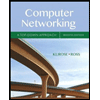
Create a dynamic array that contains an int array. dynamic array, array inside when the existing array is full and a new element needs to be added will double its size, make all the elements of the old array the same will copy the indexes and add the element to the new array. Use the following functions. Write in C language.
1.append(dynamic array, new element): function sent to itself Adds the element sturct to the end of the array inside DynamicArray. Place If not, it behaves in accordance with the dynamic array definition given above.
2. get(index): The element in the index sent to it by the function takes it from the array it hosts and sends it back.
Do not use link lists. Solve the question using array.

Step by stepSolved in 2 steps with 1 images

- C++arrow_forwardRandomize_it Write a function in C++ that takes an array as a parameter and then randomize that array, i.e. shuffles the position of the elements in random order. To randomize the array your function should perform random 100 swaps of any two elements in the array. Return the randomized array from the function.vector<int> randomize(vector<int> input){// code here}arrow_forwardC PROGRAMMING Instructions: Using the code template below, create a function that computes the average of all the elements in the array using a 'for' loop to sum the array. Use an array pointer to get the values from the array. The average is the return value from the function. Then, implement the function into the main() method. /*_____________________________________________________________________________*/ #include <stdio.h>#include <stdlib.h>int main() { float data[] = {2.5, 3.33, 4.2, 8.0, 5.1}; float sum = 0; printf("Index\tValues\n"); for(int i = 0; i < 5; i++) { printf("%d\t%f", i, data[i]); printf("\n"); sum = sum + data[i]; } sum = sum / 5; printf("Avg\t%f", sum); return 0;}arrow_forward
- Trying to write a gravity function in C languagearrow_forwardC++arrow_forwardIn C Programming: You will need all the functions from the previous assignment, as well as the structure course:• Department (string, 15 characters)• Course number (integer, 4 digits, leading 0 if necessary)• Course title (string, 30 characters)• Credits (short, 1 digit) Write a function saveAllCoursesText() which receives an array of course pointers and the array’s size, then outputs the content of the array in text format to a file named “courses.txt”.• Save the entire structure on 1 line (all the members of the structure should be saved in 1 fprintf() command)arrow_forward
- Help now please Pythonarrow_forwardWrite in C++ Sam is making a list of his favorite Pokemon. However, he changes his mind a lot. Help Sam write a function insertAfter() that takes five parameters and inserts the name of a Pokemon right after a specific index. Function specifications Name: insertAfter() Parameters (Your function should accept these parameters IN THIS ORDER): input_strings string: The array containing strings num_elements int: The number of elements that are currently stored in the array arr_size int: The number of elements that can be stored in the array index int: The location to insert a new string. Note that the new string should be inserted after this location. string_to_insert string: The new string to be inserted into the array Return Value: bool: true: If the string is successfully inserted into the array false: If the array is full If the index value exceeds the size of the arrayarrow_forwardExercise 1: //The max function the max between a and b, it returns a if a == //b public double max(double a, double b); //The mult function returns the result of a * b public double mult(double a, double b); //The exist in array function returns the index of the element //‘a’ if //‘a’ exist in array ‘arr’ otherwise returns ‘-1’ public int existsInArray(int [] arr, int a); //Are array equals method compares two arrays and returns true // if the elements of array ‘a’ are equal to elements of array // ‘b’, element by element. If equals it returns 0, it returns - // 1 if not public int areArrayEquals(int [] a, int [] b); Devise four executable test cases for every method in the JUnit notation. See the attached handout for a refresher on the notation.arrow_forward
- C++ PROGRAMMING Create a C++ program that accepts integer inputs into an array. Arrange the arrays into an ascending manner and display the missing elements in the arithmetic sequence. The number of repeating elements must be counted as well. There must be at least two user-defined functions in your program. Example 1: Input: 3, 4, 5, 1, 3, 7 Output Missing Numbers: 2, 6 Repeating: 1 Example 2: Input: 6, 1, 2, 20, 1, 2 Output Missing Numbers: 3, 4, 5, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19arrow_forwardUse C++arrow_forwardA c++ application that takes five user inputs and stores them in an array before passing them to a function. Finding the smallest integer in an array is accomplished through the use of pointers.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
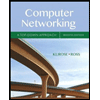
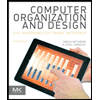
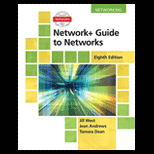
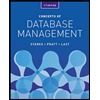
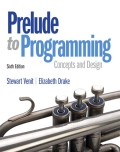
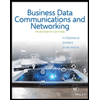