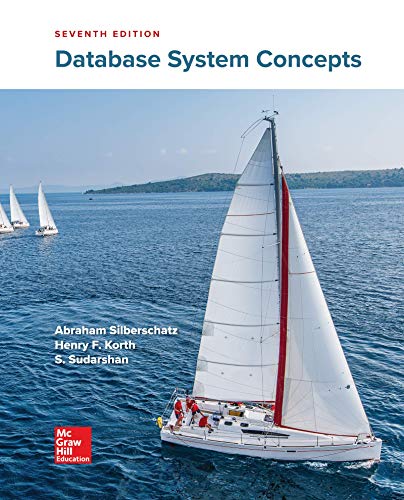
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Create a class called ResizingArrayQueueOfStrings that uses the queue abstraction using a fixed-size array. Then, as an extension, use array resizing in your implementation to get rid of the size restriction.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In this exercise you'll be completing an array-based and a link-based stack collection type discussed in this chapter. Both have similar implementations, but a link-based stack uses nodes and an array-based stack can resize the array if necessary. In the LinkedStack class of the linkedstack.py file, complete the following: Complete the implementation of the constructor method. __init__, sets the initial state of self, which includes the contents of sourceCollection, if it's present. Complete the implementation of the accessor methods. __iter__, supports iteration over a view of self and visits items from bottom to top of stack. peek(), returns the item at the top of the stack. Precondition: the stack is not empty. Raises: KeyError if the stack is empty. Complete the implementation of the mutator methods. clear(), makes self become empty. push(), adds item to the top of the stack. pop(), removes and returns the item at the top of the stack. Precondition: the stack is not…arrow_forwardImplement the complete stack functionality by using ArrayList which will containInteger values.arrow_forwardWite in java. Dont plagirize. Add comments. Keep code neat. Need this by today.arrow_forward
- You are going to implement a program that creates an unsorted list by using a linked list implemented by yourself. NOT allowed to use LinkedList class or any other classes that offers list functions. It is REQUIRED to use an ItemType class and a NodeType struct to solve this homework. The “data.txt” file has three lines of data 100, 110, 120, 130, 140, 150, 160 100, 130, 160 1@0, 2@3, 3@END You need to 1. create an empty unsorted list 2. add the numbers from the first line to list using putItem() function. Then print all the current keys to command line in one line using printAll(). 3. delete the numbers given by the second line in the list by using deleteItem() function. Then print all the current keys to command line in one line using printAll().. 4. putItem () the numbers in the third line of the data file to the corresponding location in the list. For example, 1@0 means adding number 1 at position 0 of the list. Then print all the current keys to command line in one…arrow_forwardHow do I code the interfaces, classes and JUnit 4 tests in java? this is all the information given below: A sentence in English is made of several words in a particular sequence. You must represent such a sentence as a list of words. Much like the list example we saw in class, this one is made of nodes (represented by a Sentence interface). There is one word per node, called WordNode. The sentence may also contain zero or more punctuation marks, which are represented by a PunctuationNode. The end of the sentence is denoted by a special empty node, called EmptyNode. Define the following operations for such a list of words: A method getNumberOfWords that computes and returns the number of words in a sentence. The punctuation does not count as a word. This method should answer/return an int. A method longestWord that determines and returns the longest word in a sentence. The word returned is just the word, and should not begin or end with punctuation. If the sentence contains no…arrow_forwardIn Java In this assignment, you should provide a complete CircularQueueDriver class that fullytests the functionality of your CircularQueue class that you have improved in Lab 8. YourCircularQueueDriver class should:• Instantiate a 3-element CircularQueue.• Use a loop to add strings to the queue until the add method returns false (whichindicates a full queue).• Call showQueue.• Use a loop to remove strings from the queue until the remove method returns null(which indicates an empty queue). As you remove each string from the queue, print theremoved string.Sample Run:Monsieur AMonsieur BMonsieur Cremoved: Monsieur Aremoved: Monsieur Bremoved: Monsieur C CircularQueue Class: public class CircularQueue { private String[] queue; // array that implements a circular queue private int length = 0; // number of filled elements private int capacity; // size of array private int front = 0; // index of first item in queue private int rear = 0; // one past the index of the last item…arrow_forward
- This is in c++ Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. Don't print the dummy head node. Example input : 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 output: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 main.cpp: #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the PrintNodeData() method// to print the entire linked list //…arrow_forwardProvide an explanation for the primary distinction that exists here between collection and an arraylist.arrow_forwardSuppose you are trapped on a desert island with nothing but a priority queue, and you need to implement a stack. Complete the following class that stores pairs (count, element) where the count is incremented with each insertion. Recall that make_pair(count, element) yields a pair object, and that p.second yields the second component of a pair p. The pair class defines an operator< that compares pairs by their first component, and uses the second component only to break ties.Code: #include <iostream>#include <queue>#include <string>#include <utility> using namespace std; class Stack{public: Stack(); string top(); void pop(); void push(string element);private: int count; priority_queue<pair<int, string>> pqueue;}; Stack::Stack(){ /* Your code goes here */} string Stack::top(){ /* Your code goes here */} void Stack::pop(){ /* Your code goes here */} void Stack::push(string element){ /* Your code goes here */} int main(){ Stack…arrow_forward
- In this project, you will implement a Set class that represents a general collection of values. For this assignment, a set is generally defined as a list of values that are sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. (in java)Requirements among all implementations there are some requirements that all implementations must maintain. • Your implementation should always reflect the definition of a set. • For simplicity, your set will be used to store Integer objects. • An ArrayList<Integer> object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections. the sort that automatically sorts a list may not be used. Instead, when a successful addition of an element to the Set is done, you can ensure that the elements inside the ArrayList<Integer>…arrow_forwardAssume class MyStack implements the following StackGen interface. For this question, make no assumptions about the implementation of MyStack except that the following interface methods are implemented and work as documented. Write a public instance method for MyStack, called interchange(T element) to replace the bottom "two" items in the stack with element. If there are fewer than two items on the stack, upon return the stack should contain exactly two items that are element.arrow_forwardIn python. Write a LinkedList class that has recursive implementations of the add and remove methods. It should also have recursive implementations of the contains, insert, and reverse methods. The reverse method should not change the data value each node holds - it must rearrange the order of the nodes in the linked list (by changing the next value each node holds). It should have a recursive method named to_plain_list that takes no parameters (unless they have default arguments) and returns a regular Python list that has the same values (from the data attribute of the Node objects), in the same order, as the current state of the linked list. The head data member of the LinkedList class must be private and have a get method defined (named get_head). It should return the first Node in the list (not the value inside it). As in the iterative LinkedList in the exploration, the data members of the Node class don't have to be private. The reason for that is because Node is a trivial class…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
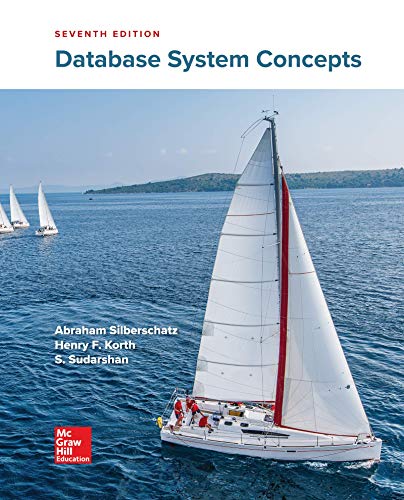
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
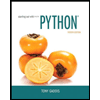
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
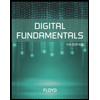
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
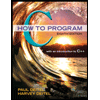
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
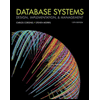
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
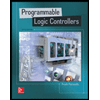
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education