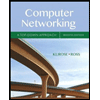
Assume the <iostream>, <fstream>, and <string> headers have already been included and that main() looks like this:
(picture 1)
Each row of the capitals.txt file contains the following data, in this order:
- The city (string, one word)
- The state (string, one word)
- The population (integer)
Here are the exact contents of the "capitals.txt" file to copy:
Phoenix Arizona 1660272
Austin Texas 964254
Ohio Columbus 892553
Indianapolis Indiana 867125
Denver Colorado 716492
Boston Massachusetts 694583
Nashville Tennesseee 635710
Sacramento California 466488
Atlanta Georgia 420003
Honolulu Hawaii 359870
Create a function named smallestCapital as follows:
The function returns an integer.
The function has 5 parameters:
Two string arrays
One integer array
An ifstream object
An integer for the length of the arrays
The function should be coded as follows:
If the ifstream object did not open the file, return a value of -1.
Otherwise, use a while loop to read data from the file and store each data value into its corresponding array. The ifstream object must be used in the loop condition.
Use any type of loop to find the index of the smallest population and return it.
Rules:
Submit only the code you are asked to write. You do not have to create a main program or a function prototype.
The code you submit must still follow all C++ syntax rules and compile.
You must use the exact type of loop specified in each part that requires a loop.
The test conditions for all loops must contain a boolean expression with a comparison operator (==, <=, >=, and so on).
No break or continue statements are allowed.
You may create as many local variables in the function as you believe are needed.
will upvote!
![int main ()
ifstream inFile;
inFile.open ("capitals.txt");
string city[10];
string state[10];
int population[10];
int here = smallestCapital (city, state, population, inFile, 10);
if (here == -1) (
cout << "File did not open." << endl;
} else {
cout <« "Smallest state capital is " <« city [here] << ", " <« state [here] << " with population " << population [here] << endl;
}
inFile.close () ;
return 0;
}](https://content.bartleby.com/qna-images/question/ad3ee529-81b1-486a-8459-b4eea2f68af5/111bc444-d2b6-42c4-acfc-5c7499015110/si7bknl_thumbnail.png)

Step by stepSolved in 2 steps with 2 images

- 2.7 LAB - Alter Movie table The Movie table has the following columns: ID - integer, primary key Title - variable-length string Genre - variable-length string RatingCode - variable-length string Year - integer Write ALTER statements to make the following modifications to the Movie table: Add a Producer column with VARCHAR data type (max 50 chars). Remove the Genre column. Change the Year column's name to ReleaseYear, and change the data type to SMALLINT.arrow_forwardR-Studio 1.) You have an airport routes.csv file that contains 3409 airports, each row has a 3-letter code and the number of routes. Example: Airport NumberOfRoutes AAE 9 AAL 20 AAN 2 AAQ 3 AAR 8 AAT 2 AAX 1 AAY 1 ABA 4 ABB 2 ABD 6 ABE 13 ABI 2 ABJ 49 ABL 4 ABM 1 ABQ 42 ABR 1 ABS 1 ABT 3 ABV 30 ABX 4 ABY 4 ABZ 41 ACA 8 ACC 54 ACE 116 ACH 2 ACI 2 ACK 6 ACR 2 ACT 2 ACU 1 ACV 3 ACX 6 ACY 10 ACZ 1 ADA 17 ADB 66 ADD 105 ADE 23 ADF 2 ADK 1 ADL 51 ADQ 11 ADU 3 ADZ 11 AEB 3 AEP 65 For this dataset, consider the following models:(a) Suppose the given data points follow a power law distribution. Estimate the corresponding α parameter. (b) Suppose the given data points follow an exponential distribution. Estimate the corresponding λ parameter. (c) Suppose the given data points follow a uniform distribution. Estimate the corresponding range parameters [a, b] of the uniform distribution.(d)…arrow_forwardCreate a new file cart.html You must get and set the data on the web page by using document.getElementById( ). Each HTML element on the web page already has an id so it is easy. No need for a loop to go through the list of products, there is only 2 products and the info is hard coded on the web page. You must write the code for function cartTotal().arrow_forward
- Write the code segments based on the given descriptions: Declare a file pointer called input. Open a file called location.txt for reading using this pointer. If the file is not available, display “File does not exist.”. The content of the text file location.txt is as shown below. It includes the location, latitude and longitude values. FILE location.txt CONTENTS <Location> <Latitude> <Longitude> UTM 1.5523763 103.63322 KLCC 3.153889 101.71333 UM 3.1185 101.665 UMS 6.0367 116.1186 UNIMAS 1.465174 110.4270601 Ask the user to enter a location. Check if the user’s location can be found in the text file. If found, display the location, latitude and longitude as shown below. SAMPLE OUTPUT Enter a location: UM Location : UM Latitude : 3.1185 Longitude : 101.6650 Otherwise display “Sorry, location could not be found”. SAMPLE OUTPUT Enter a location : MMU Sorry, location could not be foundarrow_forwardIn pythonarrow_forwardCode in C language. Please provide code for 3 different files. One header file and two .C files. I need the code for each file. Please provide photos of the output and source code for each file (3). Please follow All instructions in the photo provided. Use the provided input.txt file provided below. A1, A2 20294 Lorenzana Dr Woodland Hills, CA 91364 B1, B2 19831 Henshaw St Culver City, CA 94023 C1, C2 5142 Dumont Pl Azusa, CA 91112 D1, D2 20636 De Forest St Woodland Hills, CA 91364 A1, A2 20294 Lorenzana Dr Woodland Hills, CA 91364 E1, E2 4851 Poe Ave Woodland Hills, CA 91364 F1, F2 20225 Lorenzana Dr Los Angeles, CA 91111 G1, G2 20253 Lorenzana Dr Los Angeles, CA 90005 H1, H2 5241 Del Moreno Dr Los Angeles, CA 91110 I1, I2 5332 Felice Pl Stevenson Ranch, CA 94135 J1, J2 5135 Quakertown Ave Thousand Oaks, CA 91362 K1, K2 720 Eucalyptus Ave 105 Inglewood, CA 89030 L1, L2 5021 Dumont Pl Woodland Hills, CA 91364 M1, M2 4819 Quedo Pl Westlake Village, CA 91362 I1, I2 5332 Felice Pl…arrow_forward
- Computer Science Please use go language programma to write this code. Write a program that creates a CSV file to store and retrieve data such as name, age, and salary. You should be able to read the data out of the file and display the data sorted by name. Hint: Make use of the Sort package to sort the data. Make sure you check the existence of the file, closing the file when there is an error Use defer, panic, and recover as needed.arrow_forwardPlease use C++ code and only use the libraries <iostream> and <fstream>. Thank you!arrow_forwardDescriptionWrite a program to compute average grades for a course. The course records are in a single file and are organized according to the following format: each line contains a student's first name, then one space, then the student's last name, then one space, then some number of quiz scores that, if they exist, are separated by one space. Each student will have zero to ten scores, and each score is an integer not greater than 100. Your program will read data from this file and write its output to a second file. The date in the output file will be nearly the same as the data in the input file except that you will print the names as last-name, first-name; each quiz score, and there will be one additional number at the end of each line:the average of the student's ten quiz scores.Both files are parameters. You can access the name of the input file with argv[1]. and the name of the output file with argv[2].The output file must be formatted as described below: 1. First and last names…arrow_forward
- python: def txt_to_csv(input_file): """ Question 3 - cats.txt contains information about cat breeds and their various characteristics. - Read the cats.txt with FILE I/O. - These are TAB separated values - Use a list of lists to create a CSV file with the appropriate data WITHOUT WHITESPACE (remove \t) named "cleaned_cats.csv". - Exclude the "body_type" column - If a row has a length < 5, remove it. - For strings containing extra quotes, strip the extra quotes. - Return the list of lists. Args: input_file (cats.txt) Returns: List of lists Output: [['breed', 'type', 'coat_type', 'coat_pattern'], ['Abyssinian', 'Natural', 'Short', 'Ticked tabby'], ['Aegean', 'Natural', 'Semi-long', 'Multi-color'], ['American Bobtail', 'Mutation', 'Semi-long', 'All'], ['American Curl', 'Mutation', 'Semi-long', 'All'], ['American Ringtail', 'Mutation', 'Semi-long', 'All'], ['American Shorthair', 'Natural', 'Short', 'All'], ...…arrow_forwardhere is a text file containing the details of several invoices details. Each invoice uses 3 lines of the file. The first of the three lines is a string giving the date of the invoice, for example “1/5/2021” or “2/6/2021”. The second of the three lines contains double giving the amount, the third line is boolean indicating whether it is paid or not. However, the very first line of the file is an integer number, which says how many invoices are given in the file in the lines which follow it (i.e. how many records will follow). Text file example for two vehicles “invoices.txt” 2 1/5/2021 50.0 true 2/6/2021 20.0 false Write code for a method named processTextFile() which will open the file named ‘invoices.txt’, from which it will read the data of invoices. It will create Invoice objects using this data by calling the constructor that takes the parameters (date, amount, paid) , placing them into an ArrayList invoiceList.arrow_forwardCODE SHOULD BE PYTHON:arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
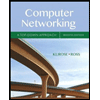
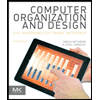
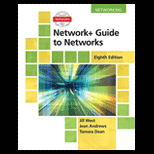
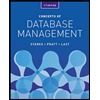
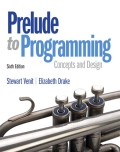
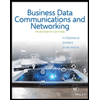