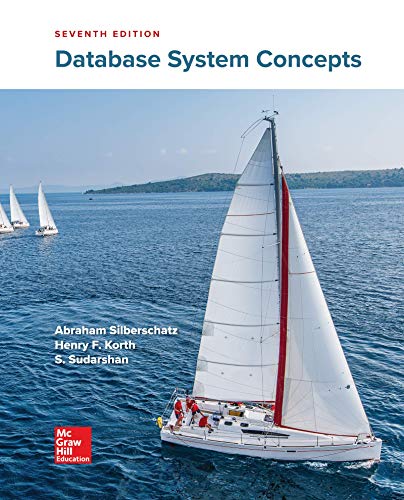
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Create a graph class using an adjacency list from scratch in Java. Can not use the util library
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- without using java's sort methods, nodes, linked list from the standary library. build a sorted java ADT implemented with a double link then build a Abstract data type manager phone book with sorted ADT list implementationarrow_forwardimport java.util.HashMap; import java.util.Map; public class LinearSearchMap { // Define a method that takes in a map and a target value as parameters public static boolean linearSearch(Map<String, Integer> map, int target) { // Iterate through each entry in the map for () { // Check if the value of the current entry is equal to the target if () { // If the value is equal to the target, return true } } // If no entry with the target value is found, return false } public static void main(String[] args) { // Create a HashMap of strings and integers Map<String, Integer> numbers = new HashMap<>(); // Populate the HashMap with key-value pairs numbers.put("One", 1); numbers.put("Two", 2); numbers.put("Three", 3); numbers.put("Four", 4); numbers.put("Five", 5); // Set the target value to search for…arrow_forwardDo the complete version of the user-defined Tree interface for the code given below (meaning you need to add the code for all methods that were not coded and have a "Left as an exercise" comment). import java.util.Collection; public interface Tree<E> extends Collection<E> { /** Return true if the element is in the tree */ public boolean search(E e); /** Insert element e into the binary tree * Return true if the element is inserted successfully */ public boolean insert(E e); /** Delete the specified element from the tree * Return true if the element is deleted successfully */ public boolean delete(E e); /** Get the number of elements in the tree */ public int getSize(); /** Inorder traversal from the root*/ public default void inorder() { } /** Postorder traversal from the root */ public default void postorder() { } /** Preorder traversal from the root */ public default void preorder() { } @Override /** Return true if the tree is empty */ public default…arrow_forward
- Use a SinglyLinked List to implement a Queuea. Define a Queue interface.b. Define a LinkedQueue class (Node class)..c. Define a Test class to test all methods please help me make a test class first code package LinkedQueue; import linkedstack.Node; public class SLinkedList<E> { private Node <E> head = null; private Node <E> tail = null; private int size = 0; public SLinkedList() { head = null; tail = null; size = 0; } public int getSize() { return size; } public boolean isEmpty() { return size == 0; } public E getFirst() { if(isEmpty()) { return null; } return head.getElement(); } public E getLast() { if(isEmpty()) { return null; } return tail.getElement(); } public void addFirst(E e) { Node<E> newest = new Node<>(e, null); newest.setNext(head); head = newest; if(getSize()==0) { tail = head; } size++; } public void addLast(E e) { Node<E> newest = new Node<>(e, null); if(isEmpty()) { head = newest; } else {…arrow_forwardComplete using Java import java.util.Scanner; import java.util.ArrayList; public class SET { ArrayListlist; int length; public SET(); { list=new ArrayList<>(10); length=0; } public SET(int cap) { list=new arrayList<>(cap); length=0; } public int getLength() { return length; } } public class SetApplication { public static void main(String[] args) { SET st=new SET(); System.out.println(st.getLength()); } }arrow_forwardAdd missing code to turn this class a singleton in Java package com.gamingroom; import java.util.ArrayList;import java.util.List; /** * A singleton service for the game engine */public class GameService { /** * A list of the active games */ private static List<Game> games = new ArrayList<Game>(); /* * Holds the next game identifier */ private static long nextGameId = 1; // FIXME: Add missing pieces to turn this class a singleton /** * Construct a new game instance * * @param name the unique name of the game * @return the game instance (new or existing) */ public Game addGame(String name) { // a local game instance Game game = null; // FIXME: Use iterator to look for existing game with same name // if found, simply return the existing instance // if not found, make a new game instance and add to list of games if (game == null) { game = new Game(nextGameId++, name); games.add(game); } // return the new/existing game instance to the caller return…arrow_forward
- Draw a class diagram that shows how to use JCF class ArrayList, LinkedList, and Iterator using a UML drawing tool.arrow_forwardUse a SinglyLinked List to implement a Queuea. Define a Queue interface.b. Define a LinkedQueue class (Node class)..c. Define a Test class to test all methods help me make a test class first code package LinkedQueue; import linkedstack.Node; public class SLinkedList<E> { private Node <E> head = null; private Node <E> tail = null; private int size = 0; public SLinkedList() { head = null; tail = null; size = 0; } public int getSize() { return size; } public boolean isEmpty() { return size == 0; } public E getFirst() { if(isEmpty()) { return null; } return head.getElement(); } public E getLast() { if(isEmpty()) { return null; } return tail.getElement(); } public void addFirst(E e) { Node<E> newest = new Node<>(e, null); newest.setNext(head); head = newest; if(getSize()==0) { tail = head; } size++; } public void addLast(E e) { Node<E> newest = new Node<>(e, null); if(isEmpty()) { head = newest; } else {…arrow_forwardI am having trouble determining time complexity when it comes to finding the runtime of a method or function. I need to know what the runtime for the following methods are. Any further explanation would also be helpful. All of these methods are dealing with a binary search tree in java. 1. public String getAllValues() - return a String that contains the elementsof the tree in ascending order. All elements are separated by a space. 2. public int numberNodes() - return the total number of nodes in the tree. 3. public void insertList(int[] list) - insert all of the values from list intothe tree. Note: My Method just goes through an array calling working insert() method on all the values in the list[] array. 4. public int removeLessThan(int value) - this method removes all integers strictly less than value from the tree. It also returns the number of nodes itremoved. Note: In this method I first call a method to count nodes less than I call another method to recursively remove by…arrow_forward
- Write a LISP function, outdegree, of two arguments, a node and the graph, which returns the outdegree of the node. (the outdegree of a node, k, is the number of arcs coming out of the node, i.e. # of arcs that have the node k as a source). The graph is represented by the adjacency list representation.arrow_forwardI need help on writing a interface using python Tkinter where a user can draw graph data structure. It should look like a typical graph with circles for vertices and straight edges betweenthe vertices. The graph should be stored in a Python dictionary. Please help. Only Python tkinter is allowed to usearrow_forwardConsider the instance variables and constructors. Given Instance Variables and Constructors: public class SimpleLinkedList<E> implements SimpleList<E>, Iterable<E>, Serializable { // First Node of the List private Node<E> first; // Last Node of the List private Node<E> last; // Number of List Elements private int count; // Total Number of Modifications (Add and Remove Calls) private int modCount; /** * Creates an empty SimpleLinkedList. */ publicSimpleLinkedList(){ first = null; last = null; count = 0; modCount = 0; } ... Assume the class contains the following methods that work correctly: public boolean isEmpty() public int size() public boolean add(E e) public E get(int index) private void validateIndex(int index, int end) Complete the following methods based on the given information from above. /** * Adds an element to the list at the…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
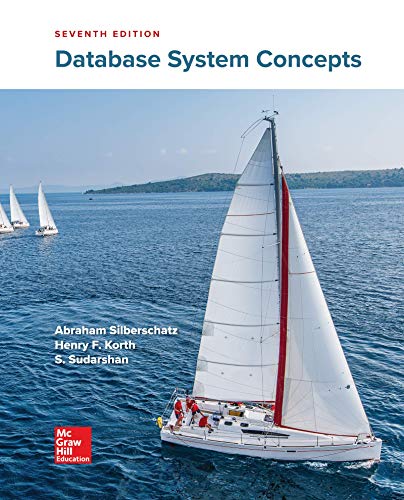
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
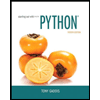
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
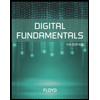
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
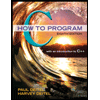
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
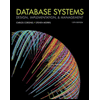
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
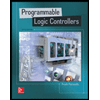
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education