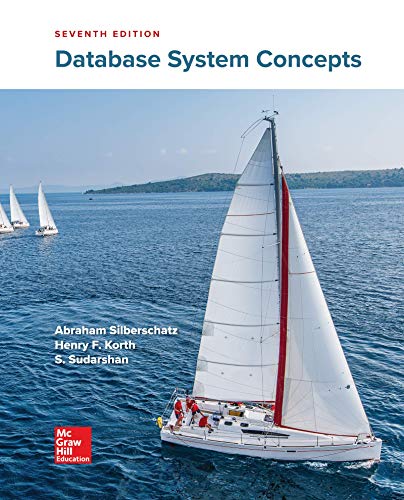
Create a java program with a class called Address. An address has the following instance variables:
- address
- address2
- city
- state
- postal code
All of these instance variables are strings.
Create the getters and setters.
Create a default, no argument constructor.
Supply two non-default constructors that accepts the following:
- all the address components
- all address components except address2
Accept the constructor parameters in the order above.
Supply a print method that returns a String representing the address on one line.
Clarification: All components of the Address should print - however, address2 should only print if one is provided. Your method should not print null if no address2 is provided.
The address class needs to only accept two characters for the state. Also only five digits can be entered for the postalCode (no less than 5, no more than 5).
Modify both of your constructors in your address class to throw an IllegalArgumentException if state or postal code is null.
In the AddressTester class, test that the exception works. Change your tester class back to working after you've tested the exception.
The Program NEEDS to be complete and able to run with the tester code provided below.
class AddressTest {
Address home = new Address("163 Main Street", "Denver", "CO","73521");
Address condo = new Address("5075 Turner Hill", "Apt 2B","Nashville", "TN", "37115");
@Test
void testInstanceVariables() {
assertNull(home.getAddress2());
assertNotNull(condo.getAddress2());
assertEquals("CO", home.getState());
assertEquals("37115", condo.getPostalCode());
}
@Test
void testPrint() {
assertEquals("163 Main Street Denver, CO 73521", home.print());
assertEquals("5075 Turner Hill Apt 2B Nashville, TN 37115", condo.print());
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Design a class named CustomerRecord that holds a customer number, name, and address. Include separate methods to 'set' the value for each data field using the parameter being passed. Include separate methods to 'get' the value for each data field, i.e. "return" the field's value. Pseudocode:arrow_forwardWhat is the purpose of a constructor? It creates an array of data values associated with an object. It prints all of the information about our object. It returns all data members from an object back to the main method. It allows us to set our own default values when we create an object. in javaarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
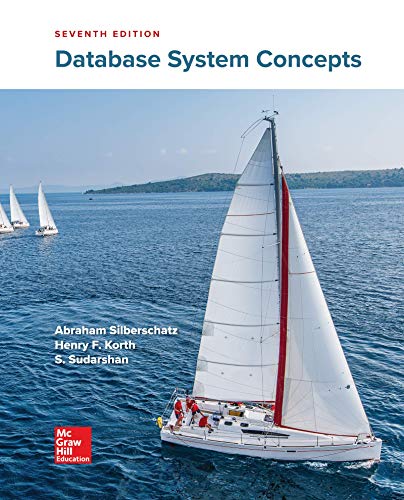
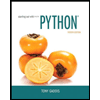
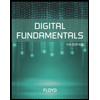
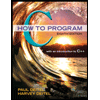
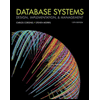
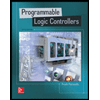