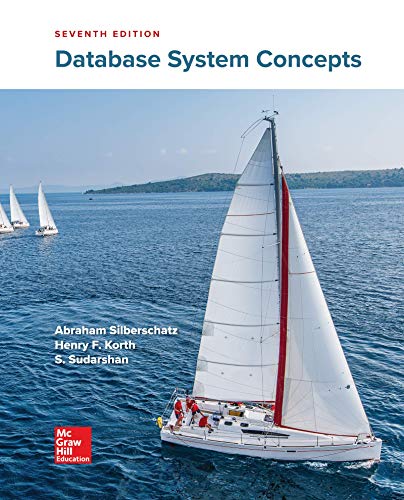
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Write a program that declares/intializes two arrays as described in main() and has two functions.
main() - The size of both arrays is 5. The first array has elements of type double. The values are for distance measurements. Choose a descriptive name for this array.The second array will have the unit of measure. Choose a descriptive name for this array. Initialize the first 4 elements using an initialization list with the values "mi", "mi", "km", "km".
Assign a new value to the first element of the second array: "km"Assign a value to the last element of the second array: "mi"
Use a loop with statement that ask the user to type in all of the values for the temperatures and assign them to the first array.
Function calls in main() -- you might want to write the definitions first (or at least read about them) before coming back to main() to write the calls. call the function that prints the measurements along with the unit of measure.Call the function that can count the number of times a string appears in an array. Use "mi" as one of the arguments so that the function returns the number of times "mi" is in the array. From main(), print the value.
Function definitions:Write a function that prints the measurements using a loop: it'll be values from both arrays so that it prints the number from one array followed by the unit of measure from the other array followed by a new line. Repeat until all of the values are printed from both arrays in that order. The function returns no values.
Write a function that can count the number of times a string appears in an array and return the count. The function takes an array of string, its size and the string it is keeping a count of. Declare a local variable called count (you might want to initialize it). Write a loop that goes through the array parameter. In the loop, write an if statement: if the element is equal to the string it is counting, increment the count variable. Return a value if necessary.
C++
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- The function list_combination takes two lists as arguments, list_one and list_two. Return from the function the combination of both lists - that is, a single list such that it alternately takes elements from both lists starting from list_one. You can assume that both list_one and list_two will be of equal lengths. As an example if list_one = [1,3,5] and if list_two = [2,4,6]. The function must return [1,2,3,4,5,6].arrow_forwardIn C++, write a program that reads in an array of type int. You may assume that there are fewer than 20 entries in the array. The output must be a two-column list. The first column is a list of the distinct array elements and the second column is the count of the number of occurences of each element.arrow_forward1. Write a program that defines a struct named Info. That Info struct should define two strings, a double and array of strings. In your main program, define a variable v using that Info struct and then initialize it to hold your first name, your last name, your lucky non-whole number (e.g. 26.5), your major, and a listing (an array) of your six most favorite college courses you have ever taken or plan to take. Then your program should access and print all the stored data held in the variable v. 2. print the data twice, once using the structure variable and do-while loop, and next using a pointer variable and a while-do loop.) Using the embed icon shown above show screenshots demoing the execution of your program.arrow_forward
- Regex, APIs, BeautifulSoup: python import requests, refrom pprint import pprintfrom bs4 import BeautifulSoup complete the missing bodies of the functions below: def mathmatic(target):"""Question 1You are doing fun math problems. Given a string of combination of '{}', '[]','()', you are required to return the substring included by the outermost '{}'.You need to write your code in one line.Args:target (str): the string to search inReturns:str>>> mathmatic('{[[[[]()]]]}')'[[[[]()]]]'>>> mathmatic('[(){([{}])}]')'([{}])'"""pass test code: # print(mathmatic('{[[[[]()]]]}'))# print(mathmatic('[(){([{}])}]'))arrow_forwardWrite a function called find_duplicates which accepts one list as a parameter. This function needs to sort through the list passed to it and find values that are duplicated in the list. The duplicated values should be compiled into another list which the function will return. No matter how many times a word is duplicated, it should only be added once to the duplicates list. NB: Only write the function. Do not call it. For example: Test Result random_words = ("remember","snakes","nappy","rough","dusty","judicious","brainy","shop","light","straw","quickest", "adventurous","yielding","grandiose","replace","fat","wipe","happy","brainy","shop","light","straw", "quickest","adventurous","yielding","grandiose","motion","gaudy","precede","medical","park","flowers", "noiseless","blade","hanging","whistle","event","slip") print(find_duplicates(sorted(random_words))) ['adventurous', 'brainy', 'grandiose', 'light', 'quickest', 'shop', 'straw', 'yielding']…arrow_forwardWrite a function called get_palindromes() that takes a list of words as input. The function should return a new list containing every word in the input list that is a palindrome (a palindrome is a word that reads the same forwards and backwards, like "noon" or "eve"). The output list should be a list of tuples, where each tuple consists of a palindrome and its length. NOTE: You must use a list comprehension to solve this exercise. Your function must be defined as a *single line* of code - CodeRunner will count the number of "new lines" inside your function definition, and there must be only one new line (separating the function header from the function body). The code editor below should show only two lines: one for the function header and one for the function body. Delete any blank lines within the function definition and do not add a new line at the end of the function body (i.e. at the end of the return statement)! For example: Test words = ["noon", "bob", "ann"] result =…arrow_forward
- Write a program with two functions: addToList(food): printList(food): Print the entire list food is used to pass a list to the function. Ask the user to input another food item. The main program will create a list: myList["apple", "oralge", "grapes] Call the function printList, using myList Call the function addToList, using myList Call the function printList, using myListarrow_forwardJava - Normalizingarrow_forward17. The following function takes a parameter that is a list of integers; the list has at least one element. It is supposed to find the integer that is the largest and find the position of that integer in the list. The function has two incorrect lines of code that make the function compute the results incorrectly. Several lines are highlighted (bold, underlined) which COULD be incorrect. In the following questions, choose the correct line of code that should be in the function. 1 def findlarge(intlist): 2 largest = 0 3 pos = 0 for i in range(1,len(intlist)): if largest > intlist[i: 4 5 largest = intlist[i] pos = i return (largest, pos) 8 Select one of the following to correct a line of code in the function. a. Line 2 should be: largest = -1 b. Line 2 should be: largest = intlist[0] c. Line 4 should be: for i in range(0,len(intlist): %3D d. Line 4 should be: for i in range(1,len(intlist)-1): e. Line 8 should be: return largest 18. Select one of the following to correct a line of code in…arrow_forward
- Write a findSpelling Function -PHP Write a function findSpellings($word, $allWords) that takes a string and an array of dictionary words as parameters. The function should return an array of possible spellings for a misspelled $word. One way to approach this is to use the soundex() Words that have the same soundex are spelled similarly. Return an array of words from $allWords that match the soundex for $word. I have most of the code. How do I append the $sound to the $mathcing array?arrow_forwardhappy Eidarrow_forwardthis code should be in python: write a function that receives a list as its only parameter. Inside the function remove all the duplicate items from the list and returns a new list. For Example: If we call the function with [“dad”, “aunt”, “mom”, “sister”, “mom”] as its input, the returned value should be [“dad”, “aunt”, “mom”, “sister”]. The order of the items inside the returned list does not matter.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
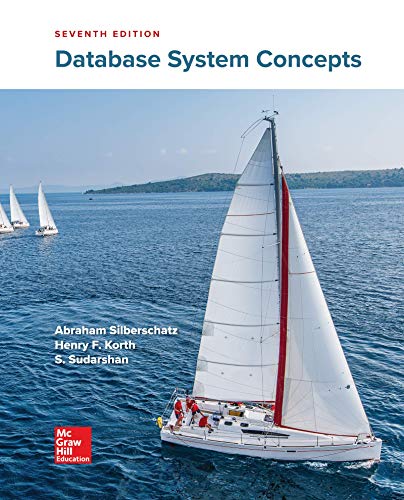
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
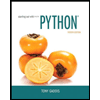
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
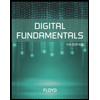
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
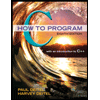
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
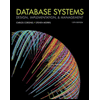
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
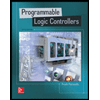
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education