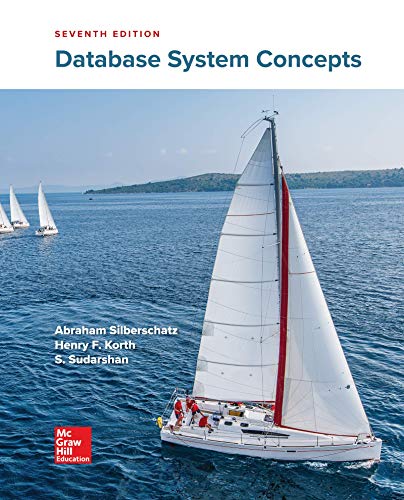
Create a graphical JAVA program that simulates a simple arcade game (like Frogger) using the ACM graphical package.
an example is written below.
import acm.program.*;
import java.awt.Color;
import acm.graphics.*;
import java.util.Random;
import java.awt.event.KeyEvent;
public class Frogger extends GraphicsProgram {
GLabel gover = new GLabel("GAME OVER ", 50, 60);
GImage[] car = { new GImage("car.png", 0, 145), new GImage("car.png", 200, 145), new GImage("car.png", 400, 145) };
GImage frog = new GImage("frogsmall.png", 200, 325);
public static void main(String[] args) {
Frogger game = new Frogger();
game.start();
}
public void drawImage() {
for (int i = 0; i < car.length; i++) {
add(car[i]);
}
add(frog);
}
public void run() {
setBackground(Color.GREEN);
// tell program to listen for keyboard events
addKeyListeners();
//set up game over label
gover.setColor(Color.RED);
gover.setFont("Arial-30");
drawRoad();
drawRoadMarkers();
drawImage();
while (true) {
// for every car in the array
for (GImage c : car) {
// move car
c.move(50, 0);
// if car goes off right side of screen,
// place back on left
if (c.getX() > getWidth()) {
c.setLocation(0, 145);
}
// if car runs over frog
if (c.getBounds().intersects(frog.getBounds())) {
// make frog invisible
frog.setVisible(false);
//stop car from moving
c.move(0, 0);
// add Game Over label
add(gover);
}
// pause so human eye can see
pause(50);
}
}
}
public void drawRoad() {
GRect road = new GRect(0, 140, 900, 180);
road.setFilled(true);
road.setColor(Color.BLACK);
add(road);
}
public void drawRoadMarkers() {
for (int x = 10; x < getWidth(); x += 50) {
GRect line = new GRect(x, 225, 25, 5);
line.setFilled(true);
line.setColor(Color.YELLOW);
add(line);
}
}
// if up down right or left arrow is pressed move frog
public void keyPressed(KeyEvent event) {
if (event.getKeyCode() == KeyEvent.VK_UP)
frog.move(0, -10);
else if (event.getKeyCode() == KeyEvent.VK_DOWN)
frog.move(0, 10);
else if (event.getKeyCode() == KeyEvent.VK_LEFT)
frog.move(-10, 0);
else if (event.getKeyCode() == KeyEvent.VK_RIGHT)
frog.move(10, 0);
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- I need help with this Java Problem as described in the image below: public class Person { private int ageYears; private String lastName; public void setName(String userName) { lastName = userName; } public void setAge(int numYears) { ageYears = numYears; } // Other parts omitted public void printAll() { System.out.print("Name: " + lastName); System.out.print(", Age: " + ageYears); }}// ===== end ===== // ===== Code from file Student.java =====public class Student extends Person { private int idNum; public void setID(int studentId) { idNum = studentId; } public int getID() { return idNum; }}// ===== end ===== // ===== Code from file StudentDerivationFromPerson.java =====public class StudentDerivationFromPerson { public static void main(String[] args) { Student courseStudent = new Student(); /* Your solution goes here */ }}arrow_forwardAnswer the prompt with given main method: Code: import java.awt.*; public class TestRandomWalker { public static final int STEPS = 500; public static void main(String[] args) { RandomWalker walker = new RandomWalker(); // instantiate Walker object DrawingPanel panel = new DrawingPanel(500, 500); Graphics g = panel.getGraphics(); // advanced features -- center and zoom in the image panel.getGraphics().translate(250, 250); panel.getGraphics().scale(4, 4); // make the walker walk, and draw its movement int prevX = walker.getX(); // initialize Walker display int prevY = walker.getY(); for (int i = 1; i <= STEPS; i++) { g.setColor(Color.BLACK); g.drawLine(prevX, prevY, walker.getX(), walker.getY()); // update Walker display walker.move();…arrow_forwardHi, can you please help me out with these?arrow_forward
- Question & instructions can be found in images please see images first Starter code import javax.swing.*;import java.awt.*;import java.awt.event.*;import java.text.DecimalFormat; public class GPACalculator extends JFrame{private JLabel gradeL, unitsL, emptyL; private JTextField gradeTF, unitsTF; private JButton addB, gpaB, resetB, exitB; private AddButtonHandler abHandler;private GPAButtonHandler cbHandler;private ResetButtonHandler rbHandler;private ExitButtonHandler ebHandler; private static final int WIDTH = 400;private static final int HEIGHT = 150; private static double totalUnits = 0.0; // total units takenprivate static double gradePoints = 0.0; // total grade points from those unitsprivate static double totalGPA = 0.0; // total GPA (gradePoints / totalUnits) //Constructorpublic GPACalculator(){//Create labelsgradeL = new JLabel("Enter your grade: ", SwingConstants.RIGHT);unitsL = new JLabel("Enter number of units: ", SwingConstants.RIGHT);emptyL = new…arrow_forwardWrite in Java a code give out what is on the image. Essentially write in the main function so that it prints what is in the image. Im stuck at that part it would be much appreciated if you could help. package javaapplication31; import java.util.Random;import java.util.Scanner;/**** @author */public class JavaApplication31 {String name;/*** @param args the command line arguments*/public static void main(String[] args) {int totalScore = 300;int itrcount =12;int reward;char direction;int x = 0;int y = 0;System.out.println(reward());System.out.println(inputDirection());}public static void displayInfo(int x, int y, int itrCount, int totalScore){x = 0;y = 0;itrCount = 0;totalScore =300;System.out.println("");}public static boolean doesExceed (int x,int y, char direction){return (y > 4 && Character.toLowerCase(direction) == 'u' || x < 0 && Character.toLowerCase(direction) == '1'||y < 0 && Character.toLowerCase(direction) == 'd' || x > 4 &&…arrow_forwardPlease help me with this. I am really struggling Please help me modify my java matching with the image provide below or at least fix the errors import java.awt.*; import java.awt.event.*; import javax.swing.*; public class Game extends JFrame implements ActionListener { int hallo[][] = {{4,6,2}, {1,4,3}, {5,5,1}, {2,3,6}}; int rows = 4; int cols = 3; JButton pics[] = new JButton[rows*cols]; public Game() { setSize(600,600); JPanel grid = new JPanel(new GridLayout(rows,cols)); int m = 0; for(int i = 0; iarrow_forward
- Hi I want to create a JAVA program that will allow me to create a canvas (specifically in eclipse). Can someone straighten my code import java.awt.*;import javax.swing.*;class Main extends JFrame { // constructor Main() { super("canvas"); // create a empty canvas Main c = new Main() { // paint the canvas public void paint(Graphics g) { // set color to red g.setColor(Color.red); // set Font g.setFont(new Font("Bold", 1, 20)); // draw a string g.drawString("This is a canvas", 100, 100); } }; // set background c.setBackground(Color.black); add(c); setSize(400, 300); show(); } // Main Method public static void main(String args[]) { Main c = new Main(); }} ReplyForwardarrow_forwardJAVA CODE: check outputarrow_forwardPlease make the code do what the description says.arrow_forward
- Java Question- Convert this source code into a GUI application using JOptionPane. Make sure the output looks similar to the following picture. Thank you. import java.util.Calendar;import java.util.TimeZone;public class lab11_5{public static void main(String[] args){//menuSystem.out.println("-----------------");System.out.println("(A)laska Time");System.out.println("(C)entral Time");System.out.println("(E)astern Time");System.out.println("(H)awaii Time");System.out.println("(M)ountain Time");System.out.println("(P)acific Time");System.out.println("-----------------"); System.out.print("Enter the time zone option [A-P]: "); //inputString tz = System.console().readLine();tz = tz.toUpperCase(); //change to uppercase //get current date and timeCalendar cal = Calendar.getInstance();TimeZone.setDefault(TimeZone.getTimeZone("GMT"));System.out.println("GMT/UTC:\t" + cal.getTime()); switch (tz){case "A":tz =…arrow_forwardIn Java import java.util.Scanner;public class Image {int numberOfPhotos; // photos on rolldouble fStop; // light let it 1.4,2.0,2.8 ... 16.0int iso; // sensativity to light 100,200, 600int filterNumber; // 1-6String subjectMatter;String color; // black and white or colorString location;boolean isblurry;public String looksBlurry(boolean key){if ( key == true){return "Photo is Blurry";}else{return "Photo is Clear";}}public void printPhotoDetails (String s1){Scanner br= new Scanner(System.in);String subjectMatter=s1;System.out.println("Data of Nature photos:");System.out.println("Enter number of photos:");numberOfPhotos= br.nextInt();int i=1;while(true){System.out.println("Enter Filter number of photos"+i+":");filterNumber= br.nextInt();System.out.println("Enter colour of photo"+i+ ":");String color= br.next();System.out.println("Enter focal length of photo"+i+":");fStop= br.nextInt();System.out.println("Enter location of photo"+i+":");String location= br.next();System.out.println("Enter…arrow_forwardIn Java code: Write the class encapsulating the concept of money, assuming that money has the following attributes: dollars, cents In addition to the constructors, the accessors and mutators, write the following methods: public Money() public Money(int dollars, int cents) public Money add(Money m) public Money substract(Money m) public Money multiply(int m) public static Money[] multiply(Money[] moneys, int amt) public boolean equals(Money money) public String toString() private void normalize() // normalize dollars and cents field Add additional helper methods if necessary. Use the following test driver program to test your Money class: public class MoneyTester{public static void main(String[] args){Money m1 = new Money(8, 75); // set dollars to 8 and cents to 75Money m2 = new Money(5, 80); // set dollars to 5 and cents to 80 MoneyMoney m3 = new Money(); // initialize dollars to 0 and cents to 0System.out.println("\tJane Doe " + "CIS35A Spring 2021 Lab 4"); // useyour…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
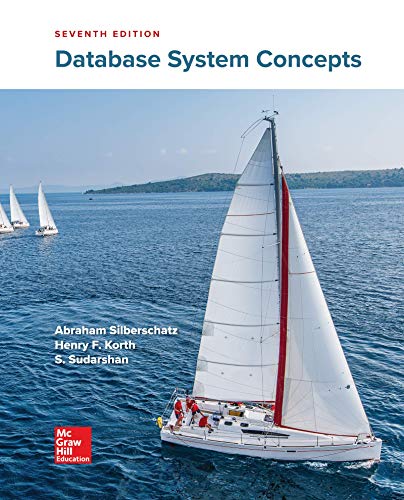
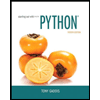
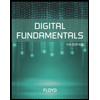
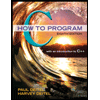
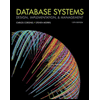
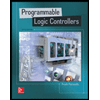