
}
}


Step by step
Solved in 2 steps with 1 images

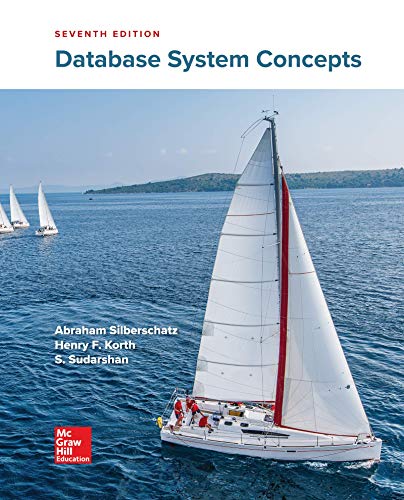
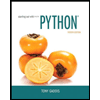
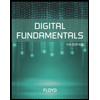
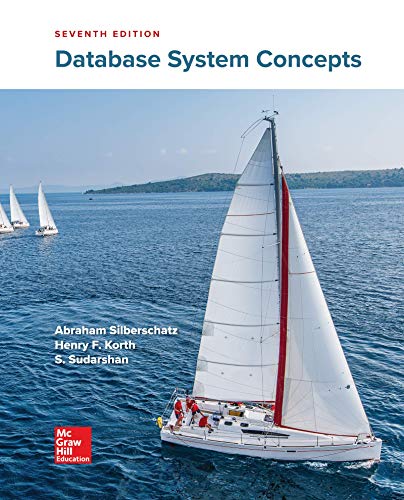
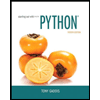
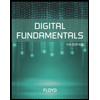
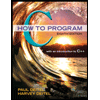
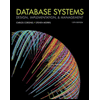
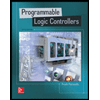